Chapter 4 Linear Models
##
## Attaching package: 'dplyr'
## The following objects are masked from 'package:stats':
##
## filter, lag
## The following objects are masked from 'package:base':
##
## intersect, setdiff, setequal, union
4.1 Figures
Why is the normal so normal?
## simulate people stepping
thousand_steppers <- replicate(1e3, runif(16, -1, 1), simplify = FALSE)
steppers_df <- thousand_steppers %>%
map(cumsum) %>%
map_df(~ data_frame(position = c(0, .x),
step_num = seq_along(position)),
.id = "stepper")
steppers_df %>%
ggplot(aes(x = step_num, y = position, group = stepper)) +
geom_line(alpha = 0.2)
steppers_df %>%
filter(step_num == 16) %>%
ggplot(aes(x = position)) +
geom_density() +
stat_function(fun = dnorm, colour = "green", args = list(sd = 2.25))
Many small products can also cause a normal distribution
replicate(1e3, prod(1 + runif(12, 0, 0.1))) %>%
density() %>%
plot
## these parameter values ar just eyeballed
curve(dnorm(x, mean = 1.8, sd = 0.2), add = TRUE, col = "green")
4.1.1 Gaussian model of height
start by digging out the data.
data("Howell1", package = "rethinking")
knitr::kable(head(Howell1))
height | weight | age | male |
---|---|---|---|
151.765 | 47.82561 | 63 | 1 |
139.700 | 36.48581 | 63 | 0 |
136.525 | 31.86484 | 65 | 0 |
156.845 | 53.04191 | 41 | 1 |
145.415 | 41.27687 | 51 | 0 |
163.830 | 62.99259 | 35 | 1 |
## we're working only with adults
d2 <- Howell1 %>% filter(age > 18)
It is a good idea to plot one’s priors
data_frame(x = c(100, 250)) %>%
ggplot(aes(x = x)) +
stat_function(fun = dnorm, args = list(mean = 178, sd = 20))
data_frame(x = c(0, 60)) %>%
ggplot(aes(x = x)) +
stat_function(fun = dunif, args = list(min = 0, max = 50))
remember, every posterior is also potentially a prior for a subsequent analysis
Sounds like an important quote! but how do you use empirical priors?
Anyways, We are recommended to try to combine these priors in a simulation, to “see what these priors imply about the distribution of individual heights”. This despite what is said later in the book, about how priors role is “epistemilogical, not mechanistic” (or something like that).
rnorm(
n = 1e4,
mean = rnorm(1e4, 178, 20),
sd = runif(1e4, 0, 50)
) %>%
data_frame(priors = .) %>%
ggplot(aes(x = priors)) + geom_density()
4.1.2 grid approximation
post <- expand.grid(
mu = seq(from = 140, to = 160, length.out = 200),
sigma = seq(from = 4 , to = 9 , length.out = 200)
) %>%
mutate(LL = map2_dbl(mu, sigma,
~ d2$height %>%
dnorm(mean = .x, sd = .y, log = TRUE) %>%
sum))
## that gives us the liklihood. next we multiply by the prior -- or, since this
## is a log scale, we add it to the prior
height_posterior <- post %>%
mutate(prod = LL +
dnorm(mu, 178, 20, log = TRUE) +
dunif(sigma, 0, 50, log = TRUE),
prob = exp(prod - max(prod)))
## why on earth do we subtract the maximum??
with(height_posterior,
rethinking::contour_xyz(mu, sigma, prob))
We can also use sample_n()
to create a resampled posterior fairly easily?
height_posterior$prob %>% sum ## er should this be 1???
## [1] 308.6014
height_post_sampled <- height_posterior %>%
sample_n(1e4, replace = TRUE, weight = prob)
height_post_sampled %>%
ggplot(aes(x = mu, y = sigma)) +
stat_bin_hex(aes(fill = ..density..), bins = 30)
## Loading required package: methods
long_height_post <- height_post_sampled %>%
select(mu, sigma) %>%
tidyr::gather(param, value)
long_height_post %>%
ggplot(aes(x = value)) + geom_density() + facet_grid(~ param, scales = "free_x") + geom_rug()
Analyzing data from only 20 values of height:
## 20 subsamples
twenty_heights <- d2 %>% sample_n(20) %>% .[["height"]]
post <- tidyr::crossing(
mu.list = seq(from = 120, to = 170, length.out = 200),
sigma.list = seq(from = 4, to = 20, length.out = 200)
) %>%
mutate(LL = map2_dbl(mu.list, sigma.list,
~ dnorm(
x = twenty_heights,
mean = .x, sd = .y,
log = TRUE) %>%
sum))
post %>%
mutate(prod = LL +
dnorm(mu.list, 178, 20, TRUE) +
dunif(sigma.list, 0, 50, TRUE),
prob = exp(prod - max(prod))) %>%
## sample posterior
sample_n(1e4, weight = prob) %>%
ggplot(aes(x = mu.list, y = sigma.list)) +
stat_bin_hex(aes(fill = ..density..), bins = 37)
4.1.3 Fittin the model with MAP
data("Howell1", package = "rethinking")
## we're working only with adults
d2 <- Howell1 %>% filter(age > 18)
flist <- alist(
height ~ dnorm(mu, sigma),
mu ~ dnorm(178, 20),
sigma ~ dunif(0, 50)
)
m4.1 <- rethinking::map(flist, data = d2)
rethinking::precis(m4.1)
## An object of class "precis"
## Slot "output":
## Mean StdDev 5.5% 94.5%
## mu 154.654530 0.4172152 153.987740 155.321321
## sigma 7.762328 0.2950798 7.290733 8.233922
##
## Slot "digits":
## [1] 2
WE could also get the HDPI for the marginal posterior
m4.1 %>%
rethinking::extract.samples(n = 1e4) %>%
map(rethinking::HPDI)
## $mu
## |0.89 0.89|
## 153.9675 155.2916
##
## $sigma
## |0.89 0.89|
## 7.243285 8.204885
Note that the estimates are conditional on each others priors. For example:
m4.2 <- rethinking::map(
alist(
height ~ dnorm(mu, sigma),
mu ~ dnorm(178, 0.1),
sigma ~ dunif(0, 50)
),
data = d2)
rethinking::precis(m4.2)
## An object of class "precis"
## Slot "output":
## Mean StdDev 5.5% 94.5%
## mu 177.86598 0.1002314 177.70579 178.0262
## sigma 24.48462 0.9356075 22.98934 25.9799
##
## Slot "digits":
## [1] 2
To simulate confidence intervals that include the covariation, we simulate from a bivariate normal distribution:
rethinking::vcov(m4.1)
## mu sigma
## mu 0.1740685496 0.0002278744
## sigma 0.0002278744 0.0870721100
4.2 Adding a predictor
m4.3 <- rethinking::map(
alist(
height ~ dnorm(mu, sigma),
mu <- a + b * weight,
a ~ dnorm(156, 100),
b ~ dnorm(0, 10),
sigma ~ dunif(0, 50)
),
data = d2
)
rethinking::precis(m4.3, corr = TRUE)
## An object of class "precis"
## Slot "output":
## Mean StdDev 5.5% 94.5% a
## a 113.8239752 1.93872846 110.725513 116.9224378 1.0000000000
## b 0.9062098 0.04260545 0.838118 0.9743015 -0.9899123065
## sigma 5.1093962 0.19423065 4.798978 5.4198143 0.0006398681
## b sigma
## a -0.9899123065 0.0006398681
## b 1.0000000000 -0.0006337993
## sigma -0.0006337993 1.0000000000
##
## Slot "digits":
## [1] 2
These parameters are highly correlated.
## same model as before with centered variables
d2 %>%
mutate(weight = weight - mean(weight)) %>%
rethinking::map(data = .,
alist(
height ~ dnorm(mu, sigma),
mu <- a + b * weight,
a ~ dnorm(156, 100),
b ~ dnorm(0, 10),
sigma ~ dunif(0, 50)
)
) %>%
rethinking::precis(., corr = TRUE)
## An object of class "precis"
## Slot "output":
## Mean StdDev 5.5% 94.5% a
## a 154.6443791 0.27468127 154.2053853 155.0833728 1.000000e+00
## b 0.9065486 0.04261321 0.8384445 0.9746528 5.819321e-10
## sigma 5.1093863 0.19422970 4.7989697 5.4198028 2.841610e-06
## b sigma
## a 5.819321e-10 2.841610e-06
## b 1.000000e+00 -2.935764e-05
## sigma -2.935764e-05 1.000000e+00
##
## Slot "digits":
## [1] 2
Now to plot the predictions
d2 %>%
ggplot(aes(x = weight, y = height)) +
geom_point() +
geom_abline(intercept = rethinking::coef(m4.3)["a"],
slope = rethinking::coef(m4.3)["b"])
The correlated values of the posterior distribution contain the slope and intercept of many lines:
m4.3 <- d2 %>%
rethinking::map(data = .,
alist(
height ~ dnorm(mu, sigma),
mu <- a + b * weight,
a ~ dnorm(156, 100),
b ~ dnorm(0, 10),
sigma ~ dunif(0, 50)
)
)
d2 %>%
ggplot(aes(x = weight, y = height)) +
geom_point() +
geom_abline(aes(intercept = a,
slope = b),
data = rethinking::extract.samples(m4.3, n = 20),
alpha = 0.6)
what is the predicted height when weight is 50kg?
rethinking::extract.samples(m4.3, n = 300) %>%
mutate(mean_height_50 = a + b * 50) %>%
ggplot(aes(x = mean_height_50)) + geom_density() + geom_rug(alpha = 0.6)
This is the error about one point on the line. What about over the whole line?
## there is a function in rethinking that will create a matrix of predicted values
mu <- rethinking::link(m4.3)
## [ 100 / 1000 ]
[ 200 / 1000 ]
[ 300 / 1000 ]
[ 400 / 1000 ]
[ 500 / 1000 ]
[ 600 / 1000 ]
[ 700 / 1000 ]
[ 800 / 1000 ]
[ 900 / 1000 ]
[ 1000 / 1000 ]
dim(mu)
## [1] 1000 346
nrow(d2)
## [1] 346
Sure enough, there are as many columns as there were observations in the input data – and as many rows as there are samples from the posterior.
## create a data.frame for reorganizing
long_predictions <- mu %>%
as.data.frame %>%
mutate(sim_number = seq_along(V1)) %>%
tidyr::gather(observation_id, datapoint, -sim_number)
intervals <- long_predictions %>%
group_by(observation_id) %>%
tidyr::nest() %>%
mutate(rangedata = map(data, ~ rethinking::HPDI(.x$datapoint)),
lower = map_dbl(rangedata, 1),
upper = map_dbl(rangedata, 2)) %>%
select(observation_id, lower, upper)
d2 %>%
bind_cols(intervals) %>%
ggplot(aes(x = weight, y = height, ymax = upper, ymin = lower)) + geom_pointrange()
Not quite what we want! better would be to have predictions for a range of original values:
library(tidyr)
library(modelr)
prediction_range <- d2 %>%
data_grid(weight = seq_range(weight, 50))
model_grid_predictions <- prediction_range %>%
rethinking::link(m4.3, data = .) %>%
as.data.frame %>%
mutate(sim_number = seq_along(V1)) %>%
gather(observation_id, datapoint, -sim_number) %>%
nest(-observation_id) %>%
bind_cols(prediction_range)
## [ 100 / 1000 ]
[ 200 / 1000 ]
[ 300 / 1000 ]
[ 400 / 1000 ]
[ 500 / 1000 ]
[ 600 / 1000 ]
[ 700 / 1000 ]
[ 800 / 1000 ]
[ 900 / 1000 ]
[ 1000 / 1000 ]
We can visualize the distribution of the posterior at every value of x this way:
library(ggforce)
model_grid_predictions %>%
unnest(data) %>%
ggplot(aes(x = weight, y = datapoint, group = observation_id)) +
geom_sina(alpha = 0.01, pch = 21, fill = "black")
And we can add that uncertainty to the line itself as well:
mean_and_raw <- model_grid_predictions %>%
mutate(rangedata = map(data, ~ rethinking::HPDI(.x$datapoint)),
lower = map_dbl(rangedata, 1),
upper = map_dbl(rangedata, 2),
mean = map_dbl(data, ~ mean(.$datapoint))) %>%
select(observation_id, weight, lower, upper) %>%
ggplot(aes(x = weight, ymax = upper, ymin = lower)) +
geom_ribbon() +
geom_point(aes(x = weight, y = height, ymax = NULL, ymin = NULL), data = d2)
mean_and_raw
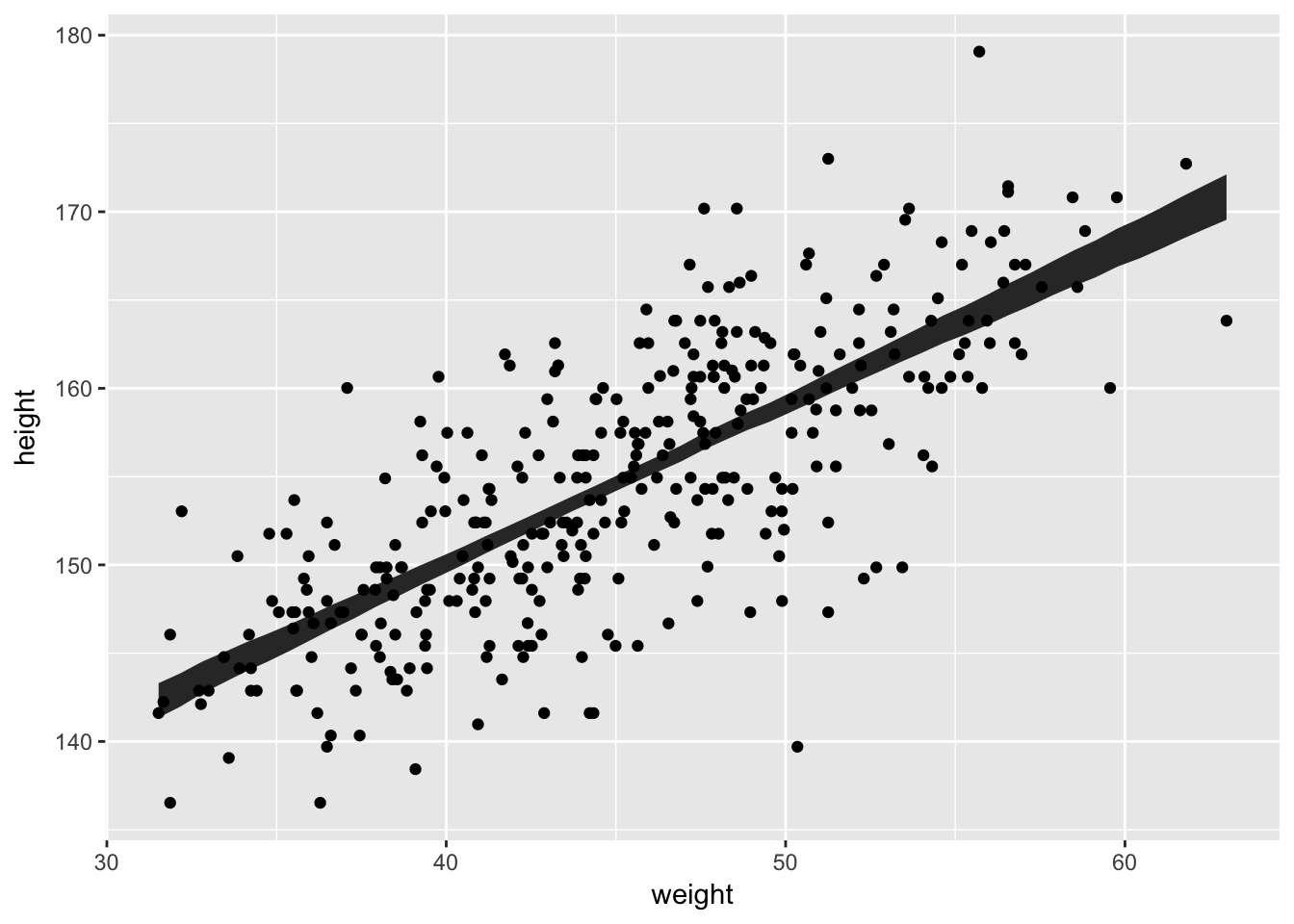
Figure 4.1: prediction of the mean as a function of weight, plotted with actual data as well.
simulated_data <- prediction_range %>%
rethinking::sim(m4.3, data = .) %>%
as.data.frame %>%
mutate(sim_number = seq_along(V1)) %>%
gather(observation_id, datapoint, -sim_number) %>%
nest(-observation_id) %>%
bind_cols(prediction_range)
## [ 100 / 1000 ]
[ 200 / 1000 ]
[ 300 / 1000 ]
[ 400 / 1000 ]
[ 500 / 1000 ]
[ 600 / 1000 ]
[ 700 / 1000 ]
[ 800 / 1000 ]
[ 900 / 1000 ]
[ 1000 / 1000 ]
simulated_data %>% head
## observation_id
## 1 V1
## 2 V2
## 3 V3
## 4 V4
## 5 V5
## 6 V6
## data
## 1 1.0000, 2.0000, 3.0000, 4.0000, 5.0000, 6.0000, 7.0000, 8.0000, 9.0000, 10.0000, 11.0000, 12.0000, 13.0000, 14.0000, 15.0000, 16.0000, 17.0000, 18.0000, 19.0000, 20.0000, 21.0000, 22.0000, 23.0000, 24.0000, 25.0000, 26.0000, 27.0000, 28.0000, 29.0000, 30.0000, 31.0000, 32.0000, 33.0000, 34.0000, 35.0000, 36.0000, 37.0000, 38.0000, 39.0000, 40.0000, 41.0000, 42.0000, 43.0000, 44.0000, 45.0000, 46.0000, 47.0000, 48.0000, 49.0000, 50.0000, 51.0000, 52.0000, 53.0000, 54.0000, 55.0000, 56.0000, 57.0000, 58.0000, 59.0000, 60.0000, 61.0000, 62.0000, 63.0000, 64.0000, 65.0000, 66.0000, 67.0000, 68.0000, 69.0000, 70.0000, 71.0000, 72.0000, 73.0000, 74.0000, 75.0000, 76.0000, 77.0000, 78.0000, 79.0000, 80.0000, 81.0000, 82.0000, 83.0000, 84.0000, 85.0000, 86.0000, 87.0000, 88.0000, 89.0000, 90.0000, 91.0000, 92.0000, 93.0000, 94.0000, 95.0000, 96.0000, 97.0000, 98.0000, 99.0000, 100.0000, 101.0000, 102.0000, 103.0000, 104.0000, 105.0000, 106.0000, 107.0000, 108.0000, 109.0000, 110.0000, 111.0000, 112.0000, 113.0000, 114.0000, 115.0000, 116.0000, 117.0000, 118.0000, 119.0000, 120.0000, 121.0000, 122.0000, 123.0000, 124.0000, 125.0000, 126.0000, 127.0000, 128.0000, 129.0000, 130.0000, 131.0000, 132.0000, 133.0000, 134.0000, 135.0000, 136.0000, 137.0000, 138.0000, 139.0000, 140.0000, 141.0000, 142.0000, 143.0000, 144.0000, 145.0000, 146.0000, 147.0000, 148.0000, 149.0000, 150.0000, 151.0000, 152.0000, 153.0000, 154.0000, 155.0000, 156.0000, 157.0000, 158.0000, 159.0000, 160.0000, 161.0000, 162.0000, 163.0000, 164.0000, 165.0000, 166.0000, 167.0000, 168.0000, 169.0000, 170.0000, 171.0000, 172.0000, 173.0000, 174.0000, 175.0000, 176.0000, 177.0000, 178.0000, 179.0000, 180.0000, 181.0000, 182.0000, 183.0000, 184.0000, 185.0000, 186.0000, 187.0000, 188.0000, 189.0000, 190.0000, 191.0000, 192.0000, 193.0000, 194.0000, 195.0000, 196.0000, 197.0000, 198.0000, 199.0000, 200.0000, 201.0000, 202.0000, 203.0000, 204.0000, 205.0000, 206.0000, 207.0000, 208.0000, 209.0000, 210.0000, 211.0000, 212.0000, 213.0000, 214.0000, 215.0000, 216.0000, 217.0000, 218.0000, 219.0000, 220.0000, 221.0000, 222.0000, 223.0000, 224.0000, 225.0000, 226.0000, 227.0000, 228.0000, 229.0000, 230.0000, 231.0000, 232.0000, 233.0000, 234.0000, 235.0000, 236.0000, 237.0000, 238.0000, 239.0000, 240.0000, 241.0000, 242.0000, 243.0000, 244.0000, 245.0000, 246.0000, 247.0000, 248.0000, 249.0000, 250.0000, 251.0000, 252.0000, 253.0000, 254.0000, 255.0000, 256.0000, 257.0000, 258.0000, 259.0000, 260.0000, 261.0000, 262.0000, 263.0000, 264.0000, 265.0000, 266.0000, 267.0000, 268.0000, 269.0000, 270.0000, 271.0000, 272.0000, 273.0000, 274.0000, 275.0000, 276.0000, 277.0000, 278.0000, 279.0000, 280.0000, 281.0000, 282.0000, 283.0000, 284.0000, 285.0000, 286.0000, 287.0000, 288.0000, 289.0000, 290.0000, 291.0000, 292.0000, 293.0000, 294.0000, 295.0000, 296.0000, 297.0000, 298.0000, 299.0000, 300.0000, 301.0000, 302.0000, 303.0000, 304.0000, 305.0000, 306.0000, 307.0000, 308.0000, 309.0000, 310.0000, 311.0000, 312.0000, 313.0000, 314.0000, 315.0000, 316.0000, 317.0000, 318.0000, 319.0000, 320.0000, 321.0000, 322.0000, 323.0000, 324.0000, 325.0000, 326.0000, 327.0000, 328.0000, 329.0000, 330.0000, 331.0000, 332.0000, 333.0000, 334.0000, 335.0000, 336.0000, 337.0000, 338.0000, 339.0000, 340.0000, 341.0000, 342.0000, 343.0000, 344.0000, 345.0000, 346.0000, 347.0000, 348.0000, 349.0000, 350.0000, 351.0000, 352.0000, 353.0000, 354.0000, 355.0000, 356.0000, 357.0000, 358.0000, 359.0000, 360.0000, 361.0000, 362.0000, 363.0000, 364.0000, 365.0000, 366.0000, 367.0000, 368.0000, 369.0000, 370.0000, 371.0000, 372.0000, 373.0000, 374.0000, 375.0000, 376.0000, 377.0000, 378.0000, 379.0000, 380.0000, 381.0000, 382.0000, 383.0000, 384.0000, 385.0000, 386.0000, 387.0000, 388.0000, 389.0000, 390.0000, 391.0000, 392.0000, 393.0000, 394.0000, 395.0000, 396.0000, 397.0000, 398.0000, 399.0000, 400.0000, 401.0000, 402.0000, 403.0000, 404.0000, 405.0000, 406.0000, 407.0000, 408.0000, 409.0000, 410.0000, 411.0000, 412.0000, 413.0000, 414.0000, 415.0000, 416.0000, 417.0000, 418.0000, 419.0000, 420.0000, 421.0000, 422.0000, 423.0000, 424.0000, 425.0000, 426.0000, 427.0000, 428.0000, 429.0000, 430.0000, 431.0000, 432.0000, 433.0000, 434.0000, 435.0000, 436.0000, 437.0000, 438.0000, 439.0000, 440.0000, 441.0000, 442.0000, 443.0000, 444.0000, 445.0000, 446.0000, 447.0000, 448.0000, 449.0000, 450.0000, 451.0000, 452.0000, 453.0000, 454.0000, 455.0000, 456.0000, 457.0000, 458.0000, 459.0000, 460.0000, 461.0000, 462.0000, 463.0000, 464.0000, 465.0000, 466.0000, 467.0000, 468.0000, 469.0000, 470.0000, 471.0000, 472.0000, 473.0000, 474.0000, 475.0000, 476.0000, 477.0000, 478.0000, 479.0000, 480.0000, 481.0000, 482.0000, 483.0000, 484.0000, 485.0000, 486.0000, 487.0000, 488.0000, 489.0000, 490.0000, 491.0000, 492.0000, 493.0000, 494.0000, 495.0000, 496.0000, 497.0000, 498.0000, 499.0000, 500.0000, 501.0000, 502.0000, 503.0000, 504.0000, 505.0000, 506.0000, 507.0000, 508.0000, 509.0000, 510.0000, 511.0000, 512.0000, 513.0000, 514.0000, 515.0000, 516.0000, 517.0000, 518.0000, 519.0000, 520.0000, 521.0000, 522.0000, 523.0000, 524.0000, 525.0000, 526.0000, 527.0000, 528.0000, 529.0000, 530.0000, 531.0000, 532.0000, 533.0000, 534.0000, 535.0000, 536.0000, 537.0000, 538.0000, 539.0000, 540.0000, 541.0000, 542.0000, 543.0000, 544.0000, 545.0000, 546.0000, 547.0000, 548.0000, 549.0000, 550.0000, 551.0000, 552.0000, 553.0000, 554.0000, 555.0000, 556.0000, 557.0000, 558.0000, 559.0000, 560.0000, 561.0000, 562.0000, 563.0000, 564.0000, 565.0000, 566.0000, 567.0000, 568.0000, 569.0000, 570.0000, 571.0000, 572.0000, 573.0000, 574.0000, 575.0000, 576.0000, 577.0000, 578.0000, 579.0000, 580.0000, 581.0000, 582.0000, 583.0000, 584.0000, 585.0000, 586.0000, 587.0000, 588.0000, 589.0000, 590.0000, 591.0000, 592.0000, 593.0000, 594.0000, 595.0000, 596.0000, 597.0000, 598.0000, 599.0000, 600.0000, 601.0000, 602.0000, 603.0000, 604.0000, 605.0000, 606.0000, 607.0000, 608.0000, 609.0000, 610.0000, 611.0000, 612.0000, 613.0000, 614.0000, 615.0000, 616.0000, 617.0000, 618.0000, 619.0000, 620.0000, 621.0000, 622.0000, 623.0000, 624.0000, 625.0000, 626.0000, 627.0000, 628.0000, 629.0000, 630.0000, 631.0000, 632.0000, 633.0000, 634.0000, 635.0000, 636.0000, 637.0000, 638.0000, 639.0000, 640.0000, 641.0000, 642.0000, 643.0000, 644.0000, 645.0000, 646.0000, 647.0000, 648.0000, 649.0000, 650.0000, 651.0000, 652.0000, 653.0000, 654.0000, 655.0000, 656.0000, 657.0000, 658.0000, 659.0000, 660.0000, 661.0000, 662.0000, 663.0000, 664.0000, 665.0000, 666.0000, 667.0000, 668.0000, 669.0000, 670.0000, 671.0000, 672.0000, 673.0000, 674.0000, 675.0000, 676.0000, 677.0000, 678.0000, 679.0000, 680.0000, 681.0000, 682.0000, 683.0000, 684.0000, 685.0000, 686.0000, 687.0000, 688.0000, 689.0000, 690.0000, 691.0000, 692.0000, 693.0000, 694.0000, 695.0000, 696.0000, 697.0000, 698.0000, 699.0000, 700.0000, 701.0000, 702.0000, 703.0000, 704.0000, 705.0000, 706.0000, 707.0000, 708.0000, 709.0000, 710.0000, 711.0000, 712.0000, 713.0000, 714.0000, 715.0000, 716.0000, 717.0000, 718.0000, 719.0000, 720.0000, 721.0000, 722.0000, 723.0000, 724.0000, 725.0000, 726.0000, 727.0000, 728.0000, 729.0000, 730.0000, 731.0000, 732.0000, 733.0000, 734.0000, 735.0000, 736.0000, 737.0000, 738.0000, 739.0000, 740.0000, 741.0000, 742.0000, 743.0000, 744.0000, 745.0000, 746.0000, 747.0000, 748.0000, 749.0000, 750.0000, 751.0000, 752.0000, 753.0000, 754.0000, 755.0000, 756.0000, 757.0000, 758.0000, 759.0000, 760.0000, 761.0000, 762.0000, 763.0000, 764.0000, 765.0000, 766.0000, 767.0000, 768.0000, 769.0000, 770.0000, 771.0000, 772.0000, 773.0000, 774.0000, 775.0000, 776.0000, 777.0000, 778.0000, 779.0000, 780.0000, 781.0000, 782.0000, 783.0000, 784.0000, 785.0000, 786.0000, 787.0000, 788.0000, 789.0000, 790.0000, 791.0000, 792.0000, 793.0000, 794.0000, 795.0000, 796.0000, 797.0000, 798.0000, 799.0000, 800.0000, 801.0000, 802.0000, 803.0000, 804.0000, 805.0000, 806.0000, 807.0000, 808.0000, 809.0000, 810.0000, 811.0000, 812.0000, 813.0000, 814.0000, 815.0000, 816.0000, 817.0000, 818.0000, 819.0000, 820.0000, 821.0000, 822.0000, 823.0000, 824.0000, 825.0000, 826.0000, 827.0000, 828.0000, 829.0000, 830.0000, 831.0000, 832.0000, 833.0000, 834.0000, 835.0000, 836.0000, 837.0000, 838.0000, 839.0000, 840.0000, 841.0000, 842.0000, 843.0000, 844.0000, 845.0000, 846.0000, 847.0000, 848.0000, 849.0000, 850.0000, 851.0000, 852.0000, 853.0000, 854.0000, 855.0000, 856.0000, 857.0000, 858.0000, 859.0000, 860.0000, 861.0000, 862.0000, 863.0000, 864.0000, 865.0000, 866.0000, 867.0000, 868.0000, 869.0000, 870.0000, 871.0000, 872.0000, 873.0000, 874.0000, 875.0000, 876.0000, 877.0000, 878.0000, 879.0000, 880.0000, 881.0000, 882.0000, 883.0000, 884.0000, 885.0000, 886.0000, 887.0000, 888.0000, 889.0000, 890.0000, 891.0000, 892.0000, 893.0000, 894.0000, 895.0000, 896.0000, 897.0000, 898.0000, 899.0000, 900.0000, 901.0000, 902.0000, 903.0000, 904.0000, 905.0000, 906.0000, 907.0000, 908.0000, 909.0000, 910.0000, 911.0000, 912.0000, 913.0000, 914.0000, 915.0000, 916.0000, 917.0000, 918.0000, 919.0000, 920.0000, 921.0000, 922.0000, 923.0000, 924.0000, 925.0000, 926.0000, 927.0000, 928.0000, 929.0000, 930.0000, 931.0000, 932.0000, 933.0000, 934.0000, 935.0000, 936.0000, 937.0000, 938.0000, 939.0000, 940.0000, 941.0000, 942.0000, 943.0000, 944.0000, 945.0000, 946.0000, 947.0000, 948.0000, 949.0000, 950.0000, 951.0000, 952.0000, 953.0000, 954.0000, 955.0000, 956.0000, 957.0000, 958.0000, 959.0000, 960.0000, 961.0000, 962.0000, 963.0000, 964.0000, 965.0000, 966.0000, 967.0000, 968.0000, 969.0000, 970.0000, 971.0000, 972.0000, 973.0000, 974.0000, 975.0000, 976.0000, 977.0000, 978.0000, 979.0000, 980.0000, 981.0000, 982.0000, 983.0000, 984.0000, 985.0000, 986.0000, 987.0000, 988.0000, 989.0000, 990.0000, 991.0000, 992.0000, 993.0000, 994.0000, 995.0000, 996.0000, 997.0000, 998.0000, 999.0000, 1000.0000, 136.7423, 146.0499, 147.9066, 143.6836, 141.6695, 145.1672, 136.8597, 136.3430, 133.0352, 139.2679, 142.2228, 140.2721, 134.9249, 140.2267, 140.8727, 143.8511, 137.4756, 133.3616, 141.0886, 140.6695, 149.2804, 150.9504, 150.6845, 137.4395, 142.6088, 146.2148, 147.6233, 138.8718, 140.9725, 141.0736, 147.3785, 143.6913, 139.0934, 143.5038, 150.5986, 136.4598, 142.8202, 143.3837, 155.0467, 134.3473, 141.9632, 137.0498, 139.5292, 134.5768, 141.9316, 143.0223, 142.5529, 135.0951, 138.2855, 142.7344, 136.9446, 141.1075, 143.8403, 144.2026, 138.3041, 147.4447, 141.2467, 140.4542, 140.1608, 144.8200, 136.1765, 153.3873, 137.8964, 148.5996, 145.6030, 140.1052, 140.4520, 150.3529, 141.0316, 142.0798, 139.2515, 139.0861, 138.5142, 150.6983, 134.0735, 138.4516, 147.1315, 142.2587, 147.1014, 143.6891, 143.3058, 146.0915, 145.0071, 157.2096, 143.3612, 139.3196, 129.4903, 142.8300, 138.1237, 132.9289, 130.7930, 135.8773, 142.0652, 144.1742, 136.8592, 137.8235, 144.4875, 137.9536, 140.2166, 152.8044, 142.8813, 145.3434, 143.4732, 145.8770, 152.0573, 153.1197, 142.8019, 141.1367, 144.4989, 147.0839, 131.4529, 150.0484, 148.6138, 140.0604, 148.3512, 138.8354, 150.6716, 139.6220, 139.2260, 141.4884, 137.4968, 136.8412, 147.0706, 136.8156, 149.9598, 142.5692, 136.3746, 140.6158, 139.9629, 139.5091, 142.4447, 135.5523, 149.1499, 139.8750, 138.5218, 143.1606, 137.9933, 144.8623, 140.5111, 138.2615, 137.7098, 141.1369, 149.2035, 143.9079, 140.5704, 141.0624, 136.3309, 141.2834, 141.7967, 137.0064, 133.1281, 133.7411, 139.0191, 140.8867, 139.9117, 143.5946, 135.4969, 145.5631, 141.7950, 143.9124, 143.9687, 146.3315, 128.5111, 153.2204, 147.7900, 140.9823, 147.2847, 142.5915, 140.3770, 143.1548, 144.6845, 144.3607, 137.4881, 149.5486, 148.1280, 140.0931, 148.7042, 145.2455, 142.0705, 145.4555, 137.5294, 146.6764, 143.8833, 146.4982, 156.2227, 148.0786, 140.5583, 138.3353, 153.3897, 143.3440, 139.6901, 149.2923, 134.5408, 139.9260, 145.6981, 136.4673, 144.1493, 143.2651, 143.1637, 132.3409, 132.9637, 144.3300, 147.9178, 138.6953, 140.1151, 145.2917, 139.5918, 137.4148, 150.6761, 145.9100, 137.0579, 139.5287, 144.9259, 144.5200, 142.8278, 151.5278, 139.4616, 155.0106, 138.0562, 142.9337, 140.7681, 143.0235, 148.0068, 145.1772, 142.7494, 136.1394, 143.7740, 144.5922, 140.9022, 148.1004, 146.5965, 135.6293, 146.1053, 144.9199, 138.3469, 139.6029, 140.5934, 148.9053, 142.7754, 138.5460, 135.1598, 148.5234, 137.6540, 146.4777, 144.6280, 139.3679, 136.9683, 150.2215, 143.9420, 140.2766, 150.4599, 144.3210, 136.0607, 145.6710, 149.4358, 142.7928, 139.2300, 146.8687, 143.5789, 146.1639, 141.2043, 135.6604, 141.5519, 138.4549, 134.9922, 142.6824, 146.1324, 148.0356, 134.9412, 135.2493, 136.3177, 154.0973, 149.1945, 141.5365, 145.7832, 146.6786, 144.8478, 149.0781, 138.6986, 144.7294, 149.9728, 136.9562, 146.0542, 146.4442, 145.4752, 144.5069, 142.0585, 148.3400, 146.3026, 146.7271, 150.9672, 141.8989, 144.2300, 135.4193, 145.2452, 143.6155, 130.1668, 151.0327, 144.4608, 142.0940, 134.8329, 139.1767, 149.0808, 141.5857, 137.4002, 144.0506, 143.5780, 135.0949, 140.9920, 138.1173, 144.4127, 146.1973, 146.8504, 140.5396, 149.2685, 140.5955, 138.6344, 137.1370, 138.5254, 141.1139, 141.2760, 149.8193, 148.9772, 145.1251, 144.0698, 146.0504, 139.7523, 145.0485, 144.7432, 140.1723, 142.5923, 142.4195, 141.9531, 137.3257, 141.8689, 145.7579, 135.8009, 140.6243, 145.3265, 149.1307, 140.9169, 138.6789, 139.6404, 139.9452, 140.5514, 138.3722, 144.2752, 139.0224, 143.0549, 135.2680, 146.7614, 140.5494, 148.8742, 149.1945, 146.7193, 148.6813, 147.4710, 141.5596, 149.7136, 143.9407, 140.9568, 146.0588, 140.4872, 137.6469, 144.3052, 149.3103, 153.5163, 136.9503, 148.0704, 144.9756, 137.4725, 142.4133, 140.7611, 135.4667, 144.8634, 142.6674, 143.7230, 138.8523, 138.4464, 139.7390, 140.1534, 151.1275, 140.3994, 140.2415, 140.0764, 153.1091, 152.5640, 144.8101, 140.0033, 139.9675, 143.4309, 154.1719, 139.0268, 129.4700, 138.7147, 138.6059, 143.6356, 137.3931, 144.2658, 151.0935, 144.8064, 136.2964, 140.4648, 139.0235, 142.9889, 139.0039, 150.3300, 140.9459, 144.3600, 144.2605, 143.6869, 138.6644, 141.4303, 131.3345, 135.7342, 139.9174, 134.4058, 140.6326, 149.6698, 140.2531, 144.3896, 135.6448, 143.5342, 134.9762, 139.9898, 136.4394, 145.6527, 141.3748, 146.0160, 149.4347, 140.6580, 145.8476, 139.2834, 139.3700, 144.1352, 141.8655, 136.7501, 144.8299, 139.7905, 131.8401, 141.7784, 145.6080, 142.8317, 126.0225, 141.5679, 146.0323, 138.3847, 150.5961, 146.1414, 147.7191, 142.7974, 138.6756, 141.3218, 141.7826, 141.3809, 140.9839, 145.1533, 142.9816, 143.6928, 139.7226, 145.1275, 150.6791, 140.0525, 132.0790, 141.2930, 137.1877, 141.5357, 142.2074, 148.8339, 134.8214, 143.6445, 142.6148, 141.8303, 141.4187, 132.8352, 143.0537, 141.6330, 148.9612, 136.4430, 142.4604, 134.3163, 141.7129, 142.3753, 143.6608, 149.3136, 149.7217, 140.8918, 140.0542, 151.0591, 143.3734, 147.4881, 150.9899, 139.4992, 135.8358, 142.2783, 138.9877, 140.4549, 149.8472, 138.4014, 148.9644, 147.7155, 140.0265, 142.1317, 151.6865, 145.0183, 146.6522, 141.4571, 142.6380, 135.8989, 133.5585, 145.0173, 141.3638, 138.9134, 142.6695, 134.1763, 149.7525, 138.5560, 136.5845, 151.2778, 135.3073, 146.2411, 138.0220, 138.5252, 135.5347, 139.5493, 150.2149, 145.4768, 144.4344, 144.6923, 141.8199, 146.0227, 142.8977, 141.0673, 137.7355, 142.2513, 145.8351, 145.6593, 142.0978, 143.3987, 142.8050, 136.7315, 142.7409, 140.1312, 148.8591, 140.2655, 145.7642, 133.1493, 146.5438, 142.5663, 135.7344, 141.1861, 139.2169, 142.9543, 141.7693, 138.6912, 148.5624, 150.3688, 148.8550, 144.9797, 134.7972, 136.9185, 142.9231, 142.3707, 146.9700, 134.6475, 143.9754, 149.4825, 149.3568, 134.4767, 138.8730, 144.2552, 145.9980, 148.9410, 136.7589, 144.3931, 134.3952, 147.2913, 141.2704, 137.6357, 142.0263, 139.9562, 150.2709, 150.0048, 145.5984, 140.9592, 133.6283, 144.7210, 142.0893, 140.7111, 140.6125, 143.3290, 138.8801, 135.2776, 139.9728, 142.2404, 136.4246, 132.3553, 145.8338, 148.3530, 141.4121, 136.5088, 146.4713, 141.5318, 146.5060, 139.5076, 147.6791, 153.2108, 147.7565, 139.4356, 138.1649, 144.0851, 137.7121, 145.7841, 144.2875, 138.4293, 149.1749, 146.9165, 140.4279, 140.2664, 134.4822, 148.6087, 148.9578, 142.3870, 144.3712, 141.1747, 144.3068, 143.4536, 139.3906, 129.4217, 143.2438, 142.6219, 145.7317, 139.6192, 149.3298, 145.4185, 140.6820, 143.6556, 142.1682, 144.6676, 144.5546, 148.2369, 141.3805, 133.8305, 140.0300, 140.4512, 135.3134, 145.2248, 147.9772, 142.9808, 143.4373, 149.1494, 136.4945, 144.4383, 150.6185, 141.6315, 144.9543, 152.8884, 137.4205, 131.6451, 138.4882, 134.2632, 145.9609, 133.0822, 139.7123, 146.3485, 149.8104, 143.1960, 142.0418, 139.5530, 145.6952, 145.8419, 147.0635, 140.7871, 148.1646, 135.4808, 141.2365, 139.5032, 140.6469, 138.5887, 139.3964, 143.3718, 137.8785, 148.6077, 146.4699, 141.1282, 148.4508, 140.8098, 152.9501, 140.8015, 140.5902, 144.9363, 139.4180, 145.6906, 143.5411, 142.3807, 143.5466, 147.8983, 148.5300, 147.4106, 142.7597, 150.1484, 136.1829, 146.0194, 128.0590, 145.3600, 146.8930, 130.1637, 140.7364, 140.8256, 129.7422, 148.1061, 137.7985, 138.8131, 139.3257, 138.0209, 141.0289, 139.9706, 136.4326, 147.0549, 133.9258, 147.7061, 133.2615, 145.3683, 144.1286, 142.9830, 146.7897, 141.8782, 133.8634, 138.0391, 132.3777, 133.7550, 139.0853, 148.2547, 148.6332, 143.5634, 144.4856, 138.4961, 138.5221, 140.3038, 142.6162, 135.8541, 137.3776, 134.5122, 146.9269, 147.0666, 138.7528, 137.8902, 150.2445, 135.6539, 141.2822, 141.4550, 146.4490, 139.9798, 143.5683, 143.0473, 148.0964, 139.5406, 149.8334, 145.7939, 140.1687, 135.2751, 148.1226, 139.8297, 140.0180, 142.0013, 140.7249, 135.1249, 145.7605, 137.9976, 145.2723, 149.3521, 144.3267, 144.7945, 135.8706, 139.3442, 148.2655, 149.0320, 142.5633, 132.3115, 143.6465, 135.8747, 140.8360, 142.4348, 140.4242, 149.9415, 137.9292, 132.9592, 142.7769, 139.9938, 133.4242, 143.0191, 151.5500, 144.0305, 141.2804, 152.1015, 144.0836, 137.0500, 143.2600, 141.2107, 133.2302, 145.7279, 142.9657, 128.0198, 145.0904, 136.7122, 143.3708, 143.8904, 137.7083, 142.8691, 147.6884, 151.5788, 136.3239, 143.8455, 144.5522, 140.0612, 143.6158, 139.7208, 144.6698, 134.9125, 150.8547, 142.6826, 140.6284, 143.0465, 145.4879, 148.6188, 146.2011, 141.1501, 131.2213, 139.3269, 142.9869, 141.5148, 147.0078, 145.4630, 139.0782, 143.9523, 136.9943, 147.3801, 140.5494, 139.6503, 140.3646, 144.1037, 138.0068, 140.7581, 146.3323, 150.4345, 144.2998, 148.0157, 135.2805, 140.0497, 136.2779, 145.8782, 141.2294, 140.6963, 135.1861, 142.0617, 149.3625, 144.0990, 149.9043, 144.0022, 145.6052, 139.7357, 144.8674, 144.2673, 150.6334, 145.0104, 135.1439, 144.9584, 142.4970, 143.9411, 150.5217, 137.6660, 138.4881, 140.8680, 142.2100, 143.3476, 144.1278, 145.0485, 131.4824, 137.5751, 139.9639, 137.9433, 136.3666, 155.7033, 151.8232, 140.1515, 136.2120, 146.6811, 138.6654, 145.7371, 148.5108, 139.9240, 141.7408, 142.9718, 130.5078, 138.2627, 148.4875, 142.6343, 139.5210, 140.3444, 142.8996, 144.8551, 149.4575, 142.5929, 128.4510, 134.5316, 136.3330, 153.9137, 143.7299, 145.6828, 136.0724, 144.7563, 137.6893, 145.7210, 148.1247, 143.2285, 155.3775, 135.7514, 144.0049, 150.3555, 144.8089, 144.7756, 141.1961, 139.5387, 145.9998, 145.0087, 147.7668, 139.4443, 140.7360, 135.4579, 138.8042, 142.8838, 134.5492, 141.3781, 147.0528, 142.9107, 145.9274, 134.6495, 146.8150, 146.1105, 139.2219, 137.4541, 136.8709, 139.2901, 135.8318, 140.0279, 142.6372, 141.8837, 149.4483, 135.9978, 139.7748, 134.2561, 144.5860, 146.0846, 144.3393, 138.1683, 136.4924, 137.1817, 138.8559, 139.0722, 149.4929, 134.2167, 143.1503, 147.3429, 135.8564, 141.7102, 135.0478, 134.4131, 145.9607, 153.6945, 149.8239, 146.6111, 143.3003, 144.2016, 132.0137, 143.8882, 141.1066, 139.3308, 138.8326, 148.9224, 147.2875, 148.1761, 141.9040, 153.5484, 138.7030, 144.2865, 138.2092, 141.0154, 145.0883, 139.8882, 146.4105, 145.9275, 147.6525, 147.9774, 147.9582, 131.9517, 143.9518, 137.2306, 143.8260, 152.3912, 133.8644
## 2 1.0000, 2.0000, 3.0000, 4.0000, 5.0000, 6.0000, 7.0000, 8.0000, 9.0000, 10.0000, 11.0000, 12.0000, 13.0000, 14.0000, 15.0000, 16.0000, 17.0000, 18.0000, 19.0000, 20.0000, 21.0000, 22.0000, 23.0000, 24.0000, 25.0000, 26.0000, 27.0000, 28.0000, 29.0000, 30.0000, 31.0000, 32.0000, 33.0000, 34.0000, 35.0000, 36.0000, 37.0000, 38.0000, 39.0000, 40.0000, 41.0000, 42.0000, 43.0000, 44.0000, 45.0000, 46.0000, 47.0000, 48.0000, 49.0000, 50.0000, 51.0000, 52.0000, 53.0000, 54.0000, 55.0000, 56.0000, 57.0000, 58.0000, 59.0000, 60.0000, 61.0000, 62.0000, 63.0000, 64.0000, 65.0000, 66.0000, 67.0000, 68.0000, 69.0000, 70.0000, 71.0000, 72.0000, 73.0000, 74.0000, 75.0000, 76.0000, 77.0000, 78.0000, 79.0000, 80.0000, 81.0000, 82.0000, 83.0000, 84.0000, 85.0000, 86.0000, 87.0000, 88.0000, 89.0000, 90.0000, 91.0000, 92.0000, 93.0000, 94.0000, 95.0000, 96.0000, 97.0000, 98.0000, 99.0000, 100.0000, 101.0000, 102.0000, 103.0000, 104.0000, 105.0000, 106.0000, 107.0000, 108.0000, 109.0000, 110.0000, 111.0000, 112.0000, 113.0000, 114.0000, 115.0000, 116.0000, 117.0000, 118.0000, 119.0000, 120.0000, 121.0000, 122.0000, 123.0000, 124.0000, 125.0000, 126.0000, 127.0000, 128.0000, 129.0000, 130.0000, 131.0000, 132.0000, 133.0000, 134.0000, 135.0000, 136.0000, 137.0000, 138.0000, 139.0000, 140.0000, 141.0000, 142.0000, 143.0000, 144.0000, 145.0000, 146.0000, 147.0000, 148.0000, 149.0000, 150.0000, 151.0000, 152.0000, 153.0000, 154.0000, 155.0000, 156.0000, 157.0000, 158.0000, 159.0000, 160.0000, 161.0000, 162.0000, 163.0000, 164.0000, 165.0000, 166.0000, 167.0000, 168.0000, 169.0000, 170.0000, 171.0000, 172.0000, 173.0000, 174.0000, 175.0000, 176.0000, 177.0000, 178.0000, 179.0000, 180.0000, 181.0000, 182.0000, 183.0000, 184.0000, 185.0000, 186.0000, 187.0000, 188.0000, 189.0000, 190.0000, 191.0000, 192.0000, 193.0000, 194.0000, 195.0000, 196.0000, 197.0000, 198.0000, 199.0000, 200.0000, 201.0000, 202.0000, 203.0000, 204.0000, 205.0000, 206.0000, 207.0000, 208.0000, 209.0000, 210.0000, 211.0000, 212.0000, 213.0000, 214.0000, 215.0000, 216.0000, 217.0000, 218.0000, 219.0000, 220.0000, 221.0000, 222.0000, 223.0000, 224.0000, 225.0000, 226.0000, 227.0000, 228.0000, 229.0000, 230.0000, 231.0000, 232.0000, 233.0000, 234.0000, 235.0000, 236.0000, 237.0000, 238.0000, 239.0000, 240.0000, 241.0000, 242.0000, 243.0000, 244.0000, 245.0000, 246.0000, 247.0000, 248.0000, 249.0000, 250.0000, 251.0000, 252.0000, 253.0000, 254.0000, 255.0000, 256.0000, 257.0000, 258.0000, 259.0000, 260.0000, 261.0000, 262.0000, 263.0000, 264.0000, 265.0000, 266.0000, 267.0000, 268.0000, 269.0000, 270.0000, 271.0000, 272.0000, 273.0000, 274.0000, 275.0000, 276.0000, 277.0000, 278.0000, 279.0000, 280.0000, 281.0000, 282.0000, 283.0000, 284.0000, 285.0000, 286.0000, 287.0000, 288.0000, 289.0000, 290.0000, 291.0000, 292.0000, 293.0000, 294.0000, 295.0000, 296.0000, 297.0000, 298.0000, 299.0000, 300.0000, 301.0000, 302.0000, 303.0000, 304.0000, 305.0000, 306.0000, 307.0000, 308.0000, 309.0000, 310.0000, 311.0000, 312.0000, 313.0000, 314.0000, 315.0000, 316.0000, 317.0000, 318.0000, 319.0000, 320.0000, 321.0000, 322.0000, 323.0000, 324.0000, 325.0000, 326.0000, 327.0000, 328.0000, 329.0000, 330.0000, 331.0000, 332.0000, 333.0000, 334.0000, 335.0000, 336.0000, 337.0000, 338.0000, 339.0000, 340.0000, 341.0000, 342.0000, 343.0000, 344.0000, 345.0000, 346.0000, 347.0000, 348.0000, 349.0000, 350.0000, 351.0000, 352.0000, 353.0000, 354.0000, 355.0000, 356.0000, 357.0000, 358.0000, 359.0000, 360.0000, 361.0000, 362.0000, 363.0000, 364.0000, 365.0000, 366.0000, 367.0000, 368.0000, 369.0000, 370.0000, 371.0000, 372.0000, 373.0000, 374.0000, 375.0000, 376.0000, 377.0000, 378.0000, 379.0000, 380.0000, 381.0000, 382.0000, 383.0000, 384.0000, 385.0000, 386.0000, 387.0000, 388.0000, 389.0000, 390.0000, 391.0000, 392.0000, 393.0000, 394.0000, 395.0000, 396.0000, 397.0000, 398.0000, 399.0000, 400.0000, 401.0000, 402.0000, 403.0000, 404.0000, 405.0000, 406.0000, 407.0000, 408.0000, 409.0000, 410.0000, 411.0000, 412.0000, 413.0000, 414.0000, 415.0000, 416.0000, 417.0000, 418.0000, 419.0000, 420.0000, 421.0000, 422.0000, 423.0000, 424.0000, 425.0000, 426.0000, 427.0000, 428.0000, 429.0000, 430.0000, 431.0000, 432.0000, 433.0000, 434.0000, 435.0000, 436.0000, 437.0000, 438.0000, 439.0000, 440.0000, 441.0000, 442.0000, 443.0000, 444.0000, 445.0000, 446.0000, 447.0000, 448.0000, 449.0000, 450.0000, 451.0000, 452.0000, 453.0000, 454.0000, 455.0000, 456.0000, 457.0000, 458.0000, 459.0000, 460.0000, 461.0000, 462.0000, 463.0000, 464.0000, 465.0000, 466.0000, 467.0000, 468.0000, 469.0000, 470.0000, 471.0000, 472.0000, 473.0000, 474.0000, 475.0000, 476.0000, 477.0000, 478.0000, 479.0000, 480.0000, 481.0000, 482.0000, 483.0000, 484.0000, 485.0000, 486.0000, 487.0000, 488.0000, 489.0000, 490.0000, 491.0000, 492.0000, 493.0000, 494.0000, 495.0000, 496.0000, 497.0000, 498.0000, 499.0000, 500.0000, 501.0000, 502.0000, 503.0000, 504.0000, 505.0000, 506.0000, 507.0000, 508.0000, 509.0000, 510.0000, 511.0000, 512.0000, 513.0000, 514.0000, 515.0000, 516.0000, 517.0000, 518.0000, 519.0000, 520.0000, 521.0000, 522.0000, 523.0000, 524.0000, 525.0000, 526.0000, 527.0000, 528.0000, 529.0000, 530.0000, 531.0000, 532.0000, 533.0000, 534.0000, 535.0000, 536.0000, 537.0000, 538.0000, 539.0000, 540.0000, 541.0000, 542.0000, 543.0000, 544.0000, 545.0000, 546.0000, 547.0000, 548.0000, 549.0000, 550.0000, 551.0000, 552.0000, 553.0000, 554.0000, 555.0000, 556.0000, 557.0000, 558.0000, 559.0000, 560.0000, 561.0000, 562.0000, 563.0000, 564.0000, 565.0000, 566.0000, 567.0000, 568.0000, 569.0000, 570.0000, 571.0000, 572.0000, 573.0000, 574.0000, 575.0000, 576.0000, 577.0000, 578.0000, 579.0000, 580.0000, 581.0000, 582.0000, 583.0000, 584.0000, 585.0000, 586.0000, 587.0000, 588.0000, 589.0000, 590.0000, 591.0000, 592.0000, 593.0000, 594.0000, 595.0000, 596.0000, 597.0000, 598.0000, 599.0000, 600.0000, 601.0000, 602.0000, 603.0000, 604.0000, 605.0000, 606.0000, 607.0000, 608.0000, 609.0000, 610.0000, 611.0000, 612.0000, 613.0000, 614.0000, 615.0000, 616.0000, 617.0000, 618.0000, 619.0000, 620.0000, 621.0000, 622.0000, 623.0000, 624.0000, 625.0000, 626.0000, 627.0000, 628.0000, 629.0000, 630.0000, 631.0000, 632.0000, 633.0000, 634.0000, 635.0000, 636.0000, 637.0000, 638.0000, 639.0000, 640.0000, 641.0000, 642.0000, 643.0000, 644.0000, 645.0000, 646.0000, 647.0000, 648.0000, 649.0000, 650.0000, 651.0000, 652.0000, 653.0000, 654.0000, 655.0000, 656.0000, 657.0000, 658.0000, 659.0000, 660.0000, 661.0000, 662.0000, 663.0000, 664.0000, 665.0000, 666.0000, 667.0000, 668.0000, 669.0000, 670.0000, 671.0000, 672.0000, 673.0000, 674.0000, 675.0000, 676.0000, 677.0000, 678.0000, 679.0000, 680.0000, 681.0000, 682.0000, 683.0000, 684.0000, 685.0000, 686.0000, 687.0000, 688.0000, 689.0000, 690.0000, 691.0000, 692.0000, 693.0000, 694.0000, 695.0000, 696.0000, 697.0000, 698.0000, 699.0000, 700.0000, 701.0000, 702.0000, 703.0000, 704.0000, 705.0000, 706.0000, 707.0000, 708.0000, 709.0000, 710.0000, 711.0000, 712.0000, 713.0000, 714.0000, 715.0000, 716.0000, 717.0000, 718.0000, 719.0000, 720.0000, 721.0000, 722.0000, 723.0000, 724.0000, 725.0000, 726.0000, 727.0000, 728.0000, 729.0000, 730.0000, 731.0000, 732.0000, 733.0000, 734.0000, 735.0000, 736.0000, 737.0000, 738.0000, 739.0000, 740.0000, 741.0000, 742.0000, 743.0000, 744.0000, 745.0000, 746.0000, 747.0000, 748.0000, 749.0000, 750.0000, 751.0000, 752.0000, 753.0000, 754.0000, 755.0000, 756.0000, 757.0000, 758.0000, 759.0000, 760.0000, 761.0000, 762.0000, 763.0000, 764.0000, 765.0000, 766.0000, 767.0000, 768.0000, 769.0000, 770.0000, 771.0000, 772.0000, 773.0000, 774.0000, 775.0000, 776.0000, 777.0000, 778.0000, 779.0000, 780.0000, 781.0000, 782.0000, 783.0000, 784.0000, 785.0000, 786.0000, 787.0000, 788.0000, 789.0000, 790.0000, 791.0000, 792.0000, 793.0000, 794.0000, 795.0000, 796.0000, 797.0000, 798.0000, 799.0000, 800.0000, 801.0000, 802.0000, 803.0000, 804.0000, 805.0000, 806.0000, 807.0000, 808.0000, 809.0000, 810.0000, 811.0000, 812.0000, 813.0000, 814.0000, 815.0000, 816.0000, 817.0000, 818.0000, 819.0000, 820.0000, 821.0000, 822.0000, 823.0000, 824.0000, 825.0000, 826.0000, 827.0000, 828.0000, 829.0000, 830.0000, 831.0000, 832.0000, 833.0000, 834.0000, 835.0000, 836.0000, 837.0000, 838.0000, 839.0000, 840.0000, 841.0000, 842.0000, 843.0000, 844.0000, 845.0000, 846.0000, 847.0000, 848.0000, 849.0000, 850.0000, 851.0000, 852.0000, 853.0000, 854.0000, 855.0000, 856.0000, 857.0000, 858.0000, 859.0000, 860.0000, 861.0000, 862.0000, 863.0000, 864.0000, 865.0000, 866.0000, 867.0000, 868.0000, 869.0000, 870.0000, 871.0000, 872.0000, 873.0000, 874.0000, 875.0000, 876.0000, 877.0000, 878.0000, 879.0000, 880.0000, 881.0000, 882.0000, 883.0000, 884.0000, 885.0000, 886.0000, 887.0000, 888.0000, 889.0000, 890.0000, 891.0000, 892.0000, 893.0000, 894.0000, 895.0000, 896.0000, 897.0000, 898.0000, 899.0000, 900.0000, 901.0000, 902.0000, 903.0000, 904.0000, 905.0000, 906.0000, 907.0000, 908.0000, 909.0000, 910.0000, 911.0000, 912.0000, 913.0000, 914.0000, 915.0000, 916.0000, 917.0000, 918.0000, 919.0000, 920.0000, 921.0000, 922.0000, 923.0000, 924.0000, 925.0000, 926.0000, 927.0000, 928.0000, 929.0000, 930.0000, 931.0000, 932.0000, 933.0000, 934.0000, 935.0000, 936.0000, 937.0000, 938.0000, 939.0000, 940.0000, 941.0000, 942.0000, 943.0000, 944.0000, 945.0000, 946.0000, 947.0000, 948.0000, 949.0000, 950.0000, 951.0000, 952.0000, 953.0000, 954.0000, 955.0000, 956.0000, 957.0000, 958.0000, 959.0000, 960.0000, 961.0000, 962.0000, 963.0000, 964.0000, 965.0000, 966.0000, 967.0000, 968.0000, 969.0000, 970.0000, 971.0000, 972.0000, 973.0000, 974.0000, 975.0000, 976.0000, 977.0000, 978.0000, 979.0000, 980.0000, 981.0000, 982.0000, 983.0000, 984.0000, 985.0000, 986.0000, 987.0000, 988.0000, 989.0000, 990.0000, 991.0000, 992.0000, 993.0000, 994.0000, 995.0000, 996.0000, 997.0000, 998.0000, 999.0000, 1000.0000, 147.6804, 135.4462, 144.4982, 142.2999, 131.3720, 138.1702, 145.9028, 147.8446, 140.1299, 143.9491, 136.7238, 141.6579, 137.6851, 138.8044, 153.2209, 148.2796, 144.4877, 149.0437, 134.1845, 137.2250, 144.0198, 141.9399, 133.7013, 138.6256, 146.5406, 148.2432, 137.0206, 146.2836, 133.1701, 143.6919, 139.1812, 138.7402, 149.2203, 140.0426, 142.4198, 151.9551, 155.7528, 141.0111, 138.4382, 135.4988, 147.4635, 142.9939, 141.0986, 147.0697, 140.3843, 144.3258, 139.9294, 141.0263, 146.5828, 144.1515, 144.7555, 140.6972, 141.3981, 144.3291, 144.7822, 145.5400, 145.5009, 156.2744, 140.1324, 142.6911, 146.4823, 152.4072, 137.8974, 143.3219, 146.0311, 138.0387, 154.1120, 139.8144, 143.3750, 145.0294, 141.8342, 149.4891, 144.0271, 141.3668, 138.5126, 141.8681, 146.9804, 144.1151, 139.4643, 152.7997, 152.7765, 139.2076, 140.5657, 138.5018, 138.4191, 137.5269, 138.4375, 144.4698, 145.2724, 138.1107, 147.0641, 135.0405, 142.5538, 140.9958, 141.8685, 149.6061, 139.7731, 150.6989, 139.3553, 142.9409, 143.8204, 152.6034, 152.1782, 136.3172, 144.3167, 141.9819, 143.7289, 146.0748, 145.9691, 139.4729, 145.2498, 150.5981, 142.5599, 148.9535, 152.5185, 141.5589, 140.1030, 144.9223, 149.9054, 142.1724, 146.9517, 142.5394, 148.0107, 145.5233, 138.5003, 144.4994, 148.9948, 142.7340, 142.6655, 145.4729, 148.8790, 138.9647, 145.5253, 148.9470, 137.3071, 139.6391, 148.1526, 147.9501, 145.7091, 142.1188, 137.9109, 146.5551, 149.7664, 147.4748, 143.4276, 149.1081, 135.9570, 142.8416, 148.2843, 137.8721, 136.8754, 131.3449, 136.8765, 138.3773, 145.1378, 141.1114, 141.0767, 136.1309, 147.4010, 155.6832, 147.5787, 132.6802, 146.5783, 139.7703, 133.3792, 140.7256, 144.0016, 148.5753, 151.3330, 148.5547, 145.0855, 152.2895, 132.9454, 139.2151, 146.7865, 139.4730, 141.9397, 135.5694, 149.0032, 144.5622, 143.1022, 139.5529, 145.2285, 139.4092, 142.1410, 141.6317, 141.3532, 145.2355, 134.3562, 149.5594, 145.5915, 145.8178, 147.0731, 142.2528, 142.0940, 135.3817, 130.9934, 149.8679, 142.7992, 144.5194, 138.1459, 153.9490, 148.8849, 143.6801, 140.8819, 138.1119, 143.0580, 142.0813, 139.3931, 139.5283, 139.0627, 141.5214, 143.0682, 151.2332, 140.0663, 141.9577, 138.2848, 144.7828, 136.1422, 137.9434, 144.4177, 140.3416, 147.3807, 142.4938, 150.0804, 136.5054, 149.5794, 132.1571, 143.1229, 146.5362, 143.0555, 136.4487, 144.2783, 140.6974, 148.6313, 144.4664, 152.1715, 148.8232, 139.5856, 134.5230, 142.1475, 142.2972, 137.1402, 141.9795, 132.6220, 151.3743, 142.4447, 145.7663, 141.9486, 152.3305, 142.6366, 138.6015, 133.7251, 140.6698, 142.1466, 137.0833, 129.8893, 143.0310, 145.7198, 144.6113, 140.2492, 140.3778, 138.3838, 142.8852, 140.6231, 145.9288, 141.6831, 147.2276, 129.8496, 145.7425, 141.2945, 143.3332, 142.7722, 143.8973, 139.4288, 144.6086, 147.1328, 135.8990, 143.1654, 133.3797, 139.0511, 135.0828, 149.6272, 144.9449, 138.8819, 143.6803, 141.3252, 139.1097, 151.7199, 143.7309, 137.8109, 136.3634, 136.9375, 138.6749, 134.1299, 141.8829, 141.9147, 141.2257, 144.6542, 141.4363, 151.6558, 148.6059, 138.7876, 145.1405, 138.8753, 144.4364, 144.9731, 145.1662, 145.3468, 138.0669, 145.0557, 134.4359, 145.5510, 138.2587, 147.2537, 139.6596, 149.2239, 136.5466, 145.5777, 141.2121, 143.6721, 144.2991, 142.2597, 151.4015, 153.1509, 146.2184, 134.8998, 137.7955, 149.6967, 147.5260, 146.0526, 150.2019, 146.3060, 139.2831, 137.5699, 135.3626, 147.3250, 142.6359, 142.3398, 139.2721, 138.7872, 142.8012, 129.7119, 141.5616, 145.5671, 134.4478, 143.8132, 148.7879, 141.0996, 150.5848, 137.9382, 152.2154, 146.6381, 140.3818, 137.2524, 135.6152, 142.0346, 141.7250, 140.1414, 139.1182, 141.9489, 141.7654, 131.7956, 147.0496, 143.0977, 151.9297, 136.3620, 138.0245, 140.4971, 152.7501, 143.1680, 143.9126, 140.6030, 146.5863, 143.1301, 154.9907, 152.8965, 140.0854, 140.9577, 145.4784, 149.8864, 146.1341, 148.9554, 146.4137, 144.8140, 145.0213, 139.1463, 152.7263, 146.1302, 140.9384, 139.4969, 139.2113, 135.6136, 137.5246, 138.8286, 146.6597, 151.5274, 153.9596, 144.8928, 142.0703, 142.9364, 139.6338, 144.3458, 147.5707, 142.1128, 143.4695, 146.0058, 127.6209, 143.3985, 135.6464, 148.6850, 134.9874, 143.6395, 140.0447, 137.5390, 130.2813, 141.8271, 141.4175, 148.2740, 136.1943, 141.3700, 140.6267, 141.2005, 133.4379, 133.4014, 146.2581, 147.8788, 146.3411, 144.3350, 145.8550, 141.1697, 150.6670, 151.4806, 133.5444, 138.0000, 143.8524, 143.2749, 143.7852, 143.3914, 137.3603, 137.7751, 147.2389, 149.2571, 136.5207, 140.5065, 132.4089, 145.0828, 144.3362, 144.3615, 145.8266, 151.9455, 141.0895, 142.6910, 149.8435, 143.8557, 140.6875, 143.2761, 143.1077, 144.6642, 144.5762, 150.9277, 138.9424, 144.5282, 135.3842, 133.5553, 145.5309, 137.2899, 142.0356, 140.7052, 146.6446, 139.9486, 148.1112, 141.7850, 135.9844, 141.6492, 142.0235, 143.7194, 130.3133, 148.9807, 138.4825, 146.0382, 147.5849, 144.1840, 154.2811, 127.6615, 146.1478, 135.6630, 130.2916, 145.9319, 145.9895, 145.8076, 147.7932, 145.7117, 147.8781, 141.6669, 133.4594, 150.0932, 150.9903, 132.3827, 138.1996, 151.0113, 139.7248, 135.4531, 149.6610, 145.1980, 132.7888, 143.8974, 137.8966, 137.8156, 137.1754, 140.5713, 143.4905, 137.5953, 139.0430, 148.1153, 140.7627, 150.7582, 149.8491, 141.9844, 145.3081, 139.8275, 140.1045, 145.2517, 149.1655, 137.8665, 150.6819, 138.0362, 147.6704, 138.9455, 141.7432, 149.1226, 143.8683, 151.4607, 154.7108, 148.5743, 146.3620, 145.4927, 146.2319, 144.1914, 140.8519, 135.1874, 142.3940, 135.7443, 137.7177, 139.8587, 139.8244, 137.1412, 135.6044, 146.4525, 136.5875, 140.7420, 142.6878, 146.8024, 151.7215, 148.0156, 145.8367, 140.1791, 148.4605, 153.4981, 151.8093, 143.6618, 138.1080, 145.2531, 138.0496, 149.2298, 145.3250, 146.5159, 143.2761, 145.0890, 139.9311, 143.3637, 145.8897, 146.6270, 132.0916, 142.2635, 142.6689, 147.7313, 145.8808, 145.5879, 143.5535, 142.6939, 142.9098, 137.5010, 144.6075, 143.0404, 134.3331, 138.4387, 157.2938, 154.1740, 150.6551, 132.9050, 142.7284, 137.7726, 140.2537, 146.1841, 135.6242, 151.6318, 142.4177, 139.1922, 138.9971, 151.4860, 145.8211, 141.8217, 153.1420, 142.8269, 146.1340, 151.7530, 147.6149, 139.6834, 139.7013, 139.9005, 139.0850, 144.3542, 139.6271, 138.8138, 139.6128, 138.2317, 148.2532, 151.3962, 135.1432, 146.6154, 147.9444, 139.9700, 141.9969, 131.0681, 137.9788, 134.2533, 138.1383, 140.1167, 152.6678, 145.7752, 139.5092, 146.0247, 141.1212, 149.4793, 139.3235, 149.0996, 140.1768, 151.2639, 153.7263, 145.3439, 147.9354, 149.1348, 135.0774, 140.8488, 145.9629, 137.3131, 147.1690, 145.6152, 135.4688, 146.3481, 147.8967, 141.3454, 144.4693, 130.5750, 145.1256, 145.0850, 145.7232, 149.2489, 151.5950, 147.3756, 129.9418, 142.2180, 148.0945, 143.4832, 143.7076, 140.8828, 148.3101, 145.7432, 137.8748, 142.3533, 139.1454, 138.2937, 142.0423, 146.8824, 143.1366, 143.1667, 136.7788, 146.8361, 140.8992, 139.6966, 128.4170, 133.8752, 138.5926, 136.6902, 141.9105, 140.7367, 147.1372, 136.3326, 132.7656, 138.6459, 142.9634, 136.4982, 141.5198, 141.5298, 147.0990, 144.7485, 148.7238, 140.6486, 144.2897, 149.1517, 141.6510, 132.6372, 147.4952, 135.3989, 157.7863, 139.1631, 138.9151, 142.3222, 144.4572, 142.5811, 144.6089, 145.3894, 143.9995, 151.4075, 143.2628, 140.5183, 139.2208, 149.6776, 142.5105, 149.1005, 146.7930, 147.9987, 133.3823, 143.5704, 139.8573, 137.7300, 142.2334, 145.7547, 155.1068, 139.5740, 140.4386, 150.9087, 138.7470, 139.1888, 143.8933, 134.3876, 144.6376, 141.1824, 145.0780, 147.5425, 137.5720, 145.0860, 141.0185, 144.3457, 140.6326, 137.3329, 141.0114, 142.6038, 148.6589, 138.6288, 140.1463, 141.1404, 146.3710, 139.2050, 135.3051, 142.8131, 133.4538, 155.1783, 136.2713, 153.0035, 144.3105, 143.0442, 149.7068, 147.6006, 142.5149, 135.5762, 156.7109, 139.0670, 145.1734, 150.8543, 144.3546, 146.2368, 134.9482, 145.4235, 152.2813, 141.8379, 149.8791, 148.1024, 142.0939, 137.1234, 148.9225, 153.0383, 146.9607, 139.6056, 146.6701, 145.8919, 133.5489, 146.2152, 147.1474, 142.2497, 143.2529, 148.4035, 135.0032, 138.0883, 143.5424, 136.0450, 135.2965, 137.2004, 141.5955, 138.7552, 144.1411, 146.4168, 141.2732, 140.3767, 149.9072, 139.9201, 151.5980, 147.8926, 141.5243, 145.1301, 147.0507, 147.4276, 137.1708, 135.7575, 138.6885, 130.2011, 141.9829, 146.5357, 138.5714, 144.1879, 142.1541, 145.1574, 143.1461, 135.4393, 142.6440, 140.9792, 141.0660, 137.5708, 140.2738, 138.3632, 141.6954, 131.4040, 141.9666, 135.5470, 140.1131, 150.5804, 150.5706, 148.2934, 149.8499, 139.8932, 139.1903, 134.2728, 144.2480, 142.0472, 145.9113, 133.7000, 142.0302, 145.9845, 146.1468, 139.2691, 152.6256, 146.7822, 153.4117, 145.9766, 140.5414, 142.3196, 154.1813, 143.3567, 136.6891, 145.0610, 138.6295, 141.5392, 145.1124, 140.7501, 140.3491, 137.6499, 127.9936, 139.1690, 149.5117, 140.4319, 143.0121, 149.1758, 155.8103, 141.6497, 140.7867, 141.2840, 143.6347, 150.4483, 142.1214, 148.6653, 144.6846, 152.0415, 139.2480, 142.9907, 148.6360, 143.4434, 148.0689, 142.3603, 146.2299, 142.6435, 153.3589, 130.6310, 146.7212, 131.9949, 142.8330, 143.6880, 138.5835, 137.2426, 146.6870, 145.2673, 139.7730, 147.4993, 135.7217, 136.4861, 127.3776, 151.5626, 147.6468, 147.4764, 140.3191, 150.2898, 142.7067, 144.7663, 130.5575, 144.6897, 148.7805, 141.7709, 142.8096, 142.9498, 143.5552, 150.6377, 139.9408, 147.3415, 146.4235, 135.3258, 142.5487, 138.1896, 133.4927, 143.2078, 140.7838, 151.7623, 147.4939, 142.5936, 141.0650, 137.0189, 147.1829, 146.6509, 153.8811, 159.2416, 157.0624, 149.7221, 142.7634, 142.0579, 138.5773, 147.0467, 143.7896, 137.4271, 138.4411, 142.2016, 139.6799, 148.3218, 143.8003, 139.7548, 150.7715, 137.7348, 151.6863, 143.3366, 138.5527, 144.0041, 144.7190, 142.9818, 146.8088, 143.3703, 144.8944, 135.6770, 148.4229, 142.6195, 134.2666, 152.8309, 136.9611, 135.4615, 144.6284, 146.2948, 135.1051, 145.9748, 149.6942, 138.0852, 145.8967, 145.5348, 149.7858, 146.9535, 146.5745, 141.2558, 138.1688, 152.5663, 139.5757, 143.8045, 142.8213, 139.5376, 155.7237, 148.0596, 138.6540, 144.3046, 135.2062, 143.5869, 137.4008, 147.3257, 141.6972, 145.6421, 140.6420
## 3 1.0000, 2.0000, 3.0000, 4.0000, 5.0000, 6.0000, 7.0000, 8.0000, 9.0000, 10.0000, 11.0000, 12.0000, 13.0000, 14.0000, 15.0000, 16.0000, 17.0000, 18.0000, 19.0000, 20.0000, 21.0000, 22.0000, 23.0000, 24.0000, 25.0000, 26.0000, 27.0000, 28.0000, 29.0000, 30.0000, 31.0000, 32.0000, 33.0000, 34.0000, 35.0000, 36.0000, 37.0000, 38.0000, 39.0000, 40.0000, 41.0000, 42.0000, 43.0000, 44.0000, 45.0000, 46.0000, 47.0000, 48.0000, 49.0000, 50.0000, 51.0000, 52.0000, 53.0000, 54.0000, 55.0000, 56.0000, 57.0000, 58.0000, 59.0000, 60.0000, 61.0000, 62.0000, 63.0000, 64.0000, 65.0000, 66.0000, 67.0000, 68.0000, 69.0000, 70.0000, 71.0000, 72.0000, 73.0000, 74.0000, 75.0000, 76.0000, 77.0000, 78.0000, 79.0000, 80.0000, 81.0000, 82.0000, 83.0000, 84.0000, 85.0000, 86.0000, 87.0000, 88.0000, 89.0000, 90.0000, 91.0000, 92.0000, 93.0000, 94.0000, 95.0000, 96.0000, 97.0000, 98.0000, 99.0000, 100.0000, 101.0000, 102.0000, 103.0000, 104.0000, 105.0000, 106.0000, 107.0000, 108.0000, 109.0000, 110.0000, 111.0000, 112.0000, 113.0000, 114.0000, 115.0000, 116.0000, 117.0000, 118.0000, 119.0000, 120.0000, 121.0000, 122.0000, 123.0000, 124.0000, 125.0000, 126.0000, 127.0000, 128.0000, 129.0000, 130.0000, 131.0000, 132.0000, 133.0000, 134.0000, 135.0000, 136.0000, 137.0000, 138.0000, 139.0000, 140.0000, 141.0000, 142.0000, 143.0000, 144.0000, 145.0000, 146.0000, 147.0000, 148.0000, 149.0000, 150.0000, 151.0000, 152.0000, 153.0000, 154.0000, 155.0000, 156.0000, 157.0000, 158.0000, 159.0000, 160.0000, 161.0000, 162.0000, 163.0000, 164.0000, 165.0000, 166.0000, 167.0000, 168.0000, 169.0000, 170.0000, 171.0000, 172.0000, 173.0000, 174.0000, 175.0000, 176.0000, 177.0000, 178.0000, 179.0000, 180.0000, 181.0000, 182.0000, 183.0000, 184.0000, 185.0000, 186.0000, 187.0000, 188.0000, 189.0000, 190.0000, 191.0000, 192.0000, 193.0000, 194.0000, 195.0000, 196.0000, 197.0000, 198.0000, 199.0000, 200.0000, 201.0000, 202.0000, 203.0000, 204.0000, 205.0000, 206.0000, 207.0000, 208.0000, 209.0000, 210.0000, 211.0000, 212.0000, 213.0000, 214.0000, 215.0000, 216.0000, 217.0000, 218.0000, 219.0000, 220.0000, 221.0000, 222.0000, 223.0000, 224.0000, 225.0000, 226.0000, 227.0000, 228.0000, 229.0000, 230.0000, 231.0000, 232.0000, 233.0000, 234.0000, 235.0000, 236.0000, 237.0000, 238.0000, 239.0000, 240.0000, 241.0000, 242.0000, 243.0000, 244.0000, 245.0000, 246.0000, 247.0000, 248.0000, 249.0000, 250.0000, 251.0000, 252.0000, 253.0000, 254.0000, 255.0000, 256.0000, 257.0000, 258.0000, 259.0000, 260.0000, 261.0000, 262.0000, 263.0000, 264.0000, 265.0000, 266.0000, 267.0000, 268.0000, 269.0000, 270.0000, 271.0000, 272.0000, 273.0000, 274.0000, 275.0000, 276.0000, 277.0000, 278.0000, 279.0000, 280.0000, 281.0000, 282.0000, 283.0000, 284.0000, 285.0000, 286.0000, 287.0000, 288.0000, 289.0000, 290.0000, 291.0000, 292.0000, 293.0000, 294.0000, 295.0000, 296.0000, 297.0000, 298.0000, 299.0000, 300.0000, 301.0000, 302.0000, 303.0000, 304.0000, 305.0000, 306.0000, 307.0000, 308.0000, 309.0000, 310.0000, 311.0000, 312.0000, 313.0000, 314.0000, 315.0000, 316.0000, 317.0000, 318.0000, 319.0000, 320.0000, 321.0000, 322.0000, 323.0000, 324.0000, 325.0000, 326.0000, 327.0000, 328.0000, 329.0000, 330.0000, 331.0000, 332.0000, 333.0000, 334.0000, 335.0000, 336.0000, 337.0000, 338.0000, 339.0000, 340.0000, 341.0000, 342.0000, 343.0000, 344.0000, 345.0000, 346.0000, 347.0000, 348.0000, 349.0000, 350.0000, 351.0000, 352.0000, 353.0000, 354.0000, 355.0000, 356.0000, 357.0000, 358.0000, 359.0000, 360.0000, 361.0000, 362.0000, 363.0000, 364.0000, 365.0000, 366.0000, 367.0000, 368.0000, 369.0000, 370.0000, 371.0000, 372.0000, 373.0000, 374.0000, 375.0000, 376.0000, 377.0000, 378.0000, 379.0000, 380.0000, 381.0000, 382.0000, 383.0000, 384.0000, 385.0000, 386.0000, 387.0000, 388.0000, 389.0000, 390.0000, 391.0000, 392.0000, 393.0000, 394.0000, 395.0000, 396.0000, 397.0000, 398.0000, 399.0000, 400.0000, 401.0000, 402.0000, 403.0000, 404.0000, 405.0000, 406.0000, 407.0000, 408.0000, 409.0000, 410.0000, 411.0000, 412.0000, 413.0000, 414.0000, 415.0000, 416.0000, 417.0000, 418.0000, 419.0000, 420.0000, 421.0000, 422.0000, 423.0000, 424.0000, 425.0000, 426.0000, 427.0000, 428.0000, 429.0000, 430.0000, 431.0000, 432.0000, 433.0000, 434.0000, 435.0000, 436.0000, 437.0000, 438.0000, 439.0000, 440.0000, 441.0000, 442.0000, 443.0000, 444.0000, 445.0000, 446.0000, 447.0000, 448.0000, 449.0000, 450.0000, 451.0000, 452.0000, 453.0000, 454.0000, 455.0000, 456.0000, 457.0000, 458.0000, 459.0000, 460.0000, 461.0000, 462.0000, 463.0000, 464.0000, 465.0000, 466.0000, 467.0000, 468.0000, 469.0000, 470.0000, 471.0000, 472.0000, 473.0000, 474.0000, 475.0000, 476.0000, 477.0000, 478.0000, 479.0000, 480.0000, 481.0000, 482.0000, 483.0000, 484.0000, 485.0000, 486.0000, 487.0000, 488.0000, 489.0000, 490.0000, 491.0000, 492.0000, 493.0000, 494.0000, 495.0000, 496.0000, 497.0000, 498.0000, 499.0000, 500.0000, 501.0000, 502.0000, 503.0000, 504.0000, 505.0000, 506.0000, 507.0000, 508.0000, 509.0000, 510.0000, 511.0000, 512.0000, 513.0000, 514.0000, 515.0000, 516.0000, 517.0000, 518.0000, 519.0000, 520.0000, 521.0000, 522.0000, 523.0000, 524.0000, 525.0000, 526.0000, 527.0000, 528.0000, 529.0000, 530.0000, 531.0000, 532.0000, 533.0000, 534.0000, 535.0000, 536.0000, 537.0000, 538.0000, 539.0000, 540.0000, 541.0000, 542.0000, 543.0000, 544.0000, 545.0000, 546.0000, 547.0000, 548.0000, 549.0000, 550.0000, 551.0000, 552.0000, 553.0000, 554.0000, 555.0000, 556.0000, 557.0000, 558.0000, 559.0000, 560.0000, 561.0000, 562.0000, 563.0000, 564.0000, 565.0000, 566.0000, 567.0000, 568.0000, 569.0000, 570.0000, 571.0000, 572.0000, 573.0000, 574.0000, 575.0000, 576.0000, 577.0000, 578.0000, 579.0000, 580.0000, 581.0000, 582.0000, 583.0000, 584.0000, 585.0000, 586.0000, 587.0000, 588.0000, 589.0000, 590.0000, 591.0000, 592.0000, 593.0000, 594.0000, 595.0000, 596.0000, 597.0000, 598.0000, 599.0000, 600.0000, 601.0000, 602.0000, 603.0000, 604.0000, 605.0000, 606.0000, 607.0000, 608.0000, 609.0000, 610.0000, 611.0000, 612.0000, 613.0000, 614.0000, 615.0000, 616.0000, 617.0000, 618.0000, 619.0000, 620.0000, 621.0000, 622.0000, 623.0000, 624.0000, 625.0000, 626.0000, 627.0000, 628.0000, 629.0000, 630.0000, 631.0000, 632.0000, 633.0000, 634.0000, 635.0000, 636.0000, 637.0000, 638.0000, 639.0000, 640.0000, 641.0000, 642.0000, 643.0000, 644.0000, 645.0000, 646.0000, 647.0000, 648.0000, 649.0000, 650.0000, 651.0000, 652.0000, 653.0000, 654.0000, 655.0000, 656.0000, 657.0000, 658.0000, 659.0000, 660.0000, 661.0000, 662.0000, 663.0000, 664.0000, 665.0000, 666.0000, 667.0000, 668.0000, 669.0000, 670.0000, 671.0000, 672.0000, 673.0000, 674.0000, 675.0000, 676.0000, 677.0000, 678.0000, 679.0000, 680.0000, 681.0000, 682.0000, 683.0000, 684.0000, 685.0000, 686.0000, 687.0000, 688.0000, 689.0000, 690.0000, 691.0000, 692.0000, 693.0000, 694.0000, 695.0000, 696.0000, 697.0000, 698.0000, 699.0000, 700.0000, 701.0000, 702.0000, 703.0000, 704.0000, 705.0000, 706.0000, 707.0000, 708.0000, 709.0000, 710.0000, 711.0000, 712.0000, 713.0000, 714.0000, 715.0000, 716.0000, 717.0000, 718.0000, 719.0000, 720.0000, 721.0000, 722.0000, 723.0000, 724.0000, 725.0000, 726.0000, 727.0000, 728.0000, 729.0000, 730.0000, 731.0000, 732.0000, 733.0000, 734.0000, 735.0000, 736.0000, 737.0000, 738.0000, 739.0000, 740.0000, 741.0000, 742.0000, 743.0000, 744.0000, 745.0000, 746.0000, 747.0000, 748.0000, 749.0000, 750.0000, 751.0000, 752.0000, 753.0000, 754.0000, 755.0000, 756.0000, 757.0000, 758.0000, 759.0000, 760.0000, 761.0000, 762.0000, 763.0000, 764.0000, 765.0000, 766.0000, 767.0000, 768.0000, 769.0000, 770.0000, 771.0000, 772.0000, 773.0000, 774.0000, 775.0000, 776.0000, 777.0000, 778.0000, 779.0000, 780.0000, 781.0000, 782.0000, 783.0000, 784.0000, 785.0000, 786.0000, 787.0000, 788.0000, 789.0000, 790.0000, 791.0000, 792.0000, 793.0000, 794.0000, 795.0000, 796.0000, 797.0000, 798.0000, 799.0000, 800.0000, 801.0000, 802.0000, 803.0000, 804.0000, 805.0000, 806.0000, 807.0000, 808.0000, 809.0000, 810.0000, 811.0000, 812.0000, 813.0000, 814.0000, 815.0000, 816.0000, 817.0000, 818.0000, 819.0000, 820.0000, 821.0000, 822.0000, 823.0000, 824.0000, 825.0000, 826.0000, 827.0000, 828.0000, 829.0000, 830.0000, 831.0000, 832.0000, 833.0000, 834.0000, 835.0000, 836.0000, 837.0000, 838.0000, 839.0000, 840.0000, 841.0000, 842.0000, 843.0000, 844.0000, 845.0000, 846.0000, 847.0000, 848.0000, 849.0000, 850.0000, 851.0000, 852.0000, 853.0000, 854.0000, 855.0000, 856.0000, 857.0000, 858.0000, 859.0000, 860.0000, 861.0000, 862.0000, 863.0000, 864.0000, 865.0000, 866.0000, 867.0000, 868.0000, 869.0000, 870.0000, 871.0000, 872.0000, 873.0000, 874.0000, 875.0000, 876.0000, 877.0000, 878.0000, 879.0000, 880.0000, 881.0000, 882.0000, 883.0000, 884.0000, 885.0000, 886.0000, 887.0000, 888.0000, 889.0000, 890.0000, 891.0000, 892.0000, 893.0000, 894.0000, 895.0000, 896.0000, 897.0000, 898.0000, 899.0000, 900.0000, 901.0000, 902.0000, 903.0000, 904.0000, 905.0000, 906.0000, 907.0000, 908.0000, 909.0000, 910.0000, 911.0000, 912.0000, 913.0000, 914.0000, 915.0000, 916.0000, 917.0000, 918.0000, 919.0000, 920.0000, 921.0000, 922.0000, 923.0000, 924.0000, 925.0000, 926.0000, 927.0000, 928.0000, 929.0000, 930.0000, 931.0000, 932.0000, 933.0000, 934.0000, 935.0000, 936.0000, 937.0000, 938.0000, 939.0000, 940.0000, 941.0000, 942.0000, 943.0000, 944.0000, 945.0000, 946.0000, 947.0000, 948.0000, 949.0000, 950.0000, 951.0000, 952.0000, 953.0000, 954.0000, 955.0000, 956.0000, 957.0000, 958.0000, 959.0000, 960.0000, 961.0000, 962.0000, 963.0000, 964.0000, 965.0000, 966.0000, 967.0000, 968.0000, 969.0000, 970.0000, 971.0000, 972.0000, 973.0000, 974.0000, 975.0000, 976.0000, 977.0000, 978.0000, 979.0000, 980.0000, 981.0000, 982.0000, 983.0000, 984.0000, 985.0000, 986.0000, 987.0000, 988.0000, 989.0000, 990.0000, 991.0000, 992.0000, 993.0000, 994.0000, 995.0000, 996.0000, 997.0000, 998.0000, 999.0000, 1000.0000, 144.7983, 138.6767, 152.6394, 135.6332, 149.1064, 137.0215, 142.1552, 150.0871, 149.1258, 142.0005, 132.3170, 149.0333, 155.9795, 133.4516, 146.1256, 142.8271, 143.3071, 145.3490, 153.1479, 144.7863, 140.2209, 142.6473, 147.6848, 143.0596, 138.4045, 136.9704, 145.6004, 150.2076, 144.8125, 139.9447, 144.2523, 147.7587, 138.7948, 153.5560, 141.9707, 149.1887, 146.3953, 147.0893, 140.1916, 143.7809, 130.1956, 141.7777, 152.5129, 157.6510, 135.1141, 139.7453, 147.4949, 149.1300, 142.5516, 157.8247, 139.4342, 137.0114, 138.2193, 138.5438, 151.4625, 140.8785, 144.6304, 141.4132, 135.0050, 143.9216, 146.6865, 141.6268, 128.5845, 148.9558, 144.8354, 147.9564, 142.9388, 140.2304, 142.7145, 141.3375, 143.3088, 137.7476, 148.3836, 148.3228, 142.3674, 146.8333, 132.9270, 141.9492, 139.4882, 143.9051, 145.2126, 144.8092, 139.7337, 142.7233, 151.9209, 145.9871, 138.6751, 140.5749, 141.1379, 143.1329, 139.0791, 139.3530, 149.4328, 142.9193, 148.4688, 144.4971, 147.9792, 148.7454, 141.3222, 134.7381, 140.6445, 141.1485, 137.8561, 145.9780, 134.7705, 147.8615, 147.8743, 148.6681, 143.2505, 134.5304, 136.6388, 141.4256, 132.5363, 141.3640, 144.5569, 140.4337, 140.0217, 144.9762, 141.8679, 139.0539, 142.0754, 143.4110, 145.4920, 139.4878, 146.6869, 144.2456, 139.4655, 136.5578, 139.4163, 148.5236, 140.2374, 139.3612, 154.7723, 137.3370, 135.0353, 149.5359, 139.0823, 139.4826, 148.5762, 142.2360, 141.5586, 146.8122, 143.5661, 142.0530, 139.4150, 143.7478, 147.0972, 143.1951, 146.6456, 149.3067, 137.4548, 137.0685, 147.3031, 139.0946, 142.3426, 139.2238, 145.1033, 140.1989, 139.7859, 150.6620, 141.9079, 144.1209, 138.2217, 146.0978, 141.7066, 147.8734, 142.5094, 141.8817, 141.8304, 136.5011, 144.2594, 147.8655, 142.2071, 143.1041, 148.9575, 142.1117, 137.8373, 144.2913, 147.0014, 137.4657, 139.7691, 138.5353, 140.8066, 133.8400, 139.4086, 140.4819, 146.9182, 146.9518, 146.7573, 147.8923, 134.6494, 153.5530, 145.0593, 145.7243, 150.8347, 143.0928, 138.4696, 139.9458, 135.8756, 150.4562, 147.7701, 145.5454, 142.3851, 153.3748, 149.6618, 147.9915, 136.1285, 138.5136, 145.9859, 145.8549, 136.1652, 136.7610, 143.6369, 134.4863, 147.6780, 144.4666, 137.6892, 135.7773, 144.4447, 143.3153, 143.7642, 142.4140, 133.8453, 141.0183, 143.4312, 137.9044, 147.4237, 147.6972, 137.7796, 149.4967, 155.9813, 142.6181, 148.6237, 143.2904, 146.8924, 148.0345, 147.4860, 146.3200, 150.9755, 145.4997, 137.5561, 149.0097, 139.6883, 141.2329, 143.0389, 151.9796, 141.3750, 147.2251, 155.3078, 148.8180, 141.7858, 140.9791, 139.8362, 137.4993, 147.3941, 141.8090, 144.1634, 142.3857, 142.3288, 150.6296, 146.8683, 145.0461, 147.5301, 141.6883, 143.6189, 142.8173, 147.2013, 150.9634, 136.6504, 149.8048, 143.6781, 153.8523, 143.4962, 144.5926, 145.9470, 142.9935, 136.7449, 144.6858, 147.4522, 143.1181, 146.5103, 133.7020, 142.9511, 140.9275, 143.6268, 146.9516, 137.0967, 146.0115, 135.6692, 136.1766, 138.8812, 152.5367, 151.9931, 138.9048, 137.3246, 138.0266, 139.4750, 146.5465, 139.5188, 149.5367, 141.6812, 141.4711, 138.7701, 151.8079, 144.9760, 148.4499, 150.2652, 143.7585, 150.6876, 142.6689, 144.0372, 138.4255, 141.7773, 134.8635, 138.1145, 144.9907, 148.7244, 141.5751, 147.0550, 147.4795, 145.0920, 140.6237, 137.9174, 143.9964, 145.4050, 144.3232, 143.8193, 146.4210, 136.5090, 140.0248, 142.5576, 142.5512, 143.6356, 154.3696, 140.1776, 147.8252, 146.5594, 140.7851, 139.9385, 137.9849, 135.5533, 143.4835, 135.3511, 139.6672, 133.1760, 139.0046, 152.1071, 140.6867, 141.3213, 148.3799, 140.5558, 139.2168, 144.4689, 145.2331, 140.1524, 149.9222, 144.0936, 138.1799, 142.3568, 138.8601, 148.9038, 143.4853, 148.2850, 137.3374, 147.1255, 144.1609, 139.9266, 151.3291, 141.9605, 149.7950, 132.7304, 143.2751, 141.6696, 140.3717, 142.2711, 139.1542, 144.0368, 135.5626, 144.9398, 137.8380, 147.5132, 145.1583, 143.5981, 137.2509, 150.3161, 148.9453, 148.5842, 145.7541, 148.9939, 132.9875, 138.6158, 142.5024, 151.6839, 133.9589, 141.0535, 148.0473, 137.1148, 149.5720, 147.3871, 138.5814, 141.7201, 136.3510, 145.1507, 141.1198, 154.3050, 136.1265, 145.9113, 134.6360, 147.3870, 138.3218, 144.7805, 142.4330, 142.2940, 148.8835, 145.9229, 142.9017, 138.4571, 147.7116, 144.0063, 148.0583, 136.7534, 145.1106, 147.5452, 145.1667, 148.2541, 143.1114, 140.0594, 142.7019, 146.6039, 141.1434, 143.1848, 136.0789, 134.0607, 140.3915, 136.6176, 137.3959, 131.9578, 139.8296, 142.3073, 143.6127, 144.1679, 136.3396, 151.6683, 138.1500, 146.4415, 147.3729, 143.2456, 129.7145, 137.3746, 146.7328, 145.6437, 143.3675, 147.4925, 130.2152, 139.2783, 143.1915, 141.7587, 150.9343, 140.8922, 140.8398, 140.9994, 141.4164, 142.4136, 149.0312, 146.6676, 132.3871, 141.1387, 151.8794, 152.7071, 145.4138, 144.3829, 132.5216, 134.2495, 136.6406, 140.2194, 128.7469, 141.6512, 147.1660, 147.2288, 137.1741, 149.6269, 146.0357, 146.8116, 143.9546, 140.4208, 146.9893, 143.8438, 138.9750, 136.5154, 135.2862, 147.0035, 147.2642, 149.1537, 141.6812, 141.4074, 140.2921, 142.4871, 145.6428, 136.5004, 145.7277, 133.6598, 154.9090, 149.1334, 136.2027, 148.6249, 146.8425, 140.5961, 139.7966, 151.5320, 142.4504, 143.7463, 136.4220, 145.8713, 135.6442, 144.9926, 148.6909, 140.6490, 144.9859, 142.1528, 140.4243, 142.4007, 148.4181, 140.5474, 149.8740, 146.3190, 145.6531, 148.1327, 139.4718, 142.4125, 138.1961, 143.1236, 145.5421, 141.1539, 151.4048, 136.0285, 138.5523, 130.7808, 136.9124, 144.7107, 156.1585, 148.1188, 139.5780, 138.9897, 139.4716, 146.8944, 145.3181, 142.6941, 138.4295, 141.5082, 146.7470, 142.0610, 145.3681, 151.2530, 147.3916, 142.7686, 147.5642, 144.5554, 140.2894, 148.2406, 133.2943, 133.4312, 141.3045, 143.7979, 147.3733, 147.9101, 141.2164, 138.1197, 157.7766, 142.2510, 141.7686, 138.7746, 146.1691, 148.5257, 141.3390, 153.4292, 139.0449, 137.9919, 146.4980, 132.9997, 145.8954, 142.9433, 145.9793, 149.0647, 137.2431, 143.4962, 146.0151, 140.3154, 151.8479, 142.8849, 140.2971, 148.1377, 153.3404, 146.3788, 145.1343, 146.7794, 149.0136, 135.2308, 140.3533, 135.2467, 138.9391, 141.0306, 141.3637, 138.5730, 139.7069, 148.8026, 139.4692, 146.2596, 137.0269, 148.2887, 139.4864, 144.1850, 150.3804, 142.4133, 146.6314, 142.1427, 141.0789, 135.7859, 148.5628, 137.9511, 147.4398, 145.4625, 144.1441, 147.0645, 143.2487, 143.4762, 140.6862, 139.3753, 139.5687, 148.7664, 138.2274, 139.9350, 136.8191, 154.3158, 141.4099, 143.0712, 153.7991, 143.5521, 140.3569, 144.0146, 140.9364, 145.5443, 134.0444, 136.5871, 142.6535, 145.8233, 127.1722, 142.7092, 144.6328, 141.7591, 142.5308, 145.3937, 143.6013, 140.3954, 150.1644, 133.8422, 142.0663, 146.6883, 143.4795, 156.5505, 134.6022, 140.9448, 137.0220, 146.3031, 146.6587, 142.9091, 138.4747, 145.5042, 141.9982, 142.9190, 148.0926, 140.7031, 147.2888, 154.0055, 142.5635, 140.8653, 148.6446, 142.9078, 143.0744, 141.7436, 140.4658, 153.7230, 141.7914, 147.7131, 157.1720, 131.1230, 142.5549, 147.9286, 142.4063, 138.7445, 145.2202, 149.7323, 138.9550, 147.2419, 140.0430, 149.0712, 149.1299, 139.7834, 145.6308, 138.1693, 147.3210, 139.4809, 138.7693, 142.8312, 140.1707, 138.4042, 151.0566, 139.3867, 146.4026, 142.9936, 152.9162, 140.9330, 143.2708, 147.2174, 137.4610, 141.1796, 150.1991, 138.9704, 142.7709, 140.2575, 153.3711, 143.4702, 139.2050, 145.2381, 142.1366, 143.8973, 133.5195, 141.1466, 143.8562, 152.3219, 146.4950, 141.4300, 137.1355, 135.1206, 147.5666, 146.5483, 145.5467, 151.8345, 149.3589, 145.6941, 150.2299, 146.7353, 138.6155, 148.7993, 148.4423, 131.3186, 139.1974, 148.8708, 154.5663, 140.4862, 152.0501, 139.9177, 148.6855, 154.4274, 147.7286, 136.9714, 144.6001, 139.2817, 149.8073, 144.9758, 149.0226, 144.4803, 150.9153, 141.6952, 136.7872, 147.3475, 137.0210, 137.1716, 142.9019, 140.2073, 146.3858, 151.6929, 146.7527, 143.7366, 143.2209, 138.5326, 144.7201, 154.3265, 152.6964, 137.1765, 145.3234, 140.0829, 148.1685, 146.8211, 147.6328, 143.1247, 144.3306, 146.4536, 139.8171, 149.9996, 142.8315, 142.4692, 138.8151, 142.8859, 145.1314, 148.9324, 143.3651, 144.6509, 135.3027, 141.5319, 143.6261, 145.8512, 142.2304, 148.4354, 142.0726, 143.6085, 144.7953, 143.3869, 139.8184, 150.7534, 139.0502, 137.9995, 143.4355, 148.4313, 148.8592, 140.8879, 146.1442, 151.1100, 142.1547, 135.5422, 151.0770, 145.9955, 142.4830, 144.5181, 149.0661, 144.8422, 148.8599, 137.2348, 145.0009, 147.1895, 148.9823, 146.2351, 138.5270, 141.0645, 148.2304, 140.7777, 146.6521, 145.1471, 147.3010, 139.1977, 137.7927, 134.6509, 152.2540, 153.1023, 136.7785, 138.2663, 137.7309, 134.0933, 135.0646, 148.0308, 150.0588, 150.3779, 152.0888, 143.7006, 144.0136, 148.6137, 144.7419, 149.6161, 143.9640, 146.4649, 152.7426, 137.7860, 143.6792, 147.0986, 147.9685, 141.9243, 132.3899, 142.6710, 144.6674, 144.1624, 140.3391, 152.4304, 154.2383, 151.8970, 141.9261, 141.6889, 149.3028, 141.4014, 141.1064, 135.0032, 140.9640, 140.3196, 142.2620, 136.3411, 149.9654, 139.9130, 140.9932, 147.4851, 145.2188, 137.0714, 147.4354, 144.0922, 146.7940, 145.3933, 146.9221, 141.7312, 148.6638, 149.5833, 151.6410, 141.0798, 130.4974, 139.1539, 145.3657, 139.6977, 148.9264, 146.7695, 145.7419, 152.1278, 145.6939, 134.6927, 146.5098, 140.3473, 135.2821, 138.9491, 138.6282, 135.0836, 152.4398, 137.6106, 139.9343, 143.3179, 147.2043, 144.7875, 147.2122, 149.0765, 146.4950, 141.7173, 146.5020, 145.6937, 138.8656, 138.6140, 142.0396, 145.1161, 142.5084, 142.8907, 145.5683, 132.0693, 140.2990, 142.3580, 151.2486, 151.1188, 144.5171, 134.5754, 143.3881, 134.2938, 138.6918, 139.6720, 139.2596, 143.4887, 142.8981, 142.9900, 139.6543, 148.5329, 147.0789, 149.0937, 142.8173, 145.0832, 142.7014, 129.2738, 144.0875, 143.8508, 145.5753, 136.1176, 139.8349, 153.0771, 143.7986, 144.2154, 141.6859, 138.0630, 149.3111, 145.5636, 137.0444, 143.7197, 148.6808, 141.0754, 131.6767, 142.2135, 141.6869, 145.9587, 140.8130, 148.0014, 140.2741, 142.7464, 144.9899, 142.3381, 137.8010, 136.3296, 147.7928, 134.4579, 142.6114, 133.9180, 151.2164, 141.0230, 146.8727, 134.4310, 137.6906, 139.0450, 140.6577, 141.7280, 134.7486, 149.5284, 142.1743
## 4 1.0000, 2.0000, 3.0000, 4.0000, 5.0000, 6.0000, 7.0000, 8.0000, 9.0000, 10.0000, 11.0000, 12.0000, 13.0000, 14.0000, 15.0000, 16.0000, 17.0000, 18.0000, 19.0000, 20.0000, 21.0000, 22.0000, 23.0000, 24.0000, 25.0000, 26.0000, 27.0000, 28.0000, 29.0000, 30.0000, 31.0000, 32.0000, 33.0000, 34.0000, 35.0000, 36.0000, 37.0000, 38.0000, 39.0000, 40.0000, 41.0000, 42.0000, 43.0000, 44.0000, 45.0000, 46.0000, 47.0000, 48.0000, 49.0000, 50.0000, 51.0000, 52.0000, 53.0000, 54.0000, 55.0000, 56.0000, 57.0000, 58.0000, 59.0000, 60.0000, 61.0000, 62.0000, 63.0000, 64.0000, 65.0000, 66.0000, 67.0000, 68.0000, 69.0000, 70.0000, 71.0000, 72.0000, 73.0000, 74.0000, 75.0000, 76.0000, 77.0000, 78.0000, 79.0000, 80.0000, 81.0000, 82.0000, 83.0000, 84.0000, 85.0000, 86.0000, 87.0000, 88.0000, 89.0000, 90.0000, 91.0000, 92.0000, 93.0000, 94.0000, 95.0000, 96.0000, 97.0000, 98.0000, 99.0000, 100.0000, 101.0000, 102.0000, 103.0000, 104.0000, 105.0000, 106.0000, 107.0000, 108.0000, 109.0000, 110.0000, 111.0000, 112.0000, 113.0000, 114.0000, 115.0000, 116.0000, 117.0000, 118.0000, 119.0000, 120.0000, 121.0000, 122.0000, 123.0000, 124.0000, 125.0000, 126.0000, 127.0000, 128.0000, 129.0000, 130.0000, 131.0000, 132.0000, 133.0000, 134.0000, 135.0000, 136.0000, 137.0000, 138.0000, 139.0000, 140.0000, 141.0000, 142.0000, 143.0000, 144.0000, 145.0000, 146.0000, 147.0000, 148.0000, 149.0000, 150.0000, 151.0000, 152.0000, 153.0000, 154.0000, 155.0000, 156.0000, 157.0000, 158.0000, 159.0000, 160.0000, 161.0000, 162.0000, 163.0000, 164.0000, 165.0000, 166.0000, 167.0000, 168.0000, 169.0000, 170.0000, 171.0000, 172.0000, 173.0000, 174.0000, 175.0000, 176.0000, 177.0000, 178.0000, 179.0000, 180.0000, 181.0000, 182.0000, 183.0000, 184.0000, 185.0000, 186.0000, 187.0000, 188.0000, 189.0000, 190.0000, 191.0000, 192.0000, 193.0000, 194.0000, 195.0000, 196.0000, 197.0000, 198.0000, 199.0000, 200.0000, 201.0000, 202.0000, 203.0000, 204.0000, 205.0000, 206.0000, 207.0000, 208.0000, 209.0000, 210.0000, 211.0000, 212.0000, 213.0000, 214.0000, 215.0000, 216.0000, 217.0000, 218.0000, 219.0000, 220.0000, 221.0000, 222.0000, 223.0000, 224.0000, 225.0000, 226.0000, 227.0000, 228.0000, 229.0000, 230.0000, 231.0000, 232.0000, 233.0000, 234.0000, 235.0000, 236.0000, 237.0000, 238.0000, 239.0000, 240.0000, 241.0000, 242.0000, 243.0000, 244.0000, 245.0000, 246.0000, 247.0000, 248.0000, 249.0000, 250.0000, 251.0000, 252.0000, 253.0000, 254.0000, 255.0000, 256.0000, 257.0000, 258.0000, 259.0000, 260.0000, 261.0000, 262.0000, 263.0000, 264.0000, 265.0000, 266.0000, 267.0000, 268.0000, 269.0000, 270.0000, 271.0000, 272.0000, 273.0000, 274.0000, 275.0000, 276.0000, 277.0000, 278.0000, 279.0000, 280.0000, 281.0000, 282.0000, 283.0000, 284.0000, 285.0000, 286.0000, 287.0000, 288.0000, 289.0000, 290.0000, 291.0000, 292.0000, 293.0000, 294.0000, 295.0000, 296.0000, 297.0000, 298.0000, 299.0000, 300.0000, 301.0000, 302.0000, 303.0000, 304.0000, 305.0000, 306.0000, 307.0000, 308.0000, 309.0000, 310.0000, 311.0000, 312.0000, 313.0000, 314.0000, 315.0000, 316.0000, 317.0000, 318.0000, 319.0000, 320.0000, 321.0000, 322.0000, 323.0000, 324.0000, 325.0000, 326.0000, 327.0000, 328.0000, 329.0000, 330.0000, 331.0000, 332.0000, 333.0000, 334.0000, 335.0000, 336.0000, 337.0000, 338.0000, 339.0000, 340.0000, 341.0000, 342.0000, 343.0000, 344.0000, 345.0000, 346.0000, 347.0000, 348.0000, 349.0000, 350.0000, 351.0000, 352.0000, 353.0000, 354.0000, 355.0000, 356.0000, 357.0000, 358.0000, 359.0000, 360.0000, 361.0000, 362.0000, 363.0000, 364.0000, 365.0000, 366.0000, 367.0000, 368.0000, 369.0000, 370.0000, 371.0000, 372.0000, 373.0000, 374.0000, 375.0000, 376.0000, 377.0000, 378.0000, 379.0000, 380.0000, 381.0000, 382.0000, 383.0000, 384.0000, 385.0000, 386.0000, 387.0000, 388.0000, 389.0000, 390.0000, 391.0000, 392.0000, 393.0000, 394.0000, 395.0000, 396.0000, 397.0000, 398.0000, 399.0000, 400.0000, 401.0000, 402.0000, 403.0000, 404.0000, 405.0000, 406.0000, 407.0000, 408.0000, 409.0000, 410.0000, 411.0000, 412.0000, 413.0000, 414.0000, 415.0000, 416.0000, 417.0000, 418.0000, 419.0000, 420.0000, 421.0000, 422.0000, 423.0000, 424.0000, 425.0000, 426.0000, 427.0000, 428.0000, 429.0000, 430.0000, 431.0000, 432.0000, 433.0000, 434.0000, 435.0000, 436.0000, 437.0000, 438.0000, 439.0000, 440.0000, 441.0000, 442.0000, 443.0000, 444.0000, 445.0000, 446.0000, 447.0000, 448.0000, 449.0000, 450.0000, 451.0000, 452.0000, 453.0000, 454.0000, 455.0000, 456.0000, 457.0000, 458.0000, 459.0000, 460.0000, 461.0000, 462.0000, 463.0000, 464.0000, 465.0000, 466.0000, 467.0000, 468.0000, 469.0000, 470.0000, 471.0000, 472.0000, 473.0000, 474.0000, 475.0000, 476.0000, 477.0000, 478.0000, 479.0000, 480.0000, 481.0000, 482.0000, 483.0000, 484.0000, 485.0000, 486.0000, 487.0000, 488.0000, 489.0000, 490.0000, 491.0000, 492.0000, 493.0000, 494.0000, 495.0000, 496.0000, 497.0000, 498.0000, 499.0000, 500.0000, 501.0000, 502.0000, 503.0000, 504.0000, 505.0000, 506.0000, 507.0000, 508.0000, 509.0000, 510.0000, 511.0000, 512.0000, 513.0000, 514.0000, 515.0000, 516.0000, 517.0000, 518.0000, 519.0000, 520.0000, 521.0000, 522.0000, 523.0000, 524.0000, 525.0000, 526.0000, 527.0000, 528.0000, 529.0000, 530.0000, 531.0000, 532.0000, 533.0000, 534.0000, 535.0000, 536.0000, 537.0000, 538.0000, 539.0000, 540.0000, 541.0000, 542.0000, 543.0000, 544.0000, 545.0000, 546.0000, 547.0000, 548.0000, 549.0000, 550.0000, 551.0000, 552.0000, 553.0000, 554.0000, 555.0000, 556.0000, 557.0000, 558.0000, 559.0000, 560.0000, 561.0000, 562.0000, 563.0000, 564.0000, 565.0000, 566.0000, 567.0000, 568.0000, 569.0000, 570.0000, 571.0000, 572.0000, 573.0000, 574.0000, 575.0000, 576.0000, 577.0000, 578.0000, 579.0000, 580.0000, 581.0000, 582.0000, 583.0000, 584.0000, 585.0000, 586.0000, 587.0000, 588.0000, 589.0000, 590.0000, 591.0000, 592.0000, 593.0000, 594.0000, 595.0000, 596.0000, 597.0000, 598.0000, 599.0000, 600.0000, 601.0000, 602.0000, 603.0000, 604.0000, 605.0000, 606.0000, 607.0000, 608.0000, 609.0000, 610.0000, 611.0000, 612.0000, 613.0000, 614.0000, 615.0000, 616.0000, 617.0000, 618.0000, 619.0000, 620.0000, 621.0000, 622.0000, 623.0000, 624.0000, 625.0000, 626.0000, 627.0000, 628.0000, 629.0000, 630.0000, 631.0000, 632.0000, 633.0000, 634.0000, 635.0000, 636.0000, 637.0000, 638.0000, 639.0000, 640.0000, 641.0000, 642.0000, 643.0000, 644.0000, 645.0000, 646.0000, 647.0000, 648.0000, 649.0000, 650.0000, 651.0000, 652.0000, 653.0000, 654.0000, 655.0000, 656.0000, 657.0000, 658.0000, 659.0000, 660.0000, 661.0000, 662.0000, 663.0000, 664.0000, 665.0000, 666.0000, 667.0000, 668.0000, 669.0000, 670.0000, 671.0000, 672.0000, 673.0000, 674.0000, 675.0000, 676.0000, 677.0000, 678.0000, 679.0000, 680.0000, 681.0000, 682.0000, 683.0000, 684.0000, 685.0000, 686.0000, 687.0000, 688.0000, 689.0000, 690.0000, 691.0000, 692.0000, 693.0000, 694.0000, 695.0000, 696.0000, 697.0000, 698.0000, 699.0000, 700.0000, 701.0000, 702.0000, 703.0000, 704.0000, 705.0000, 706.0000, 707.0000, 708.0000, 709.0000, 710.0000, 711.0000, 712.0000, 713.0000, 714.0000, 715.0000, 716.0000, 717.0000, 718.0000, 719.0000, 720.0000, 721.0000, 722.0000, 723.0000, 724.0000, 725.0000, 726.0000, 727.0000, 728.0000, 729.0000, 730.0000, 731.0000, 732.0000, 733.0000, 734.0000, 735.0000, 736.0000, 737.0000, 738.0000, 739.0000, 740.0000, 741.0000, 742.0000, 743.0000, 744.0000, 745.0000, 746.0000, 747.0000, 748.0000, 749.0000, 750.0000, 751.0000, 752.0000, 753.0000, 754.0000, 755.0000, 756.0000, 757.0000, 758.0000, 759.0000, 760.0000, 761.0000, 762.0000, 763.0000, 764.0000, 765.0000, 766.0000, 767.0000, 768.0000, 769.0000, 770.0000, 771.0000, 772.0000, 773.0000, 774.0000, 775.0000, 776.0000, 777.0000, 778.0000, 779.0000, 780.0000, 781.0000, 782.0000, 783.0000, 784.0000, 785.0000, 786.0000, 787.0000, 788.0000, 789.0000, 790.0000, 791.0000, 792.0000, 793.0000, 794.0000, 795.0000, 796.0000, 797.0000, 798.0000, 799.0000, 800.0000, 801.0000, 802.0000, 803.0000, 804.0000, 805.0000, 806.0000, 807.0000, 808.0000, 809.0000, 810.0000, 811.0000, 812.0000, 813.0000, 814.0000, 815.0000, 816.0000, 817.0000, 818.0000, 819.0000, 820.0000, 821.0000, 822.0000, 823.0000, 824.0000, 825.0000, 826.0000, 827.0000, 828.0000, 829.0000, 830.0000, 831.0000, 832.0000, 833.0000, 834.0000, 835.0000, 836.0000, 837.0000, 838.0000, 839.0000, 840.0000, 841.0000, 842.0000, 843.0000, 844.0000, 845.0000, 846.0000, 847.0000, 848.0000, 849.0000, 850.0000, 851.0000, 852.0000, 853.0000, 854.0000, 855.0000, 856.0000, 857.0000, 858.0000, 859.0000, 860.0000, 861.0000, 862.0000, 863.0000, 864.0000, 865.0000, 866.0000, 867.0000, 868.0000, 869.0000, 870.0000, 871.0000, 872.0000, 873.0000, 874.0000, 875.0000, 876.0000, 877.0000, 878.0000, 879.0000, 880.0000, 881.0000, 882.0000, 883.0000, 884.0000, 885.0000, 886.0000, 887.0000, 888.0000, 889.0000, 890.0000, 891.0000, 892.0000, 893.0000, 894.0000, 895.0000, 896.0000, 897.0000, 898.0000, 899.0000, 900.0000, 901.0000, 902.0000, 903.0000, 904.0000, 905.0000, 906.0000, 907.0000, 908.0000, 909.0000, 910.0000, 911.0000, 912.0000, 913.0000, 914.0000, 915.0000, 916.0000, 917.0000, 918.0000, 919.0000, 920.0000, 921.0000, 922.0000, 923.0000, 924.0000, 925.0000, 926.0000, 927.0000, 928.0000, 929.0000, 930.0000, 931.0000, 932.0000, 933.0000, 934.0000, 935.0000, 936.0000, 937.0000, 938.0000, 939.0000, 940.0000, 941.0000, 942.0000, 943.0000, 944.0000, 945.0000, 946.0000, 947.0000, 948.0000, 949.0000, 950.0000, 951.0000, 952.0000, 953.0000, 954.0000, 955.0000, 956.0000, 957.0000, 958.0000, 959.0000, 960.0000, 961.0000, 962.0000, 963.0000, 964.0000, 965.0000, 966.0000, 967.0000, 968.0000, 969.0000, 970.0000, 971.0000, 972.0000, 973.0000, 974.0000, 975.0000, 976.0000, 977.0000, 978.0000, 979.0000, 980.0000, 981.0000, 982.0000, 983.0000, 984.0000, 985.0000, 986.0000, 987.0000, 988.0000, 989.0000, 990.0000, 991.0000, 992.0000, 993.0000, 994.0000, 995.0000, 996.0000, 997.0000, 998.0000, 999.0000, 1000.0000, 144.1605, 145.3497, 136.9685, 138.8707, 140.0008, 144.0931, 144.4691, 133.4957, 140.4420, 134.5886, 145.0861, 149.2614, 147.1828, 138.2964, 135.6931, 150.8272, 133.9296, 149.8886, 146.0225, 140.7515, 146.5819, 142.0839, 148.0280, 148.0718, 143.4488, 139.4022, 146.5183, 153.0853, 149.8297, 142.4327, 140.3442, 145.3004, 138.8774, 141.0171, 138.4806, 139.5692, 148.7675, 139.9857, 132.2366, 143.5523, 150.2440, 143.3354, 145.2843, 141.4253, 141.6074, 146.4546, 150.4195, 152.2170, 147.3088, 139.6372, 153.5393, 139.2209, 141.4803, 146.7837, 149.1144, 145.3190, 151.5831, 138.7570, 142.2569, 132.4972, 150.0875, 146.5132, 136.5848, 143.2504, 151.3242, 140.2918, 148.2455, 140.4234, 145.0395, 149.1613, 144.7252, 146.5588, 140.7318, 139.1762, 140.0207, 144.7510, 150.4555, 149.4119, 151.3080, 152.3981, 145.8648, 155.0549, 145.3681, 149.4766, 138.9280, 136.1319, 141.7320, 144.5705, 149.3660, 137.0893, 144.7434, 135.4874, 147.4043, 137.8618, 142.8675, 140.6655, 144.8382, 143.6388, 135.6380, 141.1142, 144.9045, 150.0321, 144.2085, 144.9672, 144.1530, 146.9005, 148.2904, 139.3573, 137.9601, 138.1551, 142.4474, 146.4297, 136.0679, 145.2214, 150.1037, 137.4920, 149.5394, 132.8482, 157.2533, 142.4810, 141.5875, 142.8538, 140.2444, 146.8262, 143.9927, 138.6104, 142.6813, 135.0644, 146.8078, 147.4567, 147.4310, 144.6144, 143.5622, 148.2437, 138.9880, 144.4311, 144.4640, 145.8720, 147.2501, 146.5579, 139.4897, 147.9092, 150.7890, 153.4445, 141.4227, 149.5868, 138.4539, 141.3090, 153.2005, 147.5418, 144.0085, 142.3875, 150.1269, 153.3112, 150.8279, 144.0745, 140.7557, 147.0194, 143.8676, 142.5700, 147.9859, 146.1104, 136.5740, 145.8285, 149.9152, 150.2720, 143.4598, 144.2991, 141.3553, 145.3776, 146.8437, 141.8552, 142.4968, 144.8215, 148.3262, 141.6215, 143.0252, 145.3412, 149.0047, 143.6262, 152.9933, 148.9750, 143.7080, 144.9935, 151.4143, 134.3002, 138.3659, 150.9197, 141.2596, 146.7580, 154.5644, 135.6178, 145.7327, 139.4482, 146.6293, 146.4257, 152.7130, 145.3789, 140.2605, 141.5921, 145.7652, 150.2608, 157.1304, 142.9835, 144.7761, 141.6577, 138.8156, 140.6591, 154.3256, 143.6617, 147.8182, 146.1997, 146.4656, 144.6060, 138.4727, 139.1312, 142.7931, 147.4064, 136.9734, 144.5627, 147.3372, 141.8361, 141.9689, 148.7811, 149.5072, 147.1037, 142.6615, 143.0288, 139.8254, 150.0514, 147.5411, 144.5335, 145.7701, 144.8952, 131.8721, 138.1535, 150.8127, 145.2257, 141.6708, 145.0689, 139.9509, 135.9761, 141.4737, 145.9197, 149.3153, 147.1316, 146.9406, 148.6616, 149.6192, 139.6094, 138.5608, 131.7133, 148.1645, 145.4104, 150.4570, 137.2578, 136.7173, 143.8992, 150.4660, 145.4640, 149.5899, 150.2581, 135.9370, 142.4135, 154.3303, 141.3589, 138.1023, 137.4413, 141.1914, 150.6253, 146.9932, 145.5888, 146.1491, 142.3825, 141.5944, 147.8606, 143.8797, 140.6348, 140.2458, 136.6641, 144.7953, 136.3012, 152.3043, 144.1258, 147.2522, 150.4562, 146.8852, 137.0053, 136.7177, 133.9933, 136.8204, 142.5150, 146.8835, 154.4812, 139.9777, 146.2664, 146.3222, 145.7898, 146.4855, 147.5112, 143.4380, 147.4288, 147.3063, 140.7205, 152.2725, 143.6769, 146.8264, 155.0823, 138.9369, 141.1097, 151.3155, 138.3562, 131.3679, 142.4981, 150.3340, 140.6665, 143.2998, 149.2673, 149.8251, 145.6702, 135.3978, 132.1892, 143.6815, 143.5986, 149.3590, 149.6898, 145.2403, 141.8077, 141.6322, 143.9340, 137.0202, 144.3808, 144.7312, 150.1267, 148.1176, 139.0454, 146.8704, 147.5984, 147.4757, 141.0859, 146.5466, 150.0808, 146.9589, 149.2464, 149.1681, 146.7617, 138.2907, 130.6583, 138.1683, 145.6311, 146.9842, 138.1591, 152.1584, 148.0685, 144.5813, 145.3592, 139.4933, 148.3363, 148.1046, 142.1957, 146.7638, 150.8264, 145.2372, 144.0290, 134.3971, 143.1404, 145.9345, 144.6111, 144.0244, 143.4652, 145.8886, 148.2257, 147.0097, 138.4404, 141.1900, 133.9871, 146.5930, 138.6878, 149.0977, 140.7470, 145.1073, 144.8257, 138.1044, 148.2437, 148.2365, 142.4616, 147.3717, 141.2359, 150.1797, 149.8094, 138.6433, 142.9742, 138.6652, 140.9880, 140.7694, 139.4357, 148.2649, 143.2074, 138.9088, 149.9937, 145.7740, 151.8219, 143.3164, 144.9093, 141.9169, 149.0048, 136.7802, 150.9028, 139.4421, 140.6361, 147.2201, 147.8388, 154.0625, 152.1600, 144.0335, 148.4152, 138.3404, 155.0781, 143.0277, 140.3660, 141.9668, 146.8281, 147.8702, 143.8193, 140.9553, 159.1761, 151.8703, 138.0026, 135.1808, 147.5798, 137.2241, 148.8593, 139.7089, 144.9603, 146.0191, 144.7222, 141.0733, 139.9456, 134.6781, 151.3330, 147.5724, 141.5872, 138.2842, 144.9639, 153.1910, 137.3685, 141.4960, 145.7170, 146.5621, 138.5851, 141.4137, 140.2881, 142.6874, 145.3476, 146.7836, 131.4512, 145.4347, 146.4179, 144.9085, 134.1970, 140.4508, 145.2417, 143.5063, 151.0992, 137.1601, 153.1905, 152.9020, 154.3585, 140.5628, 144.5083, 141.0228, 146.4540, 150.7697, 134.6000, 145.8255, 141.7447, 143.0526, 143.5525, 147.8934, 143.6224, 143.6509, 150.2553, 137.1523, 145.9260, 141.6611, 149.0161, 150.3929, 149.9475, 149.2405, 139.2055, 145.4273, 146.4187, 147.4818, 147.0737, 145.7187, 141.4912, 144.9070, 145.9867, 135.8008, 141.3210, 138.1932, 142.7958, 144.8795, 145.2725, 144.2394, 140.9581, 150.0769, 140.1889, 139.3500, 151.4588, 131.8665, 138.8556, 147.6361, 139.7307, 143.9298, 157.6787, 145.4696, 141.0539, 137.6614, 145.7937, 143.1795, 151.6034, 149.4277, 142.1234, 151.0579, 152.2909, 147.9255, 145.5291, 138.0506, 148.6785, 150.9105, 154.8300, 149.9494, 144.6962, 148.6885, 147.0193, 148.6291, 136.4126, 139.6123, 150.6926, 143.9535, 144.5039, 144.0535, 139.1708, 140.9033, 150.2118, 143.9461, 143.8687, 143.8920, 139.1750, 142.2332, 140.9148, 150.3608, 137.9963, 144.0070, 143.6017, 138.9211, 151.8292, 152.2761, 147.6486, 144.4502, 146.1277, 135.8991, 139.0093, 141.0730, 136.0800, 152.9988, 143.0179, 145.3264, 149.7162, 156.7217, 143.3865, 143.7138, 149.4421, 137.2188, 137.5762, 138.9973, 143.8838, 147.3699, 146.5711, 146.6845, 141.5647, 142.3636, 141.5825, 131.5206, 138.0924, 138.9434, 138.7485, 138.8584, 147.9345, 152.6326, 147.7998, 151.1001, 139.5305, 145.7336, 147.3221, 142.1454, 140.9811, 149.3825, 145.2611, 142.0813, 138.8578, 151.1061, 142.0702, 160.5191, 140.5724, 134.4248, 140.7332, 138.1706, 151.3062, 153.2640, 143.0488, 143.8836, 147.5089, 143.4207, 145.8425, 156.9944, 149.1779, 136.7475, 151.0164, 152.5901, 139.8459, 141.5842, 150.5296, 138.2857, 156.1986, 149.5156, 146.7707, 136.2990, 146.3348, 140.5468, 144.9553, 149.5479, 151.1190, 147.4055, 139.1635, 149.7826, 148.6060, 137.4868, 150.4521, 140.8444, 145.4902, 142.1661, 140.9589, 151.0550, 135.8103, 148.1340, 146.0845, 148.0428, 135.7707, 138.8273, 145.7282, 142.0212, 135.8101, 143.1760, 143.4555, 141.7821, 136.5051, 132.1813, 150.0956, 148.5958, 155.2769, 144.5337, 137.2234, 143.5074, 149.2869, 141.7430, 140.9280, 146.8030, 134.8756, 141.2867, 142.0018, 139.4345, 138.5363, 148.2095, 133.7640, 139.3770, 135.8077, 144.0182, 146.1947, 141.6147, 149.5593, 145.5881, 152.1942, 136.1212, 144.6240, 138.6689, 141.5164, 138.1615, 145.2184, 138.7886, 140.8627, 148.8331, 146.1623, 146.9151, 161.7034, 153.2175, 145.8589, 144.5738, 136.7792, 147.5382, 149.1994, 139.3532, 147.5039, 144.9310, 150.9541, 143.1802, 147.0922, 147.5758, 159.0819, 146.2873, 146.7259, 147.9818, 136.3334, 137.3764, 147.3818, 143.5956, 147.2540, 143.2949, 139.7994, 142.5328, 144.4284, 144.0513, 140.3482, 143.4965, 145.6454, 137.6958, 152.6932, 145.3406, 138.7954, 144.0105, 141.3499, 145.3331, 150.1384, 139.3216, 149.9099, 149.3700, 141.0016, 148.7082, 141.4208, 145.4290, 157.0516, 139.8969, 142.9752, 143.4804, 147.4091, 141.3333, 145.8656, 143.9680, 142.6859, 139.7143, 146.5336, 145.1292, 143.3378, 147.0863, 146.8685, 143.1406, 143.0813, 145.3302, 146.2704, 148.2215, 158.7684, 141.7948, 140.8641, 141.9482, 138.7998, 143.5074, 136.6432, 143.1943, 139.9216, 142.6725, 143.1983, 148.9951, 144.5245, 147.9711, 152.1542, 148.6412, 140.4758, 141.3435, 137.4598, 144.1747, 141.6185, 135.6476, 132.3867, 151.4901, 145.1499, 141.7691, 139.1892, 136.4018, 146.6063, 134.2928, 147.9550, 143.7982, 143.3536, 149.7203, 148.5049, 140.8338, 145.0869, 149.7860, 141.1814, 152.9165, 137.4459, 150.9661, 142.8716, 150.1063, 147.2050, 147.7471, 141.9136, 144.3295, 150.5548, 141.6486, 146.9076, 150.1862, 146.6875, 142.4206, 144.9510, 144.6912, 144.8818, 138.8385, 137.4153, 149.7343, 149.5319, 142.8561, 142.0459, 140.0731, 142.1667, 137.1278, 152.6024, 144.4476, 144.1001, 142.0906, 141.8189, 147.9072, 148.5266, 143.7783, 142.3145, 146.7125, 136.4232, 149.4627, 138.9318, 138.4734, 139.2000, 140.6889, 145.9588, 143.6183, 144.2394, 136.9475, 137.5518, 142.3224, 149.3829, 136.8370, 146.6371, 143.9195, 146.0778, 142.4099, 151.1147, 144.7342, 152.4495, 142.3724, 145.4357, 143.9911, 145.4499, 140.3718, 142.6686, 146.3206, 136.5425, 143.8628, 137.9681, 147.4589, 140.1305, 145.7857, 148.0142, 145.8855, 145.8485, 137.4962, 147.6354, 138.6232, 143.8813, 137.4832, 143.0394, 142.3019, 148.9393, 145.7816, 147.5698, 137.1655, 142.5818, 144.4689, 137.4145, 154.5748, 151.5958, 137.6229, 143.1235, 151.5293, 142.3392, 144.9301, 141.6498, 147.3258, 153.4111, 148.2462, 140.9139, 144.1693, 151.1516, 139.3584, 135.5631, 142.2479, 146.7326, 139.6869, 147.2478, 145.5323, 146.3532, 143.6411, 154.8393, 146.7776, 144.0927, 137.3802, 134.1089, 147.3096, 142.0465, 139.0278, 145.5329, 151.9646, 148.2254, 140.5743, 146.5136, 146.4283, 147.7145, 144.3475, 139.0477, 138.9367, 138.0313, 142.3662, 138.3616, 149.0659, 135.4847, 140.4779, 136.1042, 144.0094, 143.6612, 135.0866, 145.1589, 132.6690, 142.3830, 149.9593, 143.9152, 153.6336, 143.0619, 146.6714, 151.0250, 136.5274, 148.7079, 144.5604, 147.1778, 147.8885, 144.5316, 139.6159, 141.0052, 148.7410, 146.9785, 142.8103, 147.1194, 141.8948, 147.6827, 149.6170, 151.2936, 142.0534, 145.2935, 143.1936, 148.2821, 142.4875, 143.5971, 144.2556, 151.1568, 148.9291, 151.6551, 147.9372, 141.5767, 144.7778, 141.0926, 142.1244, 135.9091, 146.2655, 143.1831, 143.4843, 142.4299, 150.9044, 140.3706, 142.3026, 147.6209, 138.9361, 141.0249, 136.7004, 138.3260, 146.8230, 146.1246, 145.6898, 146.2869, 138.9337, 136.4935, 143.8706, 150.0415
## 5 1.0000, 2.0000, 3.0000, 4.0000, 5.0000, 6.0000, 7.0000, 8.0000, 9.0000, 10.0000, 11.0000, 12.0000, 13.0000, 14.0000, 15.0000, 16.0000, 17.0000, 18.0000, 19.0000, 20.0000, 21.0000, 22.0000, 23.0000, 24.0000, 25.0000, 26.0000, 27.0000, 28.0000, 29.0000, 30.0000, 31.0000, 32.0000, 33.0000, 34.0000, 35.0000, 36.0000, 37.0000, 38.0000, 39.0000, 40.0000, 41.0000, 42.0000, 43.0000, 44.0000, 45.0000, 46.0000, 47.0000, 48.0000, 49.0000, 50.0000, 51.0000, 52.0000, 53.0000, 54.0000, 55.0000, 56.0000, 57.0000, 58.0000, 59.0000, 60.0000, 61.0000, 62.0000, 63.0000, 64.0000, 65.0000, 66.0000, 67.0000, 68.0000, 69.0000, 70.0000, 71.0000, 72.0000, 73.0000, 74.0000, 75.0000, 76.0000, 77.0000, 78.0000, 79.0000, 80.0000, 81.0000, 82.0000, 83.0000, 84.0000, 85.0000, 86.0000, 87.0000, 88.0000, 89.0000, 90.0000, 91.0000, 92.0000, 93.0000, 94.0000, 95.0000, 96.0000, 97.0000, 98.0000, 99.0000, 100.0000, 101.0000, 102.0000, 103.0000, 104.0000, 105.0000, 106.0000, 107.0000, 108.0000, 109.0000, 110.0000, 111.0000, 112.0000, 113.0000, 114.0000, 115.0000, 116.0000, 117.0000, 118.0000, 119.0000, 120.0000, 121.0000, 122.0000, 123.0000, 124.0000, 125.0000, 126.0000, 127.0000, 128.0000, 129.0000, 130.0000, 131.0000, 132.0000, 133.0000, 134.0000, 135.0000, 136.0000, 137.0000, 138.0000, 139.0000, 140.0000, 141.0000, 142.0000, 143.0000, 144.0000, 145.0000, 146.0000, 147.0000, 148.0000, 149.0000, 150.0000, 151.0000, 152.0000, 153.0000, 154.0000, 155.0000, 156.0000, 157.0000, 158.0000, 159.0000, 160.0000, 161.0000, 162.0000, 163.0000, 164.0000, 165.0000, 166.0000, 167.0000, 168.0000, 169.0000, 170.0000, 171.0000, 172.0000, 173.0000, 174.0000, 175.0000, 176.0000, 177.0000, 178.0000, 179.0000, 180.0000, 181.0000, 182.0000, 183.0000, 184.0000, 185.0000, 186.0000, 187.0000, 188.0000, 189.0000, 190.0000, 191.0000, 192.0000, 193.0000, 194.0000, 195.0000, 196.0000, 197.0000, 198.0000, 199.0000, 200.0000, 201.0000, 202.0000, 203.0000, 204.0000, 205.0000, 206.0000, 207.0000, 208.0000, 209.0000, 210.0000, 211.0000, 212.0000, 213.0000, 214.0000, 215.0000, 216.0000, 217.0000, 218.0000, 219.0000, 220.0000, 221.0000, 222.0000, 223.0000, 224.0000, 225.0000, 226.0000, 227.0000, 228.0000, 229.0000, 230.0000, 231.0000, 232.0000, 233.0000, 234.0000, 235.0000, 236.0000, 237.0000, 238.0000, 239.0000, 240.0000, 241.0000, 242.0000, 243.0000, 244.0000, 245.0000, 246.0000, 247.0000, 248.0000, 249.0000, 250.0000, 251.0000, 252.0000, 253.0000, 254.0000, 255.0000, 256.0000, 257.0000, 258.0000, 259.0000, 260.0000, 261.0000, 262.0000, 263.0000, 264.0000, 265.0000, 266.0000, 267.0000, 268.0000, 269.0000, 270.0000, 271.0000, 272.0000, 273.0000, 274.0000, 275.0000, 276.0000, 277.0000, 278.0000, 279.0000, 280.0000, 281.0000, 282.0000, 283.0000, 284.0000, 285.0000, 286.0000, 287.0000, 288.0000, 289.0000, 290.0000, 291.0000, 292.0000, 293.0000, 294.0000, 295.0000, 296.0000, 297.0000, 298.0000, 299.0000, 300.0000, 301.0000, 302.0000, 303.0000, 304.0000, 305.0000, 306.0000, 307.0000, 308.0000, 309.0000, 310.0000, 311.0000, 312.0000, 313.0000, 314.0000, 315.0000, 316.0000, 317.0000, 318.0000, 319.0000, 320.0000, 321.0000, 322.0000, 323.0000, 324.0000, 325.0000, 326.0000, 327.0000, 328.0000, 329.0000, 330.0000, 331.0000, 332.0000, 333.0000, 334.0000, 335.0000, 336.0000, 337.0000, 338.0000, 339.0000, 340.0000, 341.0000, 342.0000, 343.0000, 344.0000, 345.0000, 346.0000, 347.0000, 348.0000, 349.0000, 350.0000, 351.0000, 352.0000, 353.0000, 354.0000, 355.0000, 356.0000, 357.0000, 358.0000, 359.0000, 360.0000, 361.0000, 362.0000, 363.0000, 364.0000, 365.0000, 366.0000, 367.0000, 368.0000, 369.0000, 370.0000, 371.0000, 372.0000, 373.0000, 374.0000, 375.0000, 376.0000, 377.0000, 378.0000, 379.0000, 380.0000, 381.0000, 382.0000, 383.0000, 384.0000, 385.0000, 386.0000, 387.0000, 388.0000, 389.0000, 390.0000, 391.0000, 392.0000, 393.0000, 394.0000, 395.0000, 396.0000, 397.0000, 398.0000, 399.0000, 400.0000, 401.0000, 402.0000, 403.0000, 404.0000, 405.0000, 406.0000, 407.0000, 408.0000, 409.0000, 410.0000, 411.0000, 412.0000, 413.0000, 414.0000, 415.0000, 416.0000, 417.0000, 418.0000, 419.0000, 420.0000, 421.0000, 422.0000, 423.0000, 424.0000, 425.0000, 426.0000, 427.0000, 428.0000, 429.0000, 430.0000, 431.0000, 432.0000, 433.0000, 434.0000, 435.0000, 436.0000, 437.0000, 438.0000, 439.0000, 440.0000, 441.0000, 442.0000, 443.0000, 444.0000, 445.0000, 446.0000, 447.0000, 448.0000, 449.0000, 450.0000, 451.0000, 452.0000, 453.0000, 454.0000, 455.0000, 456.0000, 457.0000, 458.0000, 459.0000, 460.0000, 461.0000, 462.0000, 463.0000, 464.0000, 465.0000, 466.0000, 467.0000, 468.0000, 469.0000, 470.0000, 471.0000, 472.0000, 473.0000, 474.0000, 475.0000, 476.0000, 477.0000, 478.0000, 479.0000, 480.0000, 481.0000, 482.0000, 483.0000, 484.0000, 485.0000, 486.0000, 487.0000, 488.0000, 489.0000, 490.0000, 491.0000, 492.0000, 493.0000, 494.0000, 495.0000, 496.0000, 497.0000, 498.0000, 499.0000, 500.0000, 501.0000, 502.0000, 503.0000, 504.0000, 505.0000, 506.0000, 507.0000, 508.0000, 509.0000, 510.0000, 511.0000, 512.0000, 513.0000, 514.0000, 515.0000, 516.0000, 517.0000, 518.0000, 519.0000, 520.0000, 521.0000, 522.0000, 523.0000, 524.0000, 525.0000, 526.0000, 527.0000, 528.0000, 529.0000, 530.0000, 531.0000, 532.0000, 533.0000, 534.0000, 535.0000, 536.0000, 537.0000, 538.0000, 539.0000, 540.0000, 541.0000, 542.0000, 543.0000, 544.0000, 545.0000, 546.0000, 547.0000, 548.0000, 549.0000, 550.0000, 551.0000, 552.0000, 553.0000, 554.0000, 555.0000, 556.0000, 557.0000, 558.0000, 559.0000, 560.0000, 561.0000, 562.0000, 563.0000, 564.0000, 565.0000, 566.0000, 567.0000, 568.0000, 569.0000, 570.0000, 571.0000, 572.0000, 573.0000, 574.0000, 575.0000, 576.0000, 577.0000, 578.0000, 579.0000, 580.0000, 581.0000, 582.0000, 583.0000, 584.0000, 585.0000, 586.0000, 587.0000, 588.0000, 589.0000, 590.0000, 591.0000, 592.0000, 593.0000, 594.0000, 595.0000, 596.0000, 597.0000, 598.0000, 599.0000, 600.0000, 601.0000, 602.0000, 603.0000, 604.0000, 605.0000, 606.0000, 607.0000, 608.0000, 609.0000, 610.0000, 611.0000, 612.0000, 613.0000, 614.0000, 615.0000, 616.0000, 617.0000, 618.0000, 619.0000, 620.0000, 621.0000, 622.0000, 623.0000, 624.0000, 625.0000, 626.0000, 627.0000, 628.0000, 629.0000, 630.0000, 631.0000, 632.0000, 633.0000, 634.0000, 635.0000, 636.0000, 637.0000, 638.0000, 639.0000, 640.0000, 641.0000, 642.0000, 643.0000, 644.0000, 645.0000, 646.0000, 647.0000, 648.0000, 649.0000, 650.0000, 651.0000, 652.0000, 653.0000, 654.0000, 655.0000, 656.0000, 657.0000, 658.0000, 659.0000, 660.0000, 661.0000, 662.0000, 663.0000, 664.0000, 665.0000, 666.0000, 667.0000, 668.0000, 669.0000, 670.0000, 671.0000, 672.0000, 673.0000, 674.0000, 675.0000, 676.0000, 677.0000, 678.0000, 679.0000, 680.0000, 681.0000, 682.0000, 683.0000, 684.0000, 685.0000, 686.0000, 687.0000, 688.0000, 689.0000, 690.0000, 691.0000, 692.0000, 693.0000, 694.0000, 695.0000, 696.0000, 697.0000, 698.0000, 699.0000, 700.0000, 701.0000, 702.0000, 703.0000, 704.0000, 705.0000, 706.0000, 707.0000, 708.0000, 709.0000, 710.0000, 711.0000, 712.0000, 713.0000, 714.0000, 715.0000, 716.0000, 717.0000, 718.0000, 719.0000, 720.0000, 721.0000, 722.0000, 723.0000, 724.0000, 725.0000, 726.0000, 727.0000, 728.0000, 729.0000, 730.0000, 731.0000, 732.0000, 733.0000, 734.0000, 735.0000, 736.0000, 737.0000, 738.0000, 739.0000, 740.0000, 741.0000, 742.0000, 743.0000, 744.0000, 745.0000, 746.0000, 747.0000, 748.0000, 749.0000, 750.0000, 751.0000, 752.0000, 753.0000, 754.0000, 755.0000, 756.0000, 757.0000, 758.0000, 759.0000, 760.0000, 761.0000, 762.0000, 763.0000, 764.0000, 765.0000, 766.0000, 767.0000, 768.0000, 769.0000, 770.0000, 771.0000, 772.0000, 773.0000, 774.0000, 775.0000, 776.0000, 777.0000, 778.0000, 779.0000, 780.0000, 781.0000, 782.0000, 783.0000, 784.0000, 785.0000, 786.0000, 787.0000, 788.0000, 789.0000, 790.0000, 791.0000, 792.0000, 793.0000, 794.0000, 795.0000, 796.0000, 797.0000, 798.0000, 799.0000, 800.0000, 801.0000, 802.0000, 803.0000, 804.0000, 805.0000, 806.0000, 807.0000, 808.0000, 809.0000, 810.0000, 811.0000, 812.0000, 813.0000, 814.0000, 815.0000, 816.0000, 817.0000, 818.0000, 819.0000, 820.0000, 821.0000, 822.0000, 823.0000, 824.0000, 825.0000, 826.0000, 827.0000, 828.0000, 829.0000, 830.0000, 831.0000, 832.0000, 833.0000, 834.0000, 835.0000, 836.0000, 837.0000, 838.0000, 839.0000, 840.0000, 841.0000, 842.0000, 843.0000, 844.0000, 845.0000, 846.0000, 847.0000, 848.0000, 849.0000, 850.0000, 851.0000, 852.0000, 853.0000, 854.0000, 855.0000, 856.0000, 857.0000, 858.0000, 859.0000, 860.0000, 861.0000, 862.0000, 863.0000, 864.0000, 865.0000, 866.0000, 867.0000, 868.0000, 869.0000, 870.0000, 871.0000, 872.0000, 873.0000, 874.0000, 875.0000, 876.0000, 877.0000, 878.0000, 879.0000, 880.0000, 881.0000, 882.0000, 883.0000, 884.0000, 885.0000, 886.0000, 887.0000, 888.0000, 889.0000, 890.0000, 891.0000, 892.0000, 893.0000, 894.0000, 895.0000, 896.0000, 897.0000, 898.0000, 899.0000, 900.0000, 901.0000, 902.0000, 903.0000, 904.0000, 905.0000, 906.0000, 907.0000, 908.0000, 909.0000, 910.0000, 911.0000, 912.0000, 913.0000, 914.0000, 915.0000, 916.0000, 917.0000, 918.0000, 919.0000, 920.0000, 921.0000, 922.0000, 923.0000, 924.0000, 925.0000, 926.0000, 927.0000, 928.0000, 929.0000, 930.0000, 931.0000, 932.0000, 933.0000, 934.0000, 935.0000, 936.0000, 937.0000, 938.0000, 939.0000, 940.0000, 941.0000, 942.0000, 943.0000, 944.0000, 945.0000, 946.0000, 947.0000, 948.0000, 949.0000, 950.0000, 951.0000, 952.0000, 953.0000, 954.0000, 955.0000, 956.0000, 957.0000, 958.0000, 959.0000, 960.0000, 961.0000, 962.0000, 963.0000, 964.0000, 965.0000, 966.0000, 967.0000, 968.0000, 969.0000, 970.0000, 971.0000, 972.0000, 973.0000, 974.0000, 975.0000, 976.0000, 977.0000, 978.0000, 979.0000, 980.0000, 981.0000, 982.0000, 983.0000, 984.0000, 985.0000, 986.0000, 987.0000, 988.0000, 989.0000, 990.0000, 991.0000, 992.0000, 993.0000, 994.0000, 995.0000, 996.0000, 997.0000, 998.0000, 999.0000, 1000.0000, 145.6119, 144.9395, 142.1851, 133.4641, 137.4703, 145.1005, 139.1953, 140.2394, 140.2065, 144.9722, 134.7437, 154.9748, 150.4971, 140.8906, 149.6605, 132.8101, 153.5184, 138.5001, 140.6790, 151.0246, 151.8826, 147.1968, 143.8507, 134.6386, 138.5922, 142.7436, 147.2464, 139.8678, 146.2208, 143.6885, 144.5248, 147.6997, 132.1663, 152.2610, 146.0864, 141.8924, 143.7893, 140.2794, 145.5730, 140.3839, 142.8388, 143.3843, 147.6986, 149.8571, 144.9104, 148.6783, 144.3867, 150.2574, 146.8190, 142.9328, 147.0247, 149.9490, 140.5315, 143.1586, 147.2024, 133.9402, 147.2147, 149.9559, 138.9318, 141.8383, 151.3940, 138.5072, 140.5604, 149.1376, 145.9631, 142.7141, 143.4013, 149.2690, 143.5086, 145.2375, 142.9488, 146.5604, 142.2023, 145.1502, 147.1494, 139.1645, 147.6864, 136.1037, 143.1233, 145.9543, 143.1949, 144.6992, 146.3100, 144.1639, 140.9069, 152.8150, 139.7037, 139.8197, 150.4144, 155.6366, 143.1326, 140.1055, 149.2525, 145.7482, 147.7016, 145.1162, 146.5435, 152.5096, 145.6806, 155.3735, 143.0307, 142.1822, 151.5748, 144.8164, 146.1060, 144.2942, 147.9032, 149.9424, 133.1716, 152.4261, 137.9749, 148.2926, 133.0540, 141.4594, 143.2866, 146.4090, 148.5374, 151.2152, 142.3842, 146.9673, 141.4021, 142.3456, 153.7358, 145.7509, 146.6478, 141.2505, 142.6367, 145.1523, 146.9335, 138.6944, 147.2833, 137.9234, 143.7969, 139.3438, 152.8966, 141.0998, 148.6954, 152.0372, 142.5377, 151.0778, 152.4332, 149.3622, 148.6411, 148.4740, 143.0121, 144.5320, 142.9443, 145.0159, 146.7339, 141.8911, 138.5668, 141.0901, 152.4847, 150.5968, 138.5749, 137.7916, 154.2379, 140.5395, 135.9669, 140.8801, 139.7214, 142.3286, 142.5408, 144.0504, 146.6650, 145.2409, 149.4131, 144.6757, 140.8125, 149.9509, 143.6321, 145.7122, 146.1186, 144.5502, 145.4977, 142.8377, 146.4716, 136.6420, 145.4845, 148.6328, 149.8152, 142.9350, 137.9272, 134.5556, 145.2833, 143.2432, 156.5558, 137.7958, 147.8269, 139.0007, 146.8843, 140.7719, 148.5454, 140.8835, 150.6853, 142.4375, 141.9186, 150.3201, 136.6705, 139.3631, 143.2033, 153.7653, 144.0847, 144.7161, 145.3958, 143.2052, 145.0040, 150.9626, 148.5891, 148.5655, 150.2727, 142.8801, 142.0804, 151.3144, 147.5222, 135.9301, 143.8992, 141.7819, 147.5221, 148.1976, 143.0151, 143.6944, 143.5003, 140.7479, 143.1291, 157.0611, 141.3543, 143.9779, 145.8881, 145.5474, 144.9101, 137.5401, 145.1979, 140.7838, 140.5763, 132.3170, 147.2739, 149.3564, 144.4402, 140.9803, 151.3400, 148.2560, 139.5768, 142.1447, 149.8857, 146.1171, 145.8144, 146.8126, 140.5615, 145.2983, 146.3395, 137.8633, 149.7981, 141.6282, 141.3740, 145.6963, 141.7679, 141.6399, 142.2427, 141.0389, 149.3895, 145.7707, 138.7031, 135.3485, 149.6055, 144.0455, 137.6966, 137.0299, 152.2023, 145.3406, 137.9854, 145.2137, 143.4630, 134.8795, 146.7326, 141.2997, 139.3085, 147.9005, 150.7411, 140.5068, 140.0010, 148.7029, 147.9892, 145.1724, 148.5726, 148.6716, 141.1016, 141.6664, 144.6643, 141.3096, 148.0550, 152.4832, 145.3361, 138.0727, 149.0429, 147.8081, 144.5709, 154.3344, 139.7631, 147.0049, 140.5573, 150.2685, 143.9093, 148.7678, 146.3912, 151.6957, 142.5154, 146.2083, 140.3748, 141.0319, 137.8234, 147.5345, 138.4121, 146.3020, 140.7781, 141.7910, 149.2652, 138.0237, 142.7970, 138.9286, 141.5484, 142.8451, 139.6733, 145.0155, 143.9900, 149.9463, 146.7140, 146.9553, 144.6921, 143.0223, 155.0649, 138.4167, 143.1021, 138.3245, 143.8076, 134.0825, 155.3807, 148.5543, 143.3839, 143.5508, 147.7089, 148.2886, 140.2595, 142.1711, 145.1834, 136.5589, 143.6261, 150.0859, 141.2865, 143.6114, 144.1370, 145.9334, 133.5288, 133.8359, 143.9283, 150.6575, 143.4340, 137.6929, 134.4471, 137.6156, 153.9814, 141.2830, 136.2066, 137.0578, 139.3747, 140.7553, 143.7082, 140.4905, 144.8173, 143.2517, 141.1756, 149.4632, 136.9194, 145.5654, 153.5490, 147.9859, 145.7637, 138.3868, 140.1516, 139.8925, 139.3922, 153.5913, 144.5359, 136.8129, 143.9024, 146.0657, 153.1140, 150.5622, 145.2638, 141.6020, 149.0660, 140.3416, 143.0174, 145.7279, 147.3703, 138.0554, 136.2904, 137.3157, 139.8558, 143.9714, 136.2080, 140.4864, 148.4842, 149.4405, 136.8745, 150.4836, 157.2714, 151.6928, 144.8744, 136.2495, 142.0616, 137.9408, 132.8173, 138.1478, 139.6113, 143.5116, 159.1181, 143.9584, 150.1648, 136.7131, 152.1011, 142.3512, 145.7407, 152.3961, 142.4439, 147.9178, 155.4065, 144.2055, 143.1902, 149.9085, 146.1201, 146.4721, 136.9038, 141.3727, 142.8828, 147.9834, 128.9822, 141.1280, 142.2256, 146.5665, 145.2954, 139.8331, 144.7038, 146.6374, 144.6865, 147.9156, 137.6988, 135.1344, 140.8211, 147.1307, 144.6508, 140.0763, 140.7746, 138.6970, 148.0288, 141.0138, 148.2400, 145.4038, 144.3602, 140.9263, 138.9376, 152.1966, 137.2878, 145.4413, 148.2005, 151.3047, 149.7847, 148.0084, 144.8060, 152.9644, 139.9168, 148.7227, 146.1489, 141.6226, 136.4254, 141.0470, 151.2491, 147.7128, 143.8047, 144.1598, 139.2620, 144.8451, 144.4832, 141.3758, 141.8180, 145.7853, 136.2469, 147.8856, 141.6082, 157.2894, 143.2338, 148.7633, 154.4528, 137.1266, 144.8018, 140.2969, 141.0043, 150.7668, 156.2008, 143.9924, 148.9743, 147.9765, 156.1083, 140.0926, 141.3648, 142.1696, 140.1605, 143.5346, 143.3733, 151.7984, 149.5223, 137.4285, 145.6576, 142.6187, 143.8176, 143.0916, 146.6427, 144.6108, 149.8127, 148.2679, 140.2866, 145.1467, 141.7790, 152.4573, 146.0940, 151.6154, 146.8323, 144.2619, 144.3758, 141.6674, 157.9332, 147.7328, 148.6152, 148.6626, 143.6432, 140.2338, 140.4516, 138.4321, 137.4642, 151.1829, 141.8337, 150.5951, 142.7373, 141.8532, 152.9708, 142.7397, 141.9993, 146.5806, 149.0032, 142.2886, 145.8178, 160.5331, 134.4893, 139.2448, 142.0511, 150.4418, 142.5945, 151.6779, 144.7546, 144.5318, 144.9335, 143.9991, 144.2940, 140.6117, 143.7397, 146.4981, 136.5280, 148.3898, 148.5643, 153.4075, 144.0107, 142.4786, 147.5882, 151.0643, 136.2918, 148.0957, 137.1083, 145.9365, 153.6274, 139.4515, 148.5536, 143.1576, 142.6828, 135.7058, 142.8433, 147.6102, 144.9495, 141.9430, 152.5183, 149.4132, 149.0797, 147.1820, 150.9617, 148.2465, 144.9510, 148.9675, 142.6171, 141.8128, 137.1101, 145.0364, 145.8676, 146.9610, 143.9281, 140.4782, 146.1823, 140.3544, 143.2010, 140.2904, 136.8739, 158.1951, 131.4571, 151.9013, 144.4522, 148.0096, 145.8667, 140.6762, 149.9747, 140.1496, 146.4693, 148.4943, 153.2251, 143.4773, 148.4678, 135.7209, 142.3530, 148.2231, 151.7475, 147.0432, 139.3079, 138.9019, 145.5656, 135.0061, 143.9464, 143.7497, 149.0088, 148.2629, 139.1222, 144.4648, 139.3363, 143.2557, 155.7688, 133.9422, 144.4921, 142.5824, 144.2991, 142.5143, 138.5227, 144.6018, 145.4101, 145.1938, 144.7671, 144.2766, 145.9879, 147.2395, 146.1370, 148.3231, 140.1955, 147.1550, 144.3690, 153.3790, 136.2028, 149.4959, 143.9821, 144.8863, 145.3914, 142.1586, 155.1437, 133.3853, 143.9700, 135.6996, 134.4028, 143.9264, 145.8866, 147.4277, 135.2386, 148.9400, 140.7535, 143.8071, 155.9486, 148.9633, 147.1435, 138.6947, 143.6669, 145.6621, 141.6980, 134.9695, 143.9996, 131.9076, 137.7716, 146.3015, 137.6212, 141.9910, 140.7595, 150.2191, 147.7163, 147.7802, 150.8385, 137.1627, 147.3844, 139.4744, 144.1397, 142.4859, 149.8714, 147.2468, 143.5863, 143.2326, 147.4510, 145.6931, 147.2313, 144.0525, 141.5451, 145.9241, 141.5505, 156.5476, 138.1328, 141.3398, 145.9457, 147.1216, 136.2736, 154.6992, 146.1985, 152.2287, 152.6266, 146.2809, 155.1500, 150.8819, 147.0339, 146.4279, 145.6806, 141.7843, 140.4645, 146.0889, 143.2553, 137.1469, 147.8267, 141.8854, 137.7224, 142.8097, 145.8999, 160.0260, 144.1215, 141.5899, 153.0175, 148.0699, 134.4180, 150.5931, 152.0804, 137.2721, 143.1017, 145.0173, 150.5579, 139.5182, 137.0039, 146.1295, 140.9223, 151.9157, 144.0998, 150.4054, 148.6187, 140.2388, 137.2264, 146.0144, 143.4566, 145.9998, 145.1664, 141.3975, 147.0259, 137.5685, 144.2164, 134.4938, 145.4209, 142.9201, 145.1855, 141.1323, 145.2182, 138.9038, 149.1050, 137.4869, 146.0806, 137.6924, 143.0460, 143.2436, 143.6375, 135.7017, 141.6568, 139.6158, 139.2272, 139.9661, 143.0590, 134.0068, 151.4766, 140.1076, 150.6705, 138.6853, 145.4876, 138.2903, 145.2889, 139.7565, 134.9828, 149.9669, 149.5032, 143.7263, 145.8297, 139.9762, 145.0619, 135.8167, 144.7879, 151.2991, 144.9567, 150.0830, 155.5264, 137.0560, 140.4468, 138.6053, 131.0597, 151.0413, 146.6934, 149.9844, 147.1234, 135.0175, 140.2673, 146.6754, 144.8293, 142.8734, 143.0298, 148.4315, 139.8222, 139.1012, 139.8409, 148.2781, 133.5384, 138.8853, 155.6414, 149.3356, 143.8157, 144.0054, 147.4720, 137.6367, 152.4696, 145.5161, 143.8628, 129.7298, 146.4355, 141.4181, 151.3526, 151.6539, 137.5845, 143.5511, 144.6584, 139.1557, 149.5035, 142.6487, 157.9294, 147.4950, 148.0740, 143.1050, 143.7796, 136.7219, 145.6779, 152.2756, 154.9216, 144.1325, 151.8633, 149.7668, 145.9953, 138.1724, 152.3820, 147.5445, 142.9589, 145.7268, 149.6729, 153.3093, 144.4462, 137.2276, 144.8895, 140.4257, 144.1434, 139.6689, 142.0318, 147.5048, 149.8704, 150.3923, 143.0633, 148.4212, 147.8179, 144.4008, 147.5553, 141.8767, 146.2616, 155.0251, 145.0477, 146.9375, 150.3778, 138.9422, 140.5046, 150.0075, 141.7543, 137.6561, 146.8319, 149.2653, 144.5749, 146.8433, 138.9934, 145.1851, 146.1305, 145.0461, 140.0588, 136.8622, 145.7028, 142.4845, 141.7123, 148.7326, 139.7837, 147.2626, 141.3051, 144.2495, 145.9874, 147.2437, 147.6260, 150.7171, 145.2480, 138.1099, 135.9185, 141.6569, 153.7574, 145.3144, 144.2470, 140.4781, 147.9522, 155.4317, 148.3894, 142.7386, 133.0097, 142.0983, 143.5631, 137.1423, 152.1611, 141.2969, 143.9947, 135.2958, 151.2920, 141.5346, 154.3987, 147.2201, 147.0072, 147.5867, 146.1475, 140.1022, 151.9169, 145.8336, 145.1522, 153.8171, 142.4751, 128.8104, 147.0667, 146.4162, 148.2855, 148.6449, 152.6766, 145.0643, 143.7891, 145.8554, 146.0938, 144.3218, 149.3112, 143.3344, 142.4080, 150.2182, 144.2672, 143.0363, 153.7051, 148.7146, 132.4402, 142.8612, 155.4186, 142.1790, 143.7092, 150.1514, 149.4720, 138.3438, 140.7296, 145.9898, 155.5039, 150.3572, 146.6444, 143.2776, 143.9160, 140.7035, 146.1718, 136.7604, 143.8311, 137.8524, 126.0465, 143.4342, 151.7148, 142.5143, 139.1757, 142.9162, 150.4362, 143.3389
## 6 1.0000, 2.0000, 3.0000, 4.0000, 5.0000, 6.0000, 7.0000, 8.0000, 9.0000, 10.0000, 11.0000, 12.0000, 13.0000, 14.0000, 15.0000, 16.0000, 17.0000, 18.0000, 19.0000, 20.0000, 21.0000, 22.0000, 23.0000, 24.0000, 25.0000, 26.0000, 27.0000, 28.0000, 29.0000, 30.0000, 31.0000, 32.0000, 33.0000, 34.0000, 35.0000, 36.0000, 37.0000, 38.0000, 39.0000, 40.0000, 41.0000, 42.0000, 43.0000, 44.0000, 45.0000, 46.0000, 47.0000, 48.0000, 49.0000, 50.0000, 51.0000, 52.0000, 53.0000, 54.0000, 55.0000, 56.0000, 57.0000, 58.0000, 59.0000, 60.0000, 61.0000, 62.0000, 63.0000, 64.0000, 65.0000, 66.0000, 67.0000, 68.0000, 69.0000, 70.0000, 71.0000, 72.0000, 73.0000, 74.0000, 75.0000, 76.0000, 77.0000, 78.0000, 79.0000, 80.0000, 81.0000, 82.0000, 83.0000, 84.0000, 85.0000, 86.0000, 87.0000, 88.0000, 89.0000, 90.0000, 91.0000, 92.0000, 93.0000, 94.0000, 95.0000, 96.0000, 97.0000, 98.0000, 99.0000, 100.0000, 101.0000, 102.0000, 103.0000, 104.0000, 105.0000, 106.0000, 107.0000, 108.0000, 109.0000, 110.0000, 111.0000, 112.0000, 113.0000, 114.0000, 115.0000, 116.0000, 117.0000, 118.0000, 119.0000, 120.0000, 121.0000, 122.0000, 123.0000, 124.0000, 125.0000, 126.0000, 127.0000, 128.0000, 129.0000, 130.0000, 131.0000, 132.0000, 133.0000, 134.0000, 135.0000, 136.0000, 137.0000, 138.0000, 139.0000, 140.0000, 141.0000, 142.0000, 143.0000, 144.0000, 145.0000, 146.0000, 147.0000, 148.0000, 149.0000, 150.0000, 151.0000, 152.0000, 153.0000, 154.0000, 155.0000, 156.0000, 157.0000, 158.0000, 159.0000, 160.0000, 161.0000, 162.0000, 163.0000, 164.0000, 165.0000, 166.0000, 167.0000, 168.0000, 169.0000, 170.0000, 171.0000, 172.0000, 173.0000, 174.0000, 175.0000, 176.0000, 177.0000, 178.0000, 179.0000, 180.0000, 181.0000, 182.0000, 183.0000, 184.0000, 185.0000, 186.0000, 187.0000, 188.0000, 189.0000, 190.0000, 191.0000, 192.0000, 193.0000, 194.0000, 195.0000, 196.0000, 197.0000, 198.0000, 199.0000, 200.0000, 201.0000, 202.0000, 203.0000, 204.0000, 205.0000, 206.0000, 207.0000, 208.0000, 209.0000, 210.0000, 211.0000, 212.0000, 213.0000, 214.0000, 215.0000, 216.0000, 217.0000, 218.0000, 219.0000, 220.0000, 221.0000, 222.0000, 223.0000, 224.0000, 225.0000, 226.0000, 227.0000, 228.0000, 229.0000, 230.0000, 231.0000, 232.0000, 233.0000, 234.0000, 235.0000, 236.0000, 237.0000, 238.0000, 239.0000, 240.0000, 241.0000, 242.0000, 243.0000, 244.0000, 245.0000, 246.0000, 247.0000, 248.0000, 249.0000, 250.0000, 251.0000, 252.0000, 253.0000, 254.0000, 255.0000, 256.0000, 257.0000, 258.0000, 259.0000, 260.0000, 261.0000, 262.0000, 263.0000, 264.0000, 265.0000, 266.0000, 267.0000, 268.0000, 269.0000, 270.0000, 271.0000, 272.0000, 273.0000, 274.0000, 275.0000, 276.0000, 277.0000, 278.0000, 279.0000, 280.0000, 281.0000, 282.0000, 283.0000, 284.0000, 285.0000, 286.0000, 287.0000, 288.0000, 289.0000, 290.0000, 291.0000, 292.0000, 293.0000, 294.0000, 295.0000, 296.0000, 297.0000, 298.0000, 299.0000, 300.0000, 301.0000, 302.0000, 303.0000, 304.0000, 305.0000, 306.0000, 307.0000, 308.0000, 309.0000, 310.0000, 311.0000, 312.0000, 313.0000, 314.0000, 315.0000, 316.0000, 317.0000, 318.0000, 319.0000, 320.0000, 321.0000, 322.0000, 323.0000, 324.0000, 325.0000, 326.0000, 327.0000, 328.0000, 329.0000, 330.0000, 331.0000, 332.0000, 333.0000, 334.0000, 335.0000, 336.0000, 337.0000, 338.0000, 339.0000, 340.0000, 341.0000, 342.0000, 343.0000, 344.0000, 345.0000, 346.0000, 347.0000, 348.0000, 349.0000, 350.0000, 351.0000, 352.0000, 353.0000, 354.0000, 355.0000, 356.0000, 357.0000, 358.0000, 359.0000, 360.0000, 361.0000, 362.0000, 363.0000, 364.0000, 365.0000, 366.0000, 367.0000, 368.0000, 369.0000, 370.0000, 371.0000, 372.0000, 373.0000, 374.0000, 375.0000, 376.0000, 377.0000, 378.0000, 379.0000, 380.0000, 381.0000, 382.0000, 383.0000, 384.0000, 385.0000, 386.0000, 387.0000, 388.0000, 389.0000, 390.0000, 391.0000, 392.0000, 393.0000, 394.0000, 395.0000, 396.0000, 397.0000, 398.0000, 399.0000, 400.0000, 401.0000, 402.0000, 403.0000, 404.0000, 405.0000, 406.0000, 407.0000, 408.0000, 409.0000, 410.0000, 411.0000, 412.0000, 413.0000, 414.0000, 415.0000, 416.0000, 417.0000, 418.0000, 419.0000, 420.0000, 421.0000, 422.0000, 423.0000, 424.0000, 425.0000, 426.0000, 427.0000, 428.0000, 429.0000, 430.0000, 431.0000, 432.0000, 433.0000, 434.0000, 435.0000, 436.0000, 437.0000, 438.0000, 439.0000, 440.0000, 441.0000, 442.0000, 443.0000, 444.0000, 445.0000, 446.0000, 447.0000, 448.0000, 449.0000, 450.0000, 451.0000, 452.0000, 453.0000, 454.0000, 455.0000, 456.0000, 457.0000, 458.0000, 459.0000, 460.0000, 461.0000, 462.0000, 463.0000, 464.0000, 465.0000, 466.0000, 467.0000, 468.0000, 469.0000, 470.0000, 471.0000, 472.0000, 473.0000, 474.0000, 475.0000, 476.0000, 477.0000, 478.0000, 479.0000, 480.0000, 481.0000, 482.0000, 483.0000, 484.0000, 485.0000, 486.0000, 487.0000, 488.0000, 489.0000, 490.0000, 491.0000, 492.0000, 493.0000, 494.0000, 495.0000, 496.0000, 497.0000, 498.0000, 499.0000, 500.0000, 501.0000, 502.0000, 503.0000, 504.0000, 505.0000, 506.0000, 507.0000, 508.0000, 509.0000, 510.0000, 511.0000, 512.0000, 513.0000, 514.0000, 515.0000, 516.0000, 517.0000, 518.0000, 519.0000, 520.0000, 521.0000, 522.0000, 523.0000, 524.0000, 525.0000, 526.0000, 527.0000, 528.0000, 529.0000, 530.0000, 531.0000, 532.0000, 533.0000, 534.0000, 535.0000, 536.0000, 537.0000, 538.0000, 539.0000, 540.0000, 541.0000, 542.0000, 543.0000, 544.0000, 545.0000, 546.0000, 547.0000, 548.0000, 549.0000, 550.0000, 551.0000, 552.0000, 553.0000, 554.0000, 555.0000, 556.0000, 557.0000, 558.0000, 559.0000, 560.0000, 561.0000, 562.0000, 563.0000, 564.0000, 565.0000, 566.0000, 567.0000, 568.0000, 569.0000, 570.0000, 571.0000, 572.0000, 573.0000, 574.0000, 575.0000, 576.0000, 577.0000, 578.0000, 579.0000, 580.0000, 581.0000, 582.0000, 583.0000, 584.0000, 585.0000, 586.0000, 587.0000, 588.0000, 589.0000, 590.0000, 591.0000, 592.0000, 593.0000, 594.0000, 595.0000, 596.0000, 597.0000, 598.0000, 599.0000, 600.0000, 601.0000, 602.0000, 603.0000, 604.0000, 605.0000, 606.0000, 607.0000, 608.0000, 609.0000, 610.0000, 611.0000, 612.0000, 613.0000, 614.0000, 615.0000, 616.0000, 617.0000, 618.0000, 619.0000, 620.0000, 621.0000, 622.0000, 623.0000, 624.0000, 625.0000, 626.0000, 627.0000, 628.0000, 629.0000, 630.0000, 631.0000, 632.0000, 633.0000, 634.0000, 635.0000, 636.0000, 637.0000, 638.0000, 639.0000, 640.0000, 641.0000, 642.0000, 643.0000, 644.0000, 645.0000, 646.0000, 647.0000, 648.0000, 649.0000, 650.0000, 651.0000, 652.0000, 653.0000, 654.0000, 655.0000, 656.0000, 657.0000, 658.0000, 659.0000, 660.0000, 661.0000, 662.0000, 663.0000, 664.0000, 665.0000, 666.0000, 667.0000, 668.0000, 669.0000, 670.0000, 671.0000, 672.0000, 673.0000, 674.0000, 675.0000, 676.0000, 677.0000, 678.0000, 679.0000, 680.0000, 681.0000, 682.0000, 683.0000, 684.0000, 685.0000, 686.0000, 687.0000, 688.0000, 689.0000, 690.0000, 691.0000, 692.0000, 693.0000, 694.0000, 695.0000, 696.0000, 697.0000, 698.0000, 699.0000, 700.0000, 701.0000, 702.0000, 703.0000, 704.0000, 705.0000, 706.0000, 707.0000, 708.0000, 709.0000, 710.0000, 711.0000, 712.0000, 713.0000, 714.0000, 715.0000, 716.0000, 717.0000, 718.0000, 719.0000, 720.0000, 721.0000, 722.0000, 723.0000, 724.0000, 725.0000, 726.0000, 727.0000, 728.0000, 729.0000, 730.0000, 731.0000, 732.0000, 733.0000, 734.0000, 735.0000, 736.0000, 737.0000, 738.0000, 739.0000, 740.0000, 741.0000, 742.0000, 743.0000, 744.0000, 745.0000, 746.0000, 747.0000, 748.0000, 749.0000, 750.0000, 751.0000, 752.0000, 753.0000, 754.0000, 755.0000, 756.0000, 757.0000, 758.0000, 759.0000, 760.0000, 761.0000, 762.0000, 763.0000, 764.0000, 765.0000, 766.0000, 767.0000, 768.0000, 769.0000, 770.0000, 771.0000, 772.0000, 773.0000, 774.0000, 775.0000, 776.0000, 777.0000, 778.0000, 779.0000, 780.0000, 781.0000, 782.0000, 783.0000, 784.0000, 785.0000, 786.0000, 787.0000, 788.0000, 789.0000, 790.0000, 791.0000, 792.0000, 793.0000, 794.0000, 795.0000, 796.0000, 797.0000, 798.0000, 799.0000, 800.0000, 801.0000, 802.0000, 803.0000, 804.0000, 805.0000, 806.0000, 807.0000, 808.0000, 809.0000, 810.0000, 811.0000, 812.0000, 813.0000, 814.0000, 815.0000, 816.0000, 817.0000, 818.0000, 819.0000, 820.0000, 821.0000, 822.0000, 823.0000, 824.0000, 825.0000, 826.0000, 827.0000, 828.0000, 829.0000, 830.0000, 831.0000, 832.0000, 833.0000, 834.0000, 835.0000, 836.0000, 837.0000, 838.0000, 839.0000, 840.0000, 841.0000, 842.0000, 843.0000, 844.0000, 845.0000, 846.0000, 847.0000, 848.0000, 849.0000, 850.0000, 851.0000, 852.0000, 853.0000, 854.0000, 855.0000, 856.0000, 857.0000, 858.0000, 859.0000, 860.0000, 861.0000, 862.0000, 863.0000, 864.0000, 865.0000, 866.0000, 867.0000, 868.0000, 869.0000, 870.0000, 871.0000, 872.0000, 873.0000, 874.0000, 875.0000, 876.0000, 877.0000, 878.0000, 879.0000, 880.0000, 881.0000, 882.0000, 883.0000, 884.0000, 885.0000, 886.0000, 887.0000, 888.0000, 889.0000, 890.0000, 891.0000, 892.0000, 893.0000, 894.0000, 895.0000, 896.0000, 897.0000, 898.0000, 899.0000, 900.0000, 901.0000, 902.0000, 903.0000, 904.0000, 905.0000, 906.0000, 907.0000, 908.0000, 909.0000, 910.0000, 911.0000, 912.0000, 913.0000, 914.0000, 915.0000, 916.0000, 917.0000, 918.0000, 919.0000, 920.0000, 921.0000, 922.0000, 923.0000, 924.0000, 925.0000, 926.0000, 927.0000, 928.0000, 929.0000, 930.0000, 931.0000, 932.0000, 933.0000, 934.0000, 935.0000, 936.0000, 937.0000, 938.0000, 939.0000, 940.0000, 941.0000, 942.0000, 943.0000, 944.0000, 945.0000, 946.0000, 947.0000, 948.0000, 949.0000, 950.0000, 951.0000, 952.0000, 953.0000, 954.0000, 955.0000, 956.0000, 957.0000, 958.0000, 959.0000, 960.0000, 961.0000, 962.0000, 963.0000, 964.0000, 965.0000, 966.0000, 967.0000, 968.0000, 969.0000, 970.0000, 971.0000, 972.0000, 973.0000, 974.0000, 975.0000, 976.0000, 977.0000, 978.0000, 979.0000, 980.0000, 981.0000, 982.0000, 983.0000, 984.0000, 985.0000, 986.0000, 987.0000, 988.0000, 989.0000, 990.0000, 991.0000, 992.0000, 993.0000, 994.0000, 995.0000, 996.0000, 997.0000, 998.0000, 999.0000, 1000.0000, 138.1222, 153.8148, 137.7521, 141.8723, 150.6852, 152.9844, 143.9341, 142.8716, 141.5654, 146.2355, 141.4546, 147.3095, 146.9478, 148.5729, 144.1769, 154.1869, 147.5398, 147.1098, 135.8321, 143.3280, 150.4660, 139.4901, 137.6007, 142.7508, 143.6994, 146.3006, 149.4727, 140.8982, 147.6190, 143.2261, 143.4702, 141.2670, 145.1652, 149.2384, 141.1520, 138.9447, 152.6895, 136.2187, 141.2340, 143.9072, 150.6070, 148.8267, 144.4381, 137.6099, 157.4905, 140.6375, 151.6884, 155.4864, 142.4504, 140.4845, 151.3203, 152.8441, 148.3650, 138.1345, 141.5921, 145.1053, 146.1723, 143.5114, 146.2043, 149.8171, 143.8726, 147.9481, 143.4953, 143.2029, 159.1934, 139.6986, 146.5333, 152.8257, 156.7715, 149.1607, 152.3691, 155.9500, 147.3358, 142.0414, 149.1093, 146.3184, 146.6995, 145.9213, 160.2002, 148.1885, 146.5548, 141.3221, 153.2488, 141.3260, 142.9716, 149.1748, 150.6969, 138.9949, 143.3801, 136.2058, 144.0537, 151.9635, 143.2622, 151.9370, 145.3565, 150.6424, 144.0237, 146.5093, 147.0453, 152.4857, 147.1258, 145.8879, 149.8628, 145.1225, 147.9599, 139.5539, 148.7415, 148.9549, 151.3708, 149.3405, 142.8758, 142.9451, 141.0398, 141.8063, 149.1799, 146.8693, 146.0008, 147.8427, 144.4093, 149.8622, 144.3508, 139.5566, 143.1635, 146.3985, 148.1668, 140.7676, 145.6560, 151.8353, 150.6939, 142.5587, 147.5550, 149.8283, 144.1729, 137.7663, 141.7720, 134.4627, 146.7221, 147.6454, 150.8538, 136.8757, 144.1231, 142.5539, 148.8033, 134.7151, 145.0741, 150.1339, 139.8266, 148.5291, 144.2193, 146.3169, 142.0991, 137.2764, 148.7303, 145.1693, 144.6317, 143.8303, 145.8885, 148.1392, 146.6452, 142.2679, 144.9771, 140.2532, 143.0426, 137.0001, 144.6197, 147.8429, 146.9503, 141.2209, 152.9958, 137.1227, 143.2318, 144.1201, 141.7894, 145.4625, 144.7185, 154.8028, 140.5071, 143.7194, 143.7680, 144.4409, 149.1242, 143.4547, 147.5397, 137.0457, 147.1463, 140.1179, 142.0964, 149.0485, 141.3190, 140.5814, 150.5485, 141.3276, 151.8223, 148.6234, 142.9322, 137.5591, 135.8218, 144.0121, 141.2704, 133.1281, 142.6356, 140.5936, 146.2949, 143.5808, 145.9092, 141.0017, 139.2082, 145.3838, 155.2497, 149.8538, 139.9110, 152.0260, 140.9949, 143.6604, 143.6703, 147.3395, 140.6430, 147.5796, 143.5529, 148.4641, 143.7039, 143.8549, 146.8168, 147.1638, 145.2116, 146.2122, 152.7134, 147.5747, 147.6699, 141.7993, 147.8762, 148.6935, 151.8090, 151.8582, 145.0391, 152.4080, 147.1890, 145.2198, 146.8527, 156.4676, 151.1913, 146.6571, 133.8297, 148.6718, 142.8770, 151.5385, 155.1169, 137.6172, 146.7870, 146.1619, 154.7764, 139.2366, 145.2579, 151.0172, 139.2074, 145.8146, 147.3504, 144.9491, 142.3102, 142.9707, 146.2346, 150.5186, 154.1047, 127.2868, 147.3181, 131.8516, 139.2612, 147.1048, 144.0850, 148.3383, 149.7078, 142.3352, 146.9142, 143.0315, 146.1892, 145.5635, 151.7619, 149.5143, 146.6886, 138.9314, 151.9574, 149.2216, 148.6581, 144.0123, 140.4509, 152.1174, 148.0636, 146.5444, 151.0220, 146.2390, 139.0403, 150.3084, 143.0099, 148.9677, 154.0509, 151.4154, 144.2329, 152.4178, 149.7281, 143.4123, 152.1717, 143.5240, 132.3010, 147.5632, 139.9611, 149.4986, 142.0683, 140.9640, 157.3229, 146.3850, 153.1824, 139.5119, 150.2356, 147.1607, 145.9612, 142.4963, 140.5094, 146.6942, 142.2366, 149.4047, 148.7191, 146.5294, 140.2030, 142.5537, 145.9614, 142.4614, 146.1421, 150.1691, 142.5105, 145.0047, 148.5845, 141.7808, 150.2617, 142.3951, 143.2032, 146.3626, 147.8459, 143.5888, 144.2559, 148.1874, 140.3329, 143.8196, 146.0517, 148.2140, 143.8999, 139.5461, 137.3215, 138.2108, 144.3232, 149.9076, 138.1824, 140.8005, 156.9561, 140.3170, 141.5062, 144.5828, 146.8739, 139.6863, 147.0858, 157.9426, 150.0579, 148.1937, 146.7134, 144.3636, 135.5862, 149.5233, 142.3604, 141.6675, 144.6715, 141.6559, 144.1962, 145.6532, 154.7442, 148.8539, 144.2540, 141.9769, 144.3321, 150.5489, 149.1602, 147.1196, 147.6151, 150.8783, 147.3859, 146.6693, 145.6110, 137.9880, 138.8204, 141.6050, 147.4706, 134.3245, 152.8119, 143.6088, 155.0014, 149.3678, 144.5117, 154.6988, 154.7841, 146.2057, 146.9413, 143.2137, 156.9180, 149.9385, 151.9083, 145.8860, 146.4688, 148.3134, 147.6048, 149.4217, 145.4214, 154.5103, 146.8759, 137.7974, 143.5189, 141.6742, 139.4152, 153.7638, 152.4492, 144.4708, 141.9637, 140.1371, 141.6323, 146.0993, 143.4075, 141.9255, 149.3382, 158.8001, 148.3604, 147.0785, 141.4802, 146.0317, 154.1263, 148.3054, 141.0191, 154.6868, 145.0805, 146.1788, 150.6459, 141.5645, 149.6842, 143.6881, 147.7190, 145.2333, 140.9966, 142.1832, 146.6237, 146.3338, 155.3134, 134.2471, 145.7552, 142.4514, 146.8528, 141.5577, 146.4119, 140.9686, 144.1959, 140.6765, 143.0905, 145.8005, 136.2491, 144.8534, 141.6630, 139.7470, 155.0872, 147.2679, 147.8913, 145.8034, 145.5171, 149.8949, 140.8720, 143.3990, 134.0419, 139.8161, 146.8272, 138.7760, 160.3123, 145.3596, 133.2346, 155.1892, 144.1801, 140.9122, 144.0349, 143.7494, 142.2945, 141.1076, 149.5013, 142.2654, 132.5825, 154.9055, 141.5944, 143.7894, 147.6657, 143.9848, 143.9719, 146.8754, 147.9955, 145.7392, 145.0320, 146.1854, 140.9999, 142.5722, 153.5602, 141.9539, 136.1500, 147.6219, 152.2607, 138.8088, 151.5411, 140.2529, 142.0535, 143.6802, 147.8265, 137.1505, 142.8188, 151.1297, 153.2265, 142.2025, 146.0712, 146.4631, 152.3519, 145.4166, 140.0296, 144.7993, 146.2356, 146.1625, 149.1659, 153.9082, 152.6123, 142.1476, 145.1590, 144.8425, 145.2464, 141.1116, 153.4976, 139.7764, 139.2346, 146.9896, 141.9590, 139.6905, 147.0407, 139.9073, 137.2738, 139.9209, 149.0689, 145.6026, 148.0428, 139.2436, 132.7312, 143.3323, 151.9575, 146.7131, 141.6004, 141.1550, 145.8288, 151.4936, 143.9029, 144.3286, 150.8122, 149.4514, 146.4344, 153.4291, 145.4931, 145.4466, 144.3738, 142.7681, 140.1439, 140.5057, 141.6219, 158.1146, 149.9076, 150.2318, 144.3981, 140.6013, 148.7388, 135.7357, 139.7153, 151.5123, 150.0146, 142.0450, 148.6705, 142.5089, 154.5468, 138.4155, 144.9230, 145.6855, 145.0959, 147.3346, 135.3178, 137.4402, 149.1021, 147.4493, 140.2160, 154.7542, 141.9157, 153.2852, 136.4347, 130.2152, 148.8374, 151.7479, 147.5884, 143.1028, 144.2782, 154.1358, 143.2865, 144.6926, 145.2211, 146.3398, 153.2241, 152.1331, 140.5027, 146.2519, 158.2209, 151.4296, 151.4490, 138.5522, 145.5087, 147.9335, 142.9364, 142.0526, 138.7001, 144.2593, 140.2048, 147.4236, 143.8228, 142.5887, 139.4140, 153.6117, 139.4769, 143.5515, 140.9130, 144.2786, 156.0728, 144.0211, 145.0898, 140.8840, 149.1947, 140.9709, 148.6098, 146.5683, 138.3983, 140.0312, 152.4370, 143.5585, 137.6832, 144.8056, 146.0188, 148.7614, 146.5906, 141.2113, 140.9216, 139.3053, 143.2013, 138.4818, 139.1475, 156.0985, 143.4030, 148.3929, 146.9293, 149.0457, 144.1069, 149.0662, 146.7311, 155.5274, 143.9750, 146.3296, 153.8731, 138.2383, 145.9385, 141.6218, 140.2705, 137.5085, 138.7912, 138.7214, 152.9469, 145.1356, 149.9930, 145.1180, 151.0236, 150.9094, 148.0797, 140.1558, 148.2896, 136.1902, 138.8886, 147.7694, 146.4382, 147.3303, 144.4425, 143.5971, 146.8207, 140.8569, 149.7611, 141.9525, 143.0558, 141.6531, 143.6694, 145.0044, 139.1931, 142.4264, 135.9011, 148.7584, 141.8537, 149.0061, 130.7719, 147.8415, 153.8854, 145.3748, 146.4599, 145.7362, 147.2397, 137.4133, 147.1797, 139.3212, 148.6754, 149.8592, 149.2200, 141.8820, 148.8747, 138.4357, 142.9449, 141.9964, 147.0569, 143.1344, 144.6737, 149.5005, 142.3908, 147.4045, 147.6231, 148.4890, 145.2328, 142.5143, 152.4852, 147.1313, 137.7240, 144.9173, 137.0426, 139.5127, 153.9295, 139.1767, 146.8740, 142.3339, 148.8998, 143.3842, 153.4307, 152.2038, 142.9198, 146.4988, 145.2465, 145.2113, 144.1499, 151.0123, 139.5420, 143.7946, 139.0529, 151.1922, 140.2477, 141.8439, 142.6068, 145.3776, 140.6269, 137.8642, 148.1615, 153.3271, 141.5825, 147.6531, 144.7144, 139.0153, 142.5440, 145.4327, 143.0489, 149.2052, 151.6464, 143.2656, 143.6192, 143.3043, 149.1364, 135.5900, 145.5325, 149.6919, 142.7523, 145.0812, 144.4601, 143.0780, 155.3549, 138.2346, 142.7220, 152.3054, 148.7519, 151.5617, 151.7743, 148.4129, 142.5765, 142.5899, 144.5638, 153.9358, 139.1579, 138.2550, 143.8407, 139.5322, 142.7494, 157.0553, 141.5594, 140.6576, 135.1488, 141.6886, 138.5856, 151.8226, 148.3941, 142.2830, 139.9202, 140.4276, 131.5973, 148.2331, 139.2621, 141.7019, 151.4724, 147.0282, 138.7982, 141.2669, 147.7220, 148.4786, 146.6227, 141.8170, 142.7570, 150.7175, 145.2013, 141.5374, 141.0010, 152.3430, 144.7848, 141.6004, 149.7511, 154.7496, 145.4636, 150.4252, 143.0816, 147.3563, 145.0296, 137.7276, 152.2254, 137.1996, 153.3015, 146.9067, 138.7101, 145.8230, 143.7605, 142.3583, 138.6569, 140.4381, 156.6222, 145.7915, 152.9961, 139.4508, 141.8648, 142.3212, 140.9460, 149.4291, 148.9349, 137.4945, 138.6384, 137.5208, 148.2937, 151.8938, 150.1443, 133.1620, 146.4903, 143.0612, 152.4832, 156.2790, 142.9445, 148.0093, 147.6667, 145.8304, 148.2204, 140.7266, 153.3419, 150.8767, 146.8195, 143.0795, 149.9793, 148.8358, 140.6145, 143.6915, 148.1379, 147.5178, 150.6210, 143.6823, 147.2399, 148.9613, 141.1809, 148.8473, 144.6788, 131.7982, 141.3081, 149.6534, 139.7106, 149.2390, 145.8838, 145.8496, 150.1856, 142.1623, 146.0658, 147.2568, 143.7798, 148.9203, 143.9928, 147.9042, 136.3841, 139.1156, 140.1522, 148.0526, 148.6863, 145.6104, 148.4075, 146.0076, 148.1655, 154.1550, 147.9750, 142.3603, 146.4315, 148.5751, 143.9472, 149.7508, 144.2183, 141.8989, 152.0695, 147.4455, 144.2136, 142.1667, 142.2458, 141.8029, 149.3032, 137.4011, 129.6686, 149.0500, 146.4549, 142.9334, 147.6236, 152.3617, 141.8811, 142.8320, 155.5227, 139.9891, 150.9237, 138.1863, 146.9016, 146.9052, 146.7411, 149.2336, 137.6210, 137.2784, 137.1808, 149.9635, 146.7915, 142.6326, 150.0983, 145.8509, 146.7973, 138.5621, 145.3525, 143.1193, 136.3077, 146.3355, 145.5296, 156.5166, 148.4016, 139.3758, 146.6624, 146.5491, 152.3991, 156.6149, 149.7739, 152.2456, 148.5446, 151.2793, 150.8866, 141.9120, 151.7050, 142.6356, 153.2049, 147.1380, 144.8459, 149.6471, 140.5908, 145.5933, 143.7974, 137.9190, 149.3516, 148.5779, 137.9088, 135.8471, 146.0372, 156.4116, 146.9514, 144.0711, 144.7633, 141.7980, 146.9825, 145.9448, 151.9771, 145.9369
## weight
## 1 31.52464
## 2 32.16685
## 3 32.80905
## 4 33.45125
## 5 34.09346
## 6 34.73566
prediction_range <- simulated_data %>%
mutate(height_pi = map(data, ~ rethinking::PI(.x$datapoint)),
height_pi = map(height_pi,
~ data_frame(lower_pi = .x[[1]],
upper_pi = .x[[2]]))) %>%
unnest(height_pi)
mean_and_raw +
geom_ribbon(aes(ymin = lower_pi, ymax= upper_pi), data = prediction_range, alpha = 0.2) +
theme_minimal()
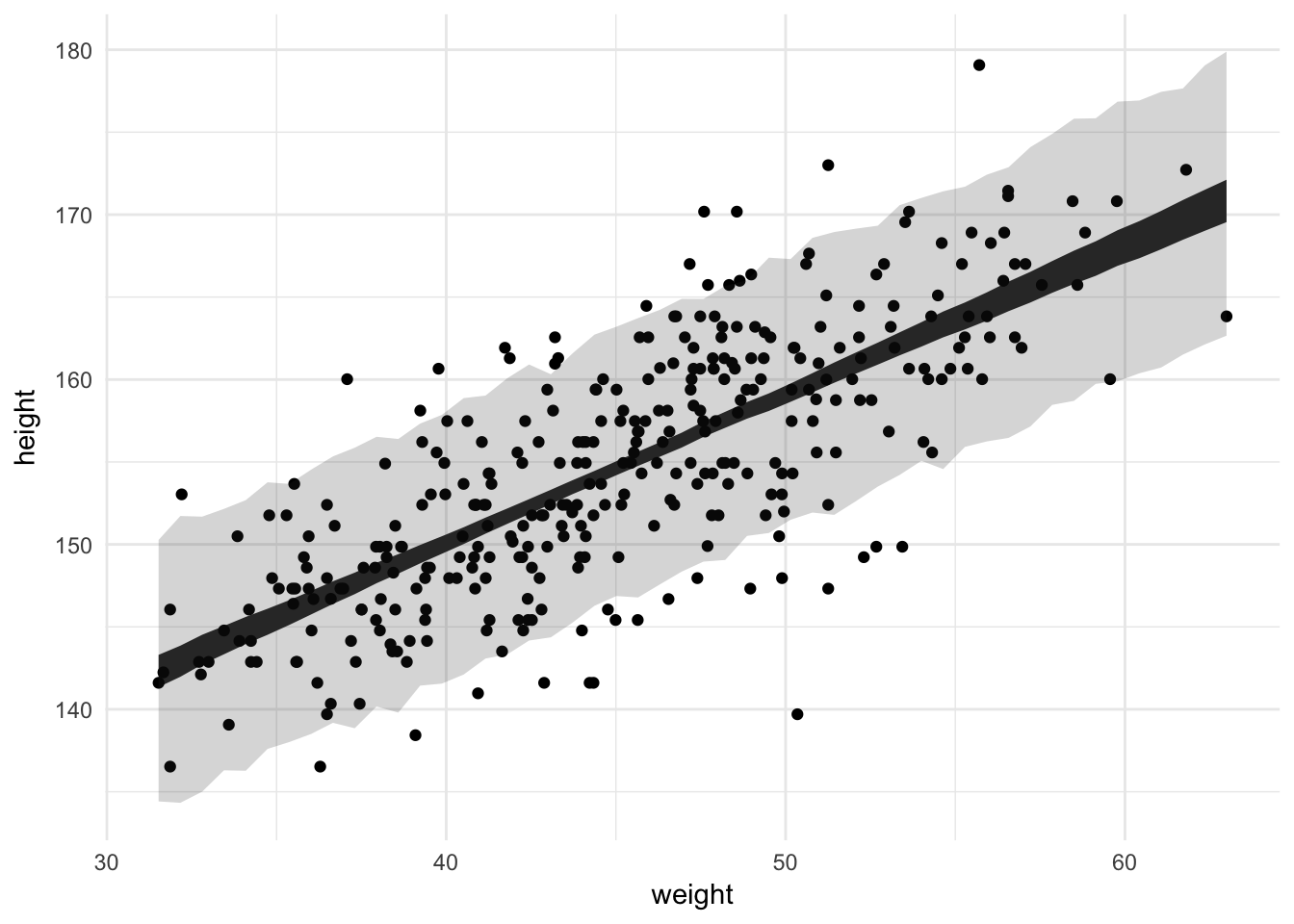
Figure 4.2: predicting the actual data. this includes the predicted standard deviation of the response.
4.2.1 Polynomial
d <- Howell1
str(d)
## 'data.frame': 544 obs. of 4 variables:
## $ height: num 152 140 137 157 145 ...
## $ weight: num 47.8 36.5 31.9 53 41.3 ...
## $ age : num 63 63 65 41 51 35 32 27 19 54 ...
## $ male : int 1 0 0 1 0 1 0 1 0 1 ...
d_stand_weight <- d %>%
mutate(weight.s = (weight - mean(weight)) / sd(weight))
d_stand_weight %>%
select(height, starts_with("weight")) %>%
gather(wtvar, weight, starts_with("weight")) %>%
ggplot(aes(x = weight, y = height)) + geom_point() + facet_grid(~wtvar, scales = "free_x")
quad_mod <- alist(
height ~ dnorm(mu, sigma),
mu <- a + b1 * weight.s + b2 * weight.s2,
a ~ dnorm(178, 100),
b1 ~ dnorm(0, 10),
b2 ~ dnorm(0, 10),
sigma ~ dunif(0, 50)
)
m4.5 <- d_stand_weight %>%
mutate(weight.s2 = weight.s^2) %>%
rethinking::map(flist = quad_mod,
data = .)
rethinking::precis(m4.5)
## Mean StdDev 5.5% 94.5%
## a 146.66 0.37 146.07 147.26
## b1 21.40 0.29 20.94 21.86
## b2 -8.42 0.28 -8.86 -7.97
## sigma 5.75 0.17 5.47 6.03
4.3 Exercises
4.3.1 easy
4.3.2 medium
4.3.3 hard
4.4 When adding variables hurts
# number of plants
N <- 100
set.seed(4812)
fungal_data <- data_frame(
# initial heights
h0 = rnorm(N, 10, 2),
treatment = rep(0:1, each = N/2),
fungus = rbinom(N, size = 1, prob = 0.5 - treatment*0.4),
h1 = h0 + rnorm(N, 5 - 3 * fungus),
# departure from the book, but why not center h0??
h0_c = h0 - mean(h0)
)
fungal_data %>%
ggplot(aes(x = h0_c, y = h1, fill = factor(fungus))) + geom_point(pch = 21) + facet_wrap(~treatment) + stat_smooth(method = "lm")
4.4.1 the trouble with fitting post-treatment effects
Sometimes biologists are tempted to include both a treatment and one of the responses in a linear model.
m5.13_define <- alist(
h1 ~ dnorm(mu, sigma),
mu <- a + b_trt * treatment + b_fungus * fungus + b_h * h0_c,
a ~ dnorm(0, 100),
c(b_trt, b_fungus, b_h) ~ dnorm(0, 10),
sigma ~ dunif(0, 10)
)
m5.13 <- rethinking::map(m5.13_define, data = as.data.frame(fungal_data))
rethinking::precis(m5.13)
## Mean StdDev 5.5% 94.5%
## a 15.05 0.18 14.76 15.35
## b_trt 0.07 0.21 -0.27 0.41
## b_fungus -3.19 0.23 -3.55 -2.83
## b_h 1.00 0.04 0.93 1.07
## sigma 0.94 0.07 0.84 1.05
alright so that can’t be the right answer!! One solution, proposed at this point in the book, is to instead remove the fungal treatment from the model.
m5.14_define <- alist(
h1 ~ dnorm(mu, sigma),
mu <- a + b_trt * treatment + b_h * h0_c,
a ~ dnorm(0, 100),
c(b_trt, b_h) ~ dnorm(0, 10),
sigma ~ dunif(0, 10)
)
m5.14 <- rethinking::map(m5.14_define, data = as.data.frame(fungal_data))
rethinking::precis(m5.14)
## Mean StdDev 5.5% 94.5%
## a 13.27 0.23 12.90 13.64
## b_trt 1.47 0.33 0.95 1.99
## b_h 1.04 0.08 0.92 1.16
## sigma 1.64 0.12 1.45 1.82
Now we see that plants definitely grow better with the treatment!
4.4.2 Could multilevel models help here?
This is not the point at this juncture in the book, but I am curious!
m5.13_hier_def <- alist(
h1 ~ dnorm(mu, sigma),
mu <- b0 + b_h * h0_c + b_fungus * fungus,
fungus ~ dbinom(1, ppp),
logit(ppp) <- b0f + b_trt * treatment,
c(b0, b_h, b_fungus) ~ dnorm(0, 5),
c(b0f, b_trt) ~ dnorm(0, 5),
sigma <- dcauchy(0,1)
)
m5.13_hier <- rethinking::map2stan(m5.13_hier_def, data = as.data.frame(fungal_data))
saveRDS(m5.13_hier, "stan_models/m5.13_hier.rds")
m5.13_hier <- readRDS("stan_models/m5.13_hier.rds")
# what are the coefficients:
rethinking::precis(m5.13_hier)
## Mean StdDev lower 0.89 upper 0.89 n_eff Rhat
## b0 15.09 0.12 14.91 15.28 1000 1
## b_h 1.00 0.04 0.93 1.07 1000 1
## b_fungus -3.22 0.20 -3.53 -2.92 858 1
## b0f 0.24 0.28 -0.20 0.71 1000 1
## b_trt -2.27 0.50 -3.07 -1.55 1000 1
## sigma 0.96 0.07 0.85 1.06 802 1
# fit to fake data for predictions
df <- data_frame(treatment = rep(c(0, 1), each = 10),
h0_c = rep(seq(from = -5, to = 5, length.out = 10), 2))
hier_predictions <- rethinking::link(m5.13_hier, data = as.data.frame(df), flatten = FALSE)
## [ 100 / 1000 ]
[ 200 / 1000 ]
[ 300 / 1000 ]
[ 400 / 1000 ]
[ 500 / 1000 ]
[ 600 / 1000 ]
[ 700 / 1000 ]
[ 800 / 1000 ]
[ 900 / 1000 ]
[ 1000 / 1000 ]
hier_predictions$ppp %>% t %>% cbind(df, .) %>%
select(-h0_c) %>%
group_by(treatment) %>%
tidyr::nest() %>%
mutate(interval = purrr::map(data, ~ .x %>% as.matrix %>% rethinking::HPDI(.)),
low = purrr::map_dbl(interval, 1),
high = purrr::map_dbl(interval, 2))
## # A tibble: 2 x 5
## treatment data interval low high
## <dbl> <list> <list> <dbl> <dbl>
## 1 0 <tibble [10 x 1,000]> <dbl [2]> 0.58293263 0.6163986
## 2 1 <tibble [10 x 1,000]> <dbl [2]> 0.05744481 0.2019443
hier_predictions$mu %>% apply(., 2, rethinking::HPDI) %>% t %>% cbind(df, .)
## treatment h0_c |0.89 0.89|
## 1 0 -5.0000000 6.432998 7.319180
## 2 0 -3.8888889 7.593195 8.350001
## 3 0 -2.7777778 8.748718 9.396839
## 4 0 -1.6666667 9.956380 10.522566
## 5 0 -0.5555556 11.083284 11.577541
## 6 0 0.5555556 12.178865 12.662983
## 7 0 1.6666667 13.264975 13.807678
## 8 0 2.7777778 14.315762 14.936999
## 9 0 3.8888889 15.421004 16.153823
## 10 0 5.0000000 16.452730 17.298147
## 11 1 -5.0000000 6.432998 7.319180
## 12 1 -3.8888889 7.593195 8.350001
## 13 1 -2.7777778 8.748718 9.396839
## 14 1 -1.6666667 9.956380 10.522566
## 15 1 -0.5555556 11.083284 11.577541
## 16 1 0.5555556 12.178865 12.662983
## 17 1 1.6666667 13.264975 13.807678
## 18 1 2.7777778 14.315762 14.936999
## 19 1 3.8888889 15.421004 16.153823
## 20 1 5.0000000 16.452730 17.298147
# link gives me the output of each linear model in the equation. The linear models give me the mean growth and the mean probability of fungal infection. Each linear model only uses one independent variable -- the model for the probability of fungus infection uses treatment, and the model for the expected growth uses the starting size (h0_c)
Suppose I want to re-create my figure, to see how the model thinks this system ought to behave (important step for model checking):
# start with samples from the posterior
m5.13_hier_samples <- rethinking::extract.samples(m5.13_hier)
m5.13_hier_samples %>% purrr::map(head)
## $b0
## [1] 15.07409 14.73151 15.10655 15.07113 15.18437 15.06501
##
## $b_h
## [1] 0.9736490 0.9876098 0.9821342 1.0393464 1.0600321 1.0016488
##
## $b_fungus
## [1] -3.179489 -3.097534 -3.405055 -3.226120 -3.176906 -3.172974
##
## $b0f
## [1] 0.33482385 0.47428983 0.28648295 -0.26575485 0.36274726 -0.06148448
##
## $b_trt
## [1] -3.132594 -1.848476 -2.721778 -1.598002 -1.994654 -1.747220
##
## $sigma
## [1] 0.9236528 0.8890455 1.0085262 0.8885583 0.8820609 0.9462573
as.data.frame(m5.13_hier_samples) %>% head
## b0 b_h b_fungus b0f b_trt sigma
## 1 15.07409 0.9736490 -3.179489 0.33482385 -3.132594 0.9236528
## 2 14.73151 0.9876098 -3.097534 0.47428983 -1.848476 0.8890455
## 3 15.10655 0.9821342 -3.405055 0.28648295 -2.721778 1.0085262
## 4 15.07113 1.0393464 -3.226120 -0.26575485 -1.598002 0.8885583
## 5 15.18437 1.0600321 -3.176906 0.36274726 -1.994654 0.8820609
## 6 15.06501 1.0016488 -3.172974 -0.06148448 -1.747220 0.9462573
samples_df <- as.data.frame(m5.13_hier_samples)
# function which creates a sampled data_frame
simulate_fungus_data <- function(b0, b_h, b_fungus, b0f, b_trt, sigma){
df %>%
mutate(prob_fungus = rethinking::inv_logit( b0f + b_trt * treatment),
has_fungus = rbinom(length(prob_fungus), size = 1, prob = prob_fungus),
mean_growth = b0 + b_h * h0_c + b_fungus * has_fungus,
sim_growth = rnorm(length(mean_growth), mean_growth, sigma))
}
fake_data <- samples_df %>%
purrr::pmap(simulate_fungus_data) %>%
bind_rows(.id = "sim")
gg_fake <- fake_data %>%
ggplot(aes(x = h0_c, y = sim_growth)) +
geom_hex(bins = 9) + facet_grid(has_fungus~treatment)
gg_fake +
geom_point(aes(x = h0_c, y = h1, color = factor(has_fungus)), pch = 21, data = fungal_data %>% rename(has_fungus = fungus))
How about some nice summary stats of this?
hier_predictions <- rethinking::link(m5.13_hier,
data = data.frame(treatment = c(0, 1),
h0_c = c(0)),
replace = list(b_fungus = matrix(1.5, nrow = 1000, ncol = 2)))
## [ 100 / 1000 ]
[ 200 / 1000 ]
[ 300 / 1000 ]
[ 400 / 1000 ]
[ 500 / 1000 ]
[ 600 / 1000 ]
[ 700 / 1000 ]
[ 800 / 1000 ]
[ 900 / 1000 ]
[ 1000 / 1000 ]
hier_predictions$mu %>% head
## [,1] [,2]
## [1,] 16.57409 16.57409
## [2,] 16.23151 16.23151
## [3,] 16.60655 16.60655
## [4,] 16.57113 16.57113
## [5,] 16.68437 16.68437
## [6,] 16.56501 16.56501
rethinking::stancode(m5.13_hier)
## data{
## int<lower=1> N;
## int<lower=1> N_fungus;
## real h1[N];
## int fungus[N_fungus];
## real h0_c[N];
## int treatment[N_fungus];
## }
## parameters{
## real b0;
## real b_h;
## real b_fungus;
## real b0f;
## real b_trt;
## real<lower=0> sigma;
## }
## model{
## vector[N_fungus] ppp;
## vector[N] mu;
## sigma ~ cauchy( 0 , 1 );
## b_trt ~ normal( 0 , 5 );
## b0f ~ normal( 0 , 5 );
## b_fungus ~ normal( 0 , 5 );
## b_h ~ normal( 0 , 5 );
## b0 ~ normal( 0 , 5 );
## for ( i in 1:N_fungus ) {
## ppp[i] = b0f + b_trt * treatment[i];
## }
## fungus ~ binomial_logit( 1 , ppp );
## for ( i in 1:N ) {
## mu[i] = b0 + b_h * h0_c[i] + b_fungus * fungus[i];
## }
## h1 ~ normal( mu , sigma );
## }
## generated quantities{
## vector[N_fungus] ppp;
## vector[N] mu;
## real dev;
## dev = 0;
## for ( i in 1:N_fungus ) {
## ppp[i] = b0f + b_trt * treatment[i];
## }
## dev = dev + (-2)*binomial_logit_lpmf( fungus | 1 , ppp );
## for ( i in 1:N ) {
## mu[i] = b0 + b_h * h0_c[i] + b_fungus * fungus[i];
## }
## dev = dev + (-2)*normal_lpdf( h1 | mu , sigma );
## }
fungal_reduction <- fake_data %>%
select(sim, treatment, prob_fungus) %>%
distinct %>%
tidyr::spread(treatment, prob_fungus) %>%
mutate(reduction = `0` - `1`) %>%
.[["reduction"]]
rethinking::HPDI(fungal_reduction)
## |0.89 0.89|
## 0.3261092 0.5773543
median(fungal_reduction)
## [1] 0.4367353
You’ll have about 43% less fungus.
how_much_fungus_hurts <- fake_data %>%
select(sim, has_fungus, sim_growth) %>%
group_by(sim, has_fungus) %>%
summarize(meangrowth = mean(sim_growth)) %>%
tidyr::spread(has_fungus, meangrowth) %>%
mutate(reduction = (`0` - `1`)/`0`) %>%
.[["reduction"]] %>%
purrr::discard(is.na)
rethinking::HPDI(how_much_fungus_hurts)
## |0.89 0.89|
## 0.04991973 0.35492453
median(how_much_fungus_hurts)
## [1] 0.2117909
This fungus makes your crops about 20% smaller
The benefit of applying fertilizer is going to depend on how dangerous this fungus is.
samples_df %>% head
## b0 b_h b_fungus b0f b_trt sigma
## 1 15.07409 0.9736490 -3.179489 0.33482385 -3.132594 0.9236528
## 2 14.73151 0.9876098 -3.097534 0.47428983 -1.848476 0.8890455
## 3 15.10655 0.9821342 -3.405055 0.28648295 -2.721778 1.0085262
## 4 15.07113 1.0393464 -3.226120 -0.26575485 -1.598002 0.8885583
## 5 15.18437 1.0600321 -3.176906 0.36274726 -1.994654 0.8820609
## 6 15.06501 1.0016488 -3.172974 -0.06148448 -1.747220 0.9462573
vary_fungal_effect <- function(fungal_effect) {
samples_df %>%
mutate(b_fungus = fungal_effect) %>%
purrr::pmap(simulate_fungus_data) %>%
bind_rows(.id = "sim") %>%
group_by(sim, treatment) %>%
summarize(mean_growth_final = mean(sim_growth)) %>%
tidyr::spread(treatment, mean_growth_final) %>%
mutate(reduction = (`0` - `1`)/`0`) %>%
.[["reduction"]]
}
fungi_effects <- seq(from = 0, to = -5, by = -1) %>%
purrr::map(vary_fungal_effect)
hdpi <- function(x){
rethinking::HPDI(samples = x)
}
fungi_effects %>%
purrr::map(~ purrr::invoke_map(list(median = median, hdpi), x = .x)) %>%
purrr::map(purrr::flatten) %>% purrr::transpose() %>%
purrr::map(purrr::flatten_dbl) %>%
tibble::as_data_frame(.) %>%
cbind(fungal_effect = seq(from = 0, to = -5, by = -1), .) %>%
ggplot(aes(x = fungal_effect, ymin = `|0.89`, ymax = `0.89|`, y = median)) + geom_pointrange()
4.5 Binomial
4.6 Poisson
library(tidyverse)
## Loading tidyverse: ggplot2
## Loading tidyverse: tibble
## Loading tidyverse: tidyr
## Loading tidyverse: readr
## Loading tidyverse: purrr
## Loading tidyverse: dplyr
## Conflicts with tidy packages ----------------------------------------------
## filter(): dplyr, stats
## lag(): dplyr, stats
data("Kline", package = "rethinking")
head(Kline)
## culture population contact total_tools mean_TU
## 1 Malekula 1100 low 13 3.2
## 2 Tikopia 1500 low 22 4.7
## 3 Santa Cruz 3600 low 24 4.0
## 4 Yap 4791 high 43 5.0
## 5 Lau Fiji 7400 high 33 5.0
## 6 Trobriand 8000 high 19 4.0
d <- Kline %>%
mutate(log_pop = log(population),
contact_high = if_else(contact == "high", true = 1, false = 0))
poisson_tools <- alist(
total_tools ~ dpois(lambda),
log(lambda) <-
)